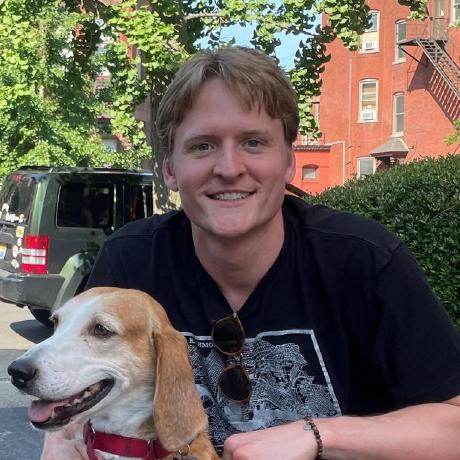
petermillspaugh
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
/** @jsxImportSource https://esm.sh/react */
import { lessons } from "https://esm.town/v/petermillspaugh/lessons";
import { email as sendEmail } from "https://esm.town/v/std/email?v=11";
import { renderToString } from "npm:react-dom/server";
export async function sendLessonResponses({ email, lesson, formData }) {
const fillBlankResponse = formData.get("fill-in-the-blank") as string;
const reflectionResponse = formData.get("reflection") as string;
const jsx = (
<>
<h1>Your responses for lesson {Number(lesson) + 1}: {lessons[lesson].title}</h1>
<h2>Fill-in-the-blank</h2>
<p>
<strong>Prompt:</strong> {lessons[lesson].fillBlank}
</p>
<p>
<strong>Your response:</strong> {fillBlankResponse}
</p>
<h2>Review</h2>
{lessons[lesson].quiz.map(({ question, answer }) => (
<>
<h3>{question}</h3>
<p>
<strong>Your response:</strong> {formData.get(`ANSWER-${question}`) as string}
</p>
<p>
<strong>Answer:</strong> {answer}
</p>
</>
))}
<h2>Reflection</h2>
<p>{reflectionResponse}</p>
</>
);
await sendEmail({
to: email,
from: {
name: "Make It Stick (in 10 days, via email)",
email: "petermillspaugh.sendLesson@valtown.email",
},
replyTo: "pete@petemillspaugh.com",
subject: `Your responses for lesson ${Number(lesson) + 1}: ${lessons[lesson].title}`,
html: renderToString(jsx),
});
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
/** @jsxImportSource https://esm.sh/react */
import { generateLessonHtml } from "https://esm.town/v/petermillspaugh/lessonTemplate";
import { renderToString } from "npm:react-dom/server";
/*
* Note: this lesson is a work in progress 👷♂️
*/
const TITLE = "Learning about learning";
const FILL_BLANK = <>TODO</>;
const CONTENT = (
<>
<h2>Metacognition: what we know about what we know.</h2>
<p>There are known knowns, known unknowns, and unknown unknowns (Rumsfeld).</p>
<p>“Being accurate in your judgement of what you know and don’t know is critical for decision making.”</p>
<p>Cognitive psychology deals in metacognition. It focuses on how we think, perceive, and remember.</p>
<p>
"Cognitive psychology is the basic science of understanding how the mind works, conducting empirical research into
how people percieve, remember, and think."
</p>
<h2>What you've learned about learning</h2>
<p>
This single sentence from <em>Make It Stick</em>{" "}
packs in most of the key themes from the books and the lessons in this course:
</p>
<blockquote>
<em>
“More complex and durable learning comes from self-testing, introducing certain difficulties in practice,
waiting to re-study material until a little forgetting has set in, and interleaving the practice of one skill or
topic with another.”
</em>
</blockquote>
<p>
Self-testing is <em>retrieval practice</em>. Introducing certain difficulties is{" "}
<em>generation</em>. Waiting to re-study material is{" "}
<em>spaced repetition</em>. And lastly, interleaving one skill or topic with another is, of course,{" "}
<em>interleaving</em>.
