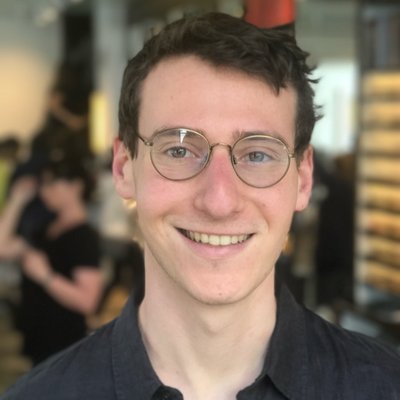
@stevekrouse
Inspector to browser json data in HTTP vals
Example: https://val.town/v/stevekrouse/weatherDescription
Thanks @mmcgrana (https://markmcgranaghan.com/) for the idea!
Blob Admin
This is a lightweight Blob Admin interface to view and debug your Blob data.
Use this button to install the val:
It uses basic authentication with your Val Town API Token as the password (leave the username field blank).
TODO
-
/new
- render a page to write a new blob key and value -
/edit/:blob
- render a page to edit a blob (prefilled with the existing content) -
/delete/:blob
- delete a blob and render success - add upload/download buttons
- Use modals for create/upload/edit/view/delete page (htmx ?)
- handle non-textual blobs properly
- use codemirror instead of a textarea for editing text blobs
Email with GPT-3
Send an email to stevekrouse.emailGPT3@valtown.email, it will forward it to gpt3, and email you back the response.
dlock - free distributed lock as a service
Usage
API
Acquire a lock.
The id path segment is the lock ID - choose your own.
https://dlock.univalent.net/lock/arbitrary-string/acquire?ttl=60
{"lease":1,"deadline":1655572186}
Another attempt to acquire the same lock within its TTL will fail with HTTP status code 409.
https://dlock.univalent.net/lock/01899dc0-2742-44f9-9c7b-01830851b299/acquire?ttl=60
{"error":"lock is acquired by another client","deadline":1655572186}
The previous lock can be renewed with its lease number, like a heartbeat
https://dlock.univalent.net/lock/01899dc0-2742-44f9-9c7b-01830851b299/acquire?ttl=60&lease=1
{"lease":1,"deadline":1655572824}
Release a lock
https://dlock.univalent.net/lock/01899dc0-2742-44f9-9c7b-01830851b299/release?lease=42
SQLite Migrate Table via cloning it into a new table example
There are a lot of migrations that SQLite doesn't allow, such as adding a primary key on a table. The way to accomplish this is by creating a new table with the schema you desire and then copying the rows of the old table into it.
This example shows how to:
- Get the schema for the existing table
- Create the new table
- Copy all rows from old to new
- Rename the old table to an archive (just in case)
- Rename the new table to the original table name
This script shows me adding a primary key constraint to the Profile
column of my DateMeDocs
database. I would console and comment out various parts of it as I went. You can see everything I did in the version history. The main tricky part for me was removing the duplicate primary key entries before doing the migration step, which is a useful thing anyways, from a data cleaning perspective.
SSR React Mini & SQLite Todo App
This Todo App is server rendered and client-hydrated React. This architecture is a lightweight alternative to NextJS, RemixJS, or other React metaframeworks with no compile or build step. The data is saved server-side in Val Town SQLite.
SSR React Mini Framework
This "framework" is currently 44 lines of code, so it's obviously not a true replacement for NextJS or Remix.
The trick is client-side importing the React component that you're server rendering. Val Town is uniquely suited for this trick because it both runs your code server-side and exposes vals as modules importable by the browser.
The tricky part is making sure that server-only code doesn't run on the client and vice-versa. For example, because this val colocates the server-side loader
and action
with the React component we have to be careful to do all server-only imports (ie sqlite) dynamically inside the loader
and action
, so they only run server-side.