Back to APIs list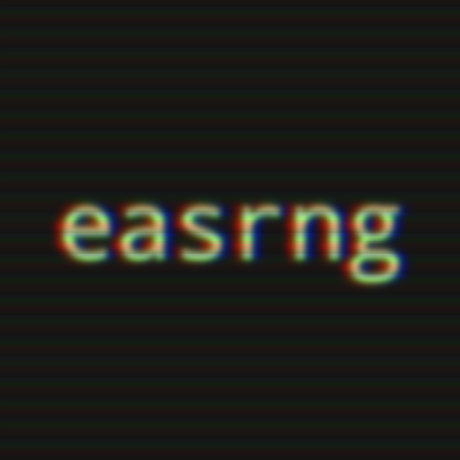
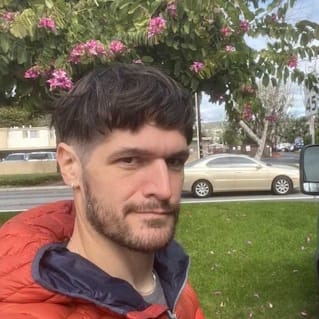
Matrix API examples & templates
Use these vals as a playground to view and fork Matrix API examples and templates on Val Town. Run any example below or find templates that can be used as a pre-built solution.
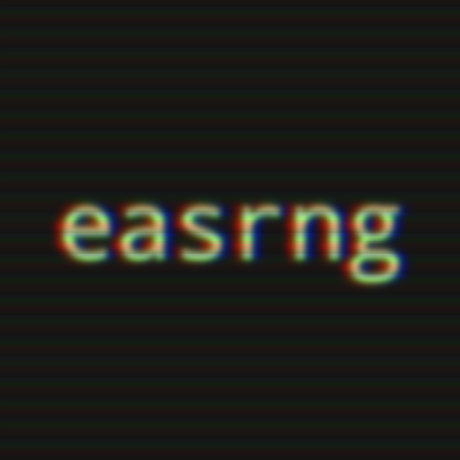
playground
@easrng
HTTP
playground edit, run, and embed vals without requiring an account (or even js enabled!) caveats: logs don't stream I haven't set up codemirror only script vals supported everything else should be fully functional. you can prefill the editor with code:
https://easrng-playground.web.val.run/?code=console.log(1) a val:
https://easrng-playground.web.val.run/?load=easrng/playground some other url: https://easrng-playground.web.val.run/?load=https://any/other/url
animatedReadmeSVG
@maxm
HTTP
Fancy animated SVGs in readmes, along with centering and image sizing. <div align="center"><img width=200 src="https://gpanders.com/img/DEC_VT100_terminal.jpg"></div> <p align="center">
<img src="https://maxm-animatedreadmesvg.web.val.run/comet.svg" />
</p> <p align="center">
<img src="https://maxm-animatedreadmesvg.web.val.run/custom text!" />
</p>