</p>
<h2>Books</h2>
<ul>
<li>Mindset by Carol Dweck</li>
<li>Thinking Fast and Slow by Daniel Kahneman</li>
<li>TODO</li>
</ul>
</>
);
const QUIZ = [
{
question: "",
answer: "",
},
];
export const learningAboutLearning = {
title: TITLE,
fillBlank: FILL_BLANK,
quiz: QUIZ,
fetchHtml: (email: string, lesson: number) =>
generateLessonHtml({
email,
lesson,
title: `Lesson ${lesson + 1}: ${TITLE}`,
fillBlank: FILL_BLANK,
content: CONTENT,
quiz: QUIZ,
}),
};
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
/** @jsxImportSource https://esm.sh/react */
import { generateLessonHtml } from "https://esm.town/v/petermillspaugh/lessonTemplate";
import { renderToString } from "npm:react-dom/server";
/*
* Note: this lesson is a work in progress 👷♂️
*/
const TITLE = "Your brain";
const FILL_BLANK = <></>;
const CONTENT = (
<>
<h2>Neurons</h2>
<p>
Humans are born with about 100 billion neurons (TODO: source).
</p>
<p>
Axons and dendrites.
TODO: great diagram here from Anki
</p>
<p>
Myelin sheath, myelination. Myelin sheath is the fatty layer around axons on neurons. Thicker, more developed
myelin sheath allows stronger and faster signals to transmit between synapses, which correlates with more advanced
skill or knowledge. Myelineation refers to the development of myelin sheath.
TODO: great diagram here from Anki
</p>
<h2>Synapses</h2>
<p>
Synapses are connections between neurons that transmit electrical signal. An axon on one neuron connects to a
dendrite on another neuron to form a synapse.
</p>
<h2>Neurogenesis</h2>
<p>
Neurogenesis is the process of creating new neurons. It mostly happens in prenatal development but also in the
hippocampus during adulthood to facilitate learning.
</p>
<h2>Synaptic pruning</h2>
<p>
Humans reach a peak number of synapses around age 1 or 2, plateau until puberty, then undergo synaptic pruning
until adulthood.
There's still research to be done on why certain neural pathways are pruned. The "use it or lose it" camp
hypothesizes that pathways that aren't used during childhood get pruned.
</p>
<h2>Neuroplasticity</h2>
<p>
Neuroplasticity refers to the brain’s ability to change, e.g. forming new pathways between neurons and
strengthening/weakening synaptic connections.
</p>
<h2>The hippocampus</h2>
<p>The hippocampus performs consolidation of short-term memory into long-term memory.</p>
<h2>The basal ganglia</h2>
<p>
The basal ganglia, which also controls some motor function—both voluntary and unvoluntary, like subconscious eye
movements, Cognitive and motor functions can be chunked together in one unit that makes up a learned habit or
skill. So you can think of learned habits become automatic, uncontrolled System 1 knowledge.
It kind of looks like an over-ear bluetooth thingy. TODO: image/diagram
</p>
</>
);
const QUIZ = [
{
question: "",
answer: "",
},
];
export const yourBrain = {
title: TITLE,
fillBlank: FILL_BLANK,
quiz: QUIZ,
fetchHtml: (email: string, lesson: number) =>
generateLessonHtml({
email,
lesson,
title: `Lesson ${lesson + 1}: ${TITLE}`,
fillBlank: FILL_BLANK,
content: CONTENT,
quiz: QUIZ,
}),
};
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
/** @jsxImportSource https://esm.sh/react */
import { generateLessonHtml } from "https://esm.town/v/petermillspaugh/lessonTemplate";
import { renderToString } from "npm:react-dom/server";
/*
* Note: this lesson is a work in progress 👷♂️
*/
const TITLE = "Growth mindset";
const FILL_BLANK = <>TODO</>;
const CONTENT = (
<>
<blockquote>Whether you think you can or you think you can't, you're right.</blockquote>
<h2>Growth mindset</h2>
<p></p>
</>
);
const QUIZ = [
{
question: "",
answer: "",
},
];
export const growthMindset = {
title: TITLE,
fillBlank: FILL_BLANK,
quiz: QUIZ,
fetchHtml: (email: string, lesson: number) =>
generateLessonHtml({
email,
lesson,
title: `Lesson ${lesson + 1}: ${TITLE}`,
fillBlank: FILL_BLANK,
content: CONTENT,
quiz: QUIZ,
}),
};
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
/** @jsxImportSource https://esm.sh/react */
import { generateLessonHtml } from "https://esm.town/v/petermillspaugh/lessonTemplate";
import { renderToString } from "npm:react-dom/server";
/*
* Note: this lesson is a work in progress 👷♂️
*/
const TITLE = "Generation";
const FILL_BLANK = <>TODO</>;
const CONTENT = (
<>
<h2>Generation</h2>
<p>
Generation is when you attempt to solve a problem before being presented with the solution or relevant knowledge.
