Public vals
47
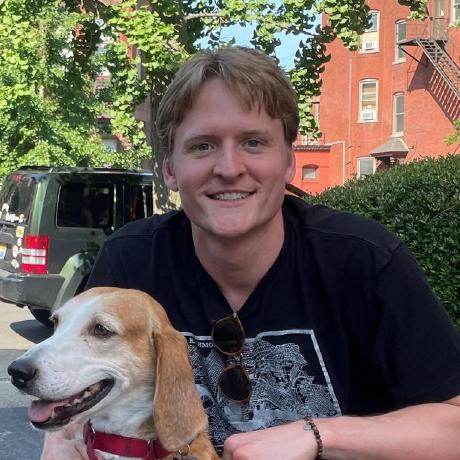
sendNewsletterReminder
@petermillspaugh
Cron
Val Town email subscriptions: newsletter reminder Cousin Val to @petermillspaugh/emailSubscription for emailing yourself a reminder to send your next newsletter. Since this Val is public, anyone can run it. I've commented out the function body that actually emails me to prevent anyone from spamming me, but you can fork this as a private Val to set a cron reminder.
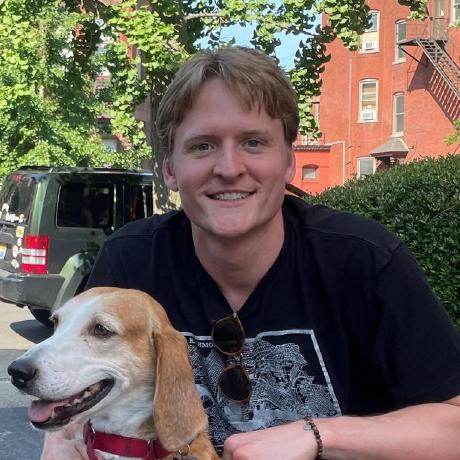
newsletters
@petermillspaugh
Script
Val Town email subscriptions: newsletters Cousin Val to @petermillspaugh/emailSubscription . Process for sending out a newsletter: Publish newsletter on the Web Fork and update monthly newsletter Val like january2024 Add new newsletter to this list Val sendEmailNewsletter cron val will attempt to send latest newsletter on the first of the month
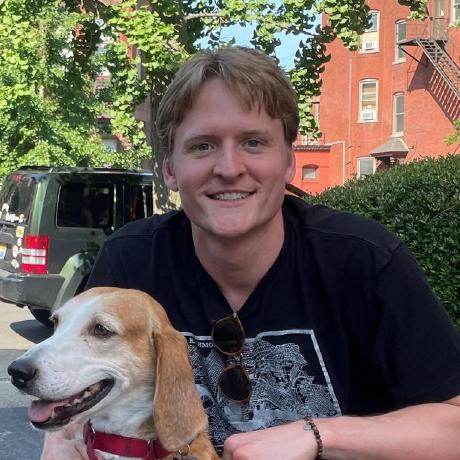
sendTestEmailNewsletter
@petermillspaugh
Cron
Val Town email subscriptions: send test email Cousin Val to @petermillspaugh/emailSubscription — see docs there. When you're writing up an email to send to subscribers, it's helpful to send it to yourself ahead of time to proofread and see how it looks in different email clients etc.
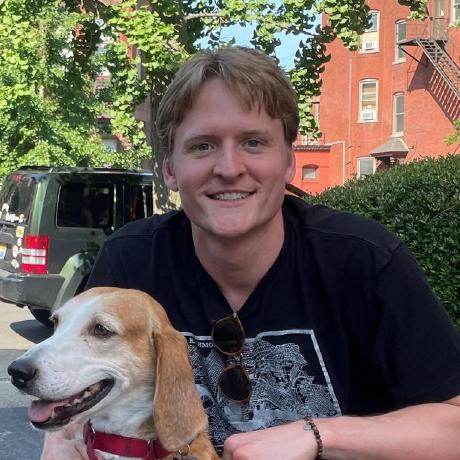
sendEmailNewsletter
@petermillspaugh
Cron
Val Town email subscriptions: send email newsletter Cousin Val to @petermillspaugh/emailSubscription — see docs there. This Val has a few layers of protection to avoid double sending. Those mechanisms feel pretty hacky, so any suggestions are welcome! Feel free to comment on the Val or submit a PR.