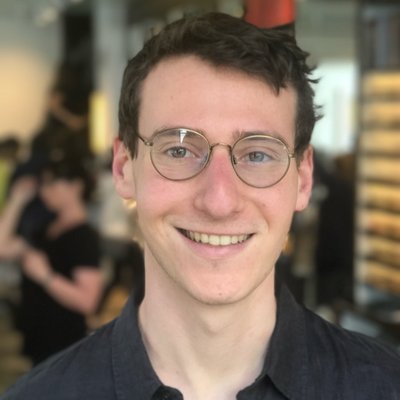
stevekrouse
CronGPT
This is a minisite to help you create cron expressions, particularly for crons on Val Town. It was inspired by Cron Prompt, but also does the timezone conversion from wherever you are to UTC (typically the server timezone).
Tech
- Hono for routing (
GET /
andPOST /compile
.) - Hono JSX
- HTMX (probably overcomplicates things; should remove)
- @stevekrouse/openai, which is a light wrapper around @std/openai
I'm finding HTMX a bit overpowered for this, so I have two experimental forks without it:
- Vanilla client-side JavaScript: @stevekrouse/cron_client_side_script_fork
- Client-side ReactJS (no SSR): @stevekrouse/cron_client_react_fork
I think (2) Client-side React without any SSR is the simplest architecture. Maybe will move to that.
This is an example call of @stevekrouse/insecureFetch
Starter App for ssr_react_mini
You need to export four things:
loader
- runs on any GET request, on the server. it accepts theRequest
and returns the props of your React compnent.action
- runs on the server on any non-GET, ie POST, PUT, DELETE, or<form>
s submitComponent
- your React component. it's initially server-rendered and then client-hydrateddefault
- you should mostly leave this line alone
This is framework is bleeding-edge. You'll need to read the code of the framework itself (it's very short) to understand what it's doing.
If you have questions or comments, please comment below on this val! (or any of these vals)
Date Me Directory
This is entry-point val for the source code for the Date Me Directory. Contributions welcome!
This app uses Hono as the server framework and for JSX.
The vals are stored in Val Town SQLite.
Contributing
Forking this repo should mostly work, except for the sqlite database. You'll need to create the table & populate it with some data. This script should do it, but I think it has a couple bugs. If you're interested in contributing to this project contact me or comment on this val and I'll get it working for ya!
Todos
- Make the SQLite database forkable and build a widget/workflow for that, ie fix @stevekrouse/dateme_sqlite
- Require an email (that isn't shared publicly)
- Verify the email address with a "magic link"
- Refactor Location to an array of Lat, Lon
- Geocode all the existing locations
- Add a geocoder map input to the form
- Allow selecting multiple location through the form
- Profile performance & speed up site, possibly add more caching
- Let people edit their forms
- Featured profiles
SQLite Admin
This is a lightweight SQLite Admin interface to view and debug your SQLite data.
It's currently super limited (no pagination, editing data, data-type specific viewers), and is just a couple dozens lines of code over a couple different vals. Forks encouraged! Just comment on the val if you add any features that you want to share.
To use it on your own Val Town SQLite database, fork it to your account.
It uses basic authentication with your Val Town API Token as the password (leave the username field blank).
GPT4 Example
This uses the brand new gpt-4-1106-preview
.
To use this, set OPENAI_API_KEY
in your Val Town Secrets.