Generation produces more durable learning.
</p>
<p>Trying to solve a problem before you know the solution is an effective technique.</p>
<p>Desirable difficulties.</p>
<p>
This course uses generation in the pre-lesson exercise.
</p>
<p>
Learning is more durable when it’s effortful. Josh Comeau uses this tactic in his{" "}
<a href="https://www.joshwcomeau.com/courses/">courses</a>, challenging students to solve a coding exercise before
he introduces the relevant content.
</p>
<h2>The case for writing</h2>
<p>
The act of writing allows you to elaborate and reflect on what you've learned (or know), often forging new
connections between related mental models. It often includes retrieval practice as you recall the information
while translating your thoughts to paper. Writing can also expose gaps and fuzzy spots in your knowledge, forcing
you to refine what you know.
</p>
<p>
Chapter 4 of <em>Make It Stick</em>{" "}
on embracing difficulties includes the story of "The Blundering Gardener" Bonnie Blodgett who became an expert
gardener by getting her hands dirty and learning things one at a time by trial and error. Bonnie also writes
extensively about her gardening in a quarterly called <em>The Garden Letter</em>. The book notes that "in{" "}
<em>writing</em>{" "}
about her experiences, Bonnie is engaging in two potent learning processes beyond the act of gardening itself. She
is retrieving the details and story of what she has discovered—say, about an experiment in grafting two species of
fruit trees—and then she is elaborating by explainging the experience to her readers, connecting the outcome to
what she already knows about the subject or has learned as a result."
</p>
</>
);
const QUIZ = [
{
question: "",
answer: "",
},
];
export const generation = {
title: TITLE,
fillBlank: FILL_BLANK,
quiz: QUIZ,
fetchHtml: (email: string, lesson: number) =>
generateLessonHtml({
email,
lesson,
title: `Lesson ${lesson + 1}: ${TITLE}`,
fillBlank: FILL_BLANK,
content: CONTENT,
quiz: QUIZ,
}),
};
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
/** @jsxImportSource https://esm.sh/react */
import { generateLessonHtml } from "https://esm.town/v/petermillspaugh/lessonTemplate";
import { renderToString } from "npm:react-dom/server";
/*
* Note: this lesson is a work in progress 👷♂️
*/
const TITLE = "Encoding & consolidation";
// TODO: better prompt
const FILL_BLANK = <>Where are memories stored in your brain?</>;
const CONTENT = (
<>
<h2>Memory traces</h2>
<p>
A memory trace is a metaphorical/theoretical term for the brain’s representation of some knowledge or memory. The
“trace” includes synaptic connections between neurons. Also known as an “engram”.
</p>
{/* TODO: maybe this should be included in an earlier lesson */}
<h2>Mental models</h2>
<p>
"A mental model is a mental representation of some external reality." Fitting new knowledge into an existing
mental model or creating a new mental model that connects to another existing one is key for learning.
</p>
<p>
Placing new knowledge in a larger context aids learning. I've termed this <em>contextualization</em>{" "}
so that I have a label to attach to the concept, although that's not an official term. Same idea as fitting new
learnings into an existing mental model.
</p>
<p>
You can fit new knowledge into an existing mental model, and that connection improves retention. Or you might
create a new mental model that connects to another existing mental model.
</p>
<p>"All new learning requires a foundation of prior knowledge." Learn the fundamentals!</p>
<p>Identifying underlying rules helps solve related but unfamiliar problems later.</p>
<h2>Encoding</h2>
<p>
Encoding is the process of transforming sensory perceptions—things we read, hear, see, feel—into memory traces in
the brain. Encoding places new learning in short-term memory, and consolidation moves it to long-term memory.
</p>
<h2>Memory consolidation</h2>
<p>
Memory consolidation is the process of transforming short-term memories into long term memories. It happens over
the course of hours and days, including during deep, slow-wave sleep. During consolidation, memory traces are
strengthened and connected to prior knowledge. The hippocampus performs consolidation of short-term memory into
long-term memory.
