Public vals
48
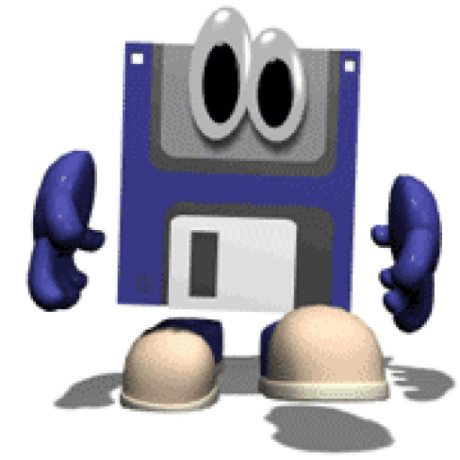
telemetry
@saolsen
Script
Telemetry For Vals. Telemetry is a library that lets you trace val town executions with opentelemetry. All traces are stored in val.town sqlite and there is an integrated trace viewer to see them. Quickstart Instrument an http val like this. import {
init,
tracedHandler,
} from "https://esm.town/v/saolsen/telemetry";
// Set up tracing by passing in `import.meta.url`.
// be sure to await it!!!
await init(import.meta.url);
async function handler(req: Request): Promise<Response> {
// whatever else you do.
return
}
export default tracedHandler(handler); This will instrument the http val and trace every request. Too add additional traces see this widgets example . Then, too see your traces create another http val like this. import { traceViewer } from "https://esm.town/v/saolsen/telemetry";
export default traceViewer; This val will serve a UI that lets you browse traces. For example, you can see my UI here . Tracing By wrapping your http handler in tracedHandler all your val executions will be traced. You can add additional traces by using the helpers. trace lets you trace a block of syncronous code. import { trace } from "https://esm.town/v/saolsen/telemetry";
trace("traced block", () => {
// do something
}); traceAsync lets you trace a block of async code. import { traceAsync } from "https://esm.town/v/saolsen/telemetry";
await traceAsync("traced block", await () => {
// await doSomething();
}); traced wraps an async function in tracing. import { traceAsync } from "https://esm.town/v/saolsen/telemetry";
const myTracedFunction: () => Promise<string> = traced(
"myTracedFunction",
async () => {
// await sleep(100);
return "something";
},
); fetch is a traced version of the builtin fetch function that traces the request. Just import it and use it like you would use fetch . sqlite is a traced version of the val town sqlite client. Just import it and use it like you would use https://www.val.town/v/std/sqlite attribute adds an attribute to the current span, which you can see in the UI. event adds an event to the current span, which you can see in the UI.