Back to APIs list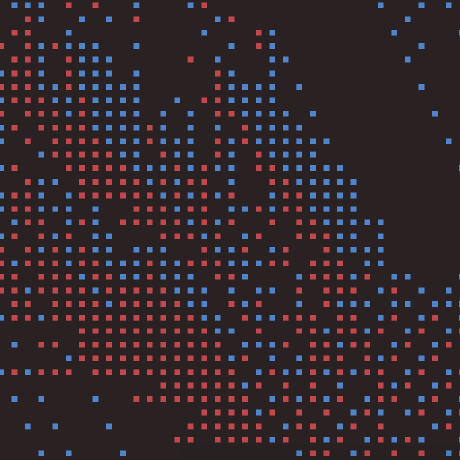
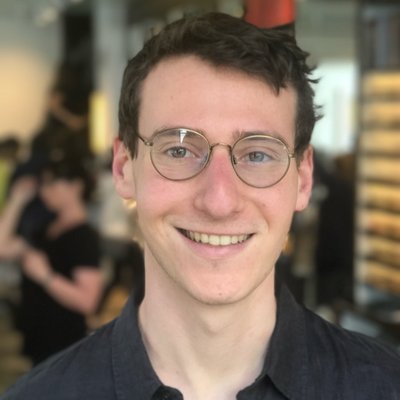
Rime API examples & templates
Use these vals as a playground to view and fork Rime API examples and templates on Val Town. Run any example below or find templates that can be used as a pre-built solution.
IllustratedPrimer
@kousun12
HTTP
Generates a streaming illustrated primer on a subject. ℹ️ Open the url and add a query parameter with a subject (click on titles to "delve"): https://substrate-illustratedprimer.web.val.run/?prompt=modernism 🪩 If you fork, you can sign up for Substrate to get your own API key and $50 in credits
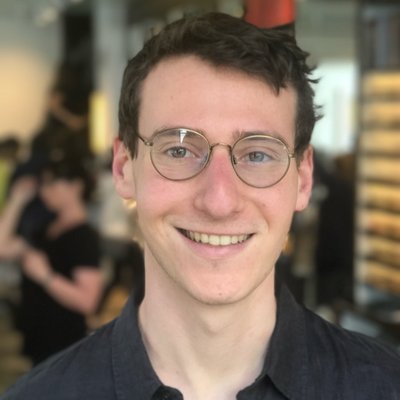
cron
@stevekrouse
HTTP (preview)
CronGPT This is a minisite to help you create cron expressions, particularly for crons on Val Town. It was inspired by Cron Prompt , but also does the timezone conversion from wherever you are to UTC (typically the server timezone). Tech Hono for routing ( GET / and POST /compile .) Hono JSX HTMX (probably overcomplicates things; should remove) @stevekrouse/openai, which is a light wrapper around @std/openai I'm finding HTMX a bit overpowered for this, so I have two experimental forks without it: Vanilla client-side JavaScript: @stevekrouse/cron_client_side_script_fork Client-side ReactJS (no SSR): @stevekrouse/cron_client_react_fork I think (2) Client-side React without any SSR is the simplest architecture. Maybe will move to that.