</p>
<h2>Synaptic pruning</h2>
</>
);
const QUIZ = [
{
question: "",
answer: "",
},
];
export const encodingAndConsolidation = {
title: TITLE,
fillBlank: FILL_BLANK,
quiz: QUIZ,
fetchHtml: (email: string, lesson: number) =>
generateLessonHtml({
email,
lesson,
title: `Lesson ${lesson + 1}: ${TITLE}`,
fillBlank: FILL_BLANK,
content: CONTENT,
quiz: QUIZ,
}),
};
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
/** @jsxImportSource https://esm.sh/react */
import { generateLessonHtml } from "https://esm.town/v/petermillspaugh/lessonTemplate";
import { renderToString } from "npm:react-dom/server";
/*
* Note: this lesson is a work in progress 👷♂️
*/
const TITLE = "Elaboration & reflection";
const FILL_BLANK = <>TODO</>;
const CONTENT = (
<>
<h2>Elaboration</h2>
<p>
Elaboration is the process of explaining newly learned things in your own words and making connections to stuff
you already know. It’s an effective learning technique.
</p>
<p>
Elaboration strengthens learning. That’s when you explain something you’ve learned in different terms, connecting
it to something else you know.
</p>
<h2>Reflection</h2>
<p>
Writing down questions or mentally going through what you’ve learned after you learn it. Reflection aids learning.
</p>
<p>That's why each lesson ends with a reflection writing exercise.</p>
</>
);
const QUIZ = [
{
question: "",
answer: "",
},
];
export const elaborationAndReflection = {
title: TITLE,
fillBlank: FILL_BLANK,
quiz: QUIZ,
fetchHtml: (email: string, lesson: number) =>
generateLessonHtml({
email,
lesson,
title: `Lesson ${lesson + 1}: ${TITLE}`,
fillBlank: FILL_BLANK,
content: CONTENT,
quiz: QUIZ,
}),
};
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
/** @jsxImportSource https://esm.sh/react */
import { generateLessonHtml } from "https://esm.town/v/petermillspaugh/lessonTemplate";
import { renderToString } from "npm:react-dom/server";
/*
* Note: this lesson is a work in progress 👷♂️
*/
const TITLE = "Varied practice";
const FILL_BLANK = (
<>
Two basketball players want to improve their 3-point shooting with a weekly routine. The first player takes 100
shots from a different location on the court each day Monday to Friday (e.g. 100 shots from the left corner on the
first day, 100 shots from the wing on the second day, 100 shots from the top of the arc on the third day, and so
on). The second player takes 20 shots from 5 locations{" "}
<em>every day</em>. So both players take 100 shots per day, totaling 500 shots each week. Which routine do you think
would be more effective?
</>
);
const CONTENT = (
<>
<h2>Varied practice versus massed practice</h2>
<p>
Is massed practice–focusing study on one topic for a concentrated time period–effective? No. Learning gains are
transitory.
</p>
<p>
Varied practice is more effective than massed practice and is consolidated in a different part of the brain that
encodes higher-order, more flexible learning.
</p>
<h2>Varied practice in sports</h2>
<p>TODO: beanbag toss experiment</p>
<p>TODO: basketball example</p>
<h2>Flexible memory storage in your brain</h2>
<p>TODO</p>
</>
);
const QUIZ = [
{
question: "",
answer: "",
},
];
export const variedPractice = {
title: TITLE,
fillBlank: FILL_BLANK,
quiz: QUIZ,
fetchHtml: (email: string, lesson: number) =>
generateLessonHtml({
email,
lesson,
title: `Lesson ${lesson + 1}: ${TITLE}`,
fillBlank: FILL_BLANK,
content: CONTENT,
quiz: QUIZ,
}),
};
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
/** @jsxImportSource https://esm.sh/react */
import { generateLessonHtml } from "https://esm.town/v/petermillspaugh/lessonTemplate";
import { renderToString } from "npm:react-dom/server";
/*
* Note: this lesson is a work in progress 👷♂️
*/
const TITLE = "Interleaving";
const FILL_BLANK = <>TODO</>;
const CONTENT = (
<>
<h2>Interleaving</h2>
<p>
Interleaving the study of different topics results in more effective learning than studying distinct topics
sequentially.
</p>
<p>
Does interleaving yield better short-term learning? No, but it does yield better long-term learning.
