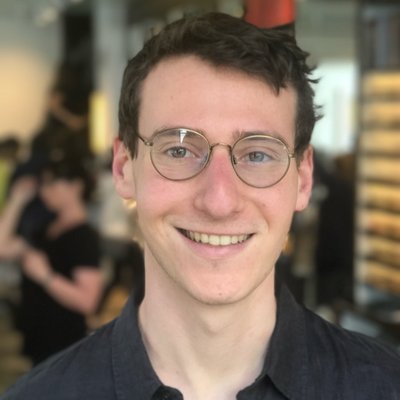
Public
Like
4
company
Val Town is a collaborative website to build and scale JavaScript apps.
Deploy APIs, crons, & store data – all from the browser, and deployed in miliseconds.
This val forwards emails to addresses that don't exist to all of us at Val Town. For example, this forwards feedback@val.town
to all of us. We achieve this by forwarding emails to this email handler, and this email handler forwards them along.
To accomplish this without Val Town would require setting up a Google Group. I prefer doing it in code. Over time we will have more complex routing here.
Migrated from folder: Archive/company
Email