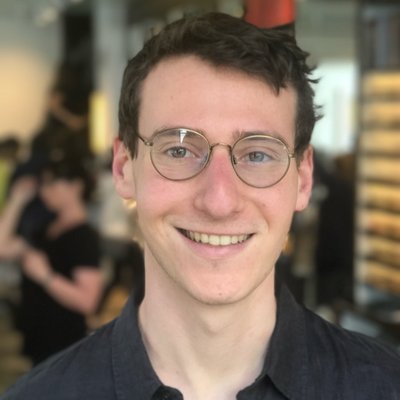
@stevekrouse
SSR React Mini & SQLite Todo App
This Todo App is server rendered and client-hydrated React. This architecture is a lightweight alternative to NextJS, RemixJS, or other React metaframeworks with no compile or build step. The data is saved server-side in Val Town SQLite.
SSR React Mini Framework
This "framework" is currently 44 lines of code, so it's obviously not a true replacement for NextJS or Remix.
The trick is client-side importing the React component that you're server rendering. Val Town is uniquely suited for this trick because it both runs your code server-side and exposes vals as modules importable by the browser.
The tricky part is making sure that server-only code doesn't run on the client and vice-versa. For example, because this val colocates the server-side loader
and action
with the React component we have to be careful to do all server-only imports (ie sqlite) dynamically inside the loader
and action
, so they only run server-side.
☔️ Umbrella reminder if there's rain today
Setup
- Fork this val 👉 https://val.town/v/stevekrouse.umbrellaReminder/fork
- Customize the
location
(line 8). You can supply any free-form description of a location.
⚠️ Only works for US-based locations (where weather.gov covers).
How it works
- Geocodes an free-form description of a location to latitude and longitude – @stevekrouse.nominatimSearch
- Converts a latitude and longitude to weather.gov grid – @stevekrouse.weatherGovGrid
- Gets the hourly forecast for that grid
- Filters the forecast for periods that are today and >30% chance of rain
- If there are any, it formats them appropriately, and sends me an email
Planes Above Me
Inspired by https://louison.substack.com/p/i-built-a-plane-spotter-for-my-son
A little script that grabs that planes above you, just change line 4 to whatever location you want and it'll pull the lat/log for it and query.
Message yourself on Telegram
This val lets you send yourself Telegram messages via ValTownBot. This ValTownBot saves you from creating your own Telegram Bot.
However if I'm being honest, it's really simple and fun to make your own Telegram bot. (You just message BotFather.) I'd recommend most folks going that route so you have an unmediated connection to Telegram. However if you want to have the simplest possible setup to just send yourself messages, read on...
Installation
It takes less than a minute to set up!
-
Start a conversation with ValTownBot
-
Copy the secret it gives you
-
Save it in your Val Town Environment Variables under
telegram
-
Send a message!
Usage
telegramText
Create valimport { telegramText } from "https://esm.town/v/stevekrouse/telegram?v=14";
const statusResponse = await telegramText("Hello from Val.Town!!");
console.log(statusResponse);
telegramPhoto
Create valimport { telegramPhoto } from "https://esm.town/v/stevekrouse/telegram?v=14";
const statusResponse = await telegramPhoto({
photo: "https://placekitten.com/200/300",
});
console.log(statusResponse);
ValTownBot Commands
/roll
- Roll your secret in case you accidentally leak it./webhook
- Set a webhook to receive messages you send to @ValTownBot
Receiving Messages
If you send /webhook
to @ValTownBot, it will let you specify a webhook URL. It will then forward on any messages (that aren't recognized @ValTownBot commands) to that webhook. It's particularly useful for creating personal chatbots, like my telegram <-> DallE bot.
How it works
Telegram has a lovely API.
- I created a @ValTownBot via Bot Father.
- I created a webhook and registered it with telegram
- Whenever someone new messages @ValTownBot, I generate a secret and save it along with their Chat Id in @stevekrouse/telegramValTownBotSecrets (a private val), and message it back to them
- Now whenever you call this val, it calls
telegramValTownAPI
, which looks up your Chat Id via your secret and sends you a message
Telegram Resources
- Val Town Telegram Echo Bot Guide
- Telegram <-> DallE Bot
- Bot Father - the father of all Telegram Bots
- Telegram Bot Tutorial
Credits
This val was originally made by pomdtr.
Todo
- Store user data in Val Town SQLite
- Parse user data on the API side using Zod
Get all the pages in a notion database
Usage
- Find your
databaseId
: https://developers.notion.com/reference/retrieve-a-database - Get
auth
by setting up an internal integration: https://developers.notion.com/docs/authorization#internal-integration-auth-flow-set-up
Example usage: @stevekrouse.dateMeNotionDatabase
deno-notion-sdk docs: https://github.com/cloudydeno/deno-notion_sdk
Poll RSS feeds
This val periodically polls specified RSS feeds and send the author an email with new items. It checks each feed defined in rssFeeds
for new content since the last run and sends an email with the details of the new items.
Usage
- Fork @stevekrouse/rssFeeds and update it with your desired RSS feeds;
- Fork this val and replace the
https://esm.town/v/stevekrouse/rssFeeds
import with your newly forked val; - Enjoy RSS updates on your email!
This is an example call of @stevekrouse/insecureFetch
Starter App for ssr_react_mini
You need to export four things:
loader
- runs on any GET request, on the server. it accepts theRequest
and returns the props of your React compnent.action
- runs on the server on any non-GET, ie POST, PUT, DELETE, or<form>
s submitComponent
- your React component. it's initially server-rendered and then client-hydrateddefault
- you should mostly leave this line alone
This is framework is bleeding-edge. You'll need to read the code of the framework itself (it's very short) to understand what it's doing.
If you have questions or comments, please comment below on this val! (or any of these vals)