</p>
<p>Interleaving different but related topics. Connects our mental models together.</p>
</>
);
const QUIZ = [
{
question: "",
answer: "",
},
];
export const interleaving = {
title: TITLE,
fillBlank: FILL_BLANK,
quiz: QUIZ,
fetchHtml: (email: string, lesson: number) =>
generateLessonHtml({
email,
lesson,
title: `Lesson ${lesson + 1}: ${TITLE}`,
fillBlank: FILL_BLANK,
content: CONTENT,
quiz: QUIZ,
}),
};
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
/** @jsxImportSource https://esm.sh/react */
import { generateLessonHtml } from "https://esm.town/v/petermillspaugh/lessonTemplate";
import { renderToString } from "npm:react-dom/server";
/*
* Work-in-progress! 👷♂️
*/
const TITLE = "Retrieval practice";
const FILL_BLANK = (
<>
We often hear things like, "I am a visual learner," or "the way I learn best is by re-reading my notes and
underlining key concepts." Do you think there is any merit to personal preference of learning style? Why or why not?
</>
);
const CONTENT = (
<>
<h2>About this course</h2>
<p>
The main purpose of this course is to teach you about about effective, research-backed learning techniques covered
in the book{" "}
<a href="https://www.goodreads.com/book/show/18770267-make-it-stick">
<em>Make It Stick</em>
</a>. The secondary purpose of this course is to provide a copyable template for creating email-based courses with
a structure informed by effective learning techniques covered in that book. If you know some JavaScript/HTML and
are interested in that, all the code is public and{" "}
<a href="https://petemillspaugh.com/email-course-creator">
I wrote about the implementation on my digital garden
</a>.
</p>
<p>
As a disclaimer, I am not a learning expert. I like to learn, and I like learning about learning. As mentioned
just above, this course is a distillation of{" "}
<em>Make It Stick</em>, supported by some follow-up research I did. It's also a way for me to strengthen what I
learned from the book. If you have any feedback on the course please shoot me an email at
pete@petemillspaugh.com—I'm open to suggestions, corrections, or anything else you'd like to share.
</p>
{/* <h2>Is rereading an effective learning tactic?</h2> */}
{/* <p>Massed practice</p> */}
{/* <p>Rereading is not effective.</p> */}
{
/* <p>
We are poor judges of when we are learning well and when we are not. A good example of this for me is when I take
an online coding course or tutorial on a new technology/tool, writing lots of caffeine-fueled notes and not
self-testing nor applying that new technology to a project. The good feeling from cruising through content and
taking notes is illusory—durable learning requires retrieval and application.
</p> */
}
<h2>📣 This course is still a work-in-progress 📣</h2>
<p>
Thank you for signing up to test things out! I'm still working on the course content, so this lesson is
incomplete, but lmk any thoughts or suggestions you have in the meantime.
</p>
<h2>What is retrieval practice?</h2>
<p>
<em>Retrieval practice</em>{" "}
is cognitive psychology's term for the act of recalling something from memory. Studying flashcards is a very
structured form of retrieval practice, but we retrieve things all the time—like when a friend asks you about the
book you're reading, when you cook a recipe without referencing instructions, or when speaking in another language
that you don't use often.
</p>
<p>
As chapter 2 of <em>Make It Stick</em>—"To Learn, Retrieve"—puts it:
</p>
<blockquote>
<code>
Retrieval practice—recalling facts or concepts or events from memory—is a more effective learning strategy than
review by rereading. Flashcards are a simple example. Retrieval strengthens the memory and interrupts
forgetting. A single, simple quiz after reading a text or hearing a lecture produces better learning and
remembering than rereading the text or reviewing lecture notes.
</code>
</blockquote>
<p>
Each lesson in this course starts with a fill-in-the-blank question and ends with a set of review questions and
reflection. This structure is deliberately designed to force retrieval practice. Not only that, but retrieval
should be spaced, which is the topic of the next lesson and the reason this course includes a one-day delay before
sending the following lesson. From chapter 2 of the book:
</p>
<blockquote>
<code>
When retrieval practice is spaced, allowing some forgetting to occur between tests, it leads to stronger long-
term retention than when it is massed.
</code>
</blockquote>
<h2>Brain food: retrieval as reconsolidation</h2>
{/* <p>Your brain reconsolidates memory upon recall.</p> */}
<p>From the book:</p>
<blockquote>
<code>
While the brain is not a muscle that gets stronger with exercise, the neural pathways that make up a body of
learning do get stronger, when the memory is retrieved and the learning is practiced. Periodic practice arrests
forgetting, strengthens retrieval routes, and is essential for hanging onto the knowledge you want to gain.
</code>
</blockquote>