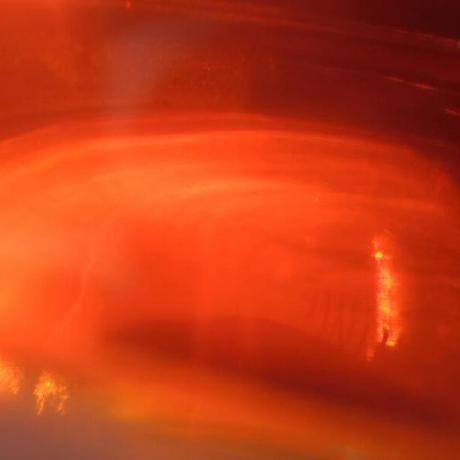
dpetrouk
12 public vals
Joined July 14, 2023
1
2
3
4
5
6
7
8
9
10
11
12
13
import { fetch } from "https://esm.town/v/std/fetch";
import { normalizeURL } from "https://esm.town/v/stevekrouse/normalizeURL?v=3";
export async function fetchTweet(url) {
const tweetId = url.match(/(\d{19})/)[1];
const tweetUrl =
`https://cdn.syndication.twimg.com/tweet-result?id=${tweetId}&lang=en`;
const res = await fetch(normalizeURL(tweetUrl), {
redirect: "follow",
});
const tweetData = await res.json();
return tweetData;
}
Usage in bash:
# Can be in .bashrc or .zsrhc:
to-tg() {
local input=""
if [[ -p /dev/stdin ]]; then
input="$(cat -)"
else
input="${@}"
fi
if [[ -z "${input}" ]]; then
return 1
fi
local chat_id="-1001826545120" # Set chat_id where your bot is
local message="$input"
curl -G https://api.val.town/v1/run/dpetrouk.notifyInTg --data-urlencode 'args=["'"$chat_id"'", "'"$message"'"]'
}
# You will get notification where command is succesfully finished
<command in bash> && to-tg Success
# Can be like that?
<command in bash> | to-tg Success
1
2
3
4
5
6
7
8
9
10
import process from "node:process";
import { telegramSendMessage } from "https://esm.town/v/vtdocs/telegramSendMessage?v=5";
export async function notifyInTg(chatId, text) {
telegramSendMessage(
process.env.telegramBotToken,
{ chat_id: chatId, text },
);
return `Sent message '${text}' to Telegram chat ${chatId}`;
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
import process from "node:process";
import { telegramSetWebhook } from "https://esm.town/v/vtdocs/telegramSetWebhook?v=2";
export const setWebhookExample = telegramSetWebhook(
process.env.telegramBotToken,
{
// Replace this with _your_ express endpoint
url: "https://dpetrouk-telegramWebhookEchoMessage.express.val.run",
// Optionally, filter what kind of updates you want to receive here
allowed_updates: ["message"],
secret_token: process.env.telegramWebhookSecret,
},
);
// Forked from @vtdocs.setWebhookExample
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
import { telegramSendMessage } from "https://esm.town/v/vtdocs/telegramSendMessage?v=5";
import { fetchTweet } from "https://esm.town/v/dpetrouk/fetchTweet";
import process from "node:process";
export const telegramWebhookEchoMessage = async (
req: express.Request,
res: express.Response,
) => {
// Verify this webhook came from our bot
if (
req.get("x-telegram-bot-api-secret-token") !==
process.env.telegramWebhookSecret
) {
return res.status(401);
}
// Echo back the user's message
const { created_at, text, user } = await fetchTweet(
req.body.message.text,
);
const dateTime = created_at.slice(0, 19).split("T").join(" ");
const messageText = [
[dateTime, `${user.name} • ${user.screen_name}`].join("\n"),
text.replaceAll(req.body.message.text, ""),
].join("\n\n");
const chatId: number = req.body.message.chat.id;
telegramSendMessage(
process.env.telegramBotToken,
{ chat_id: chatId, text: messageText },
);
};
// Forked from @vtdocs.telegramWebhookEchoMessage
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
import { fetch } from "https://esm.town/v/std/fetch";
export async function fetchTimeline(url, options) {
// const tweetId = url.match(/\/(\d*)\?+/)[1];
// const tweetUrl =
// `https://cdn.syndication.twimg.com/tweet-result?id=${tweetId}&lang=en`;
// return { url, tweetId, tweetUrl };
const res = await fetch(
"https://syndication.twimg.com/srv/timeline-profile/screen-name/patttten",
// "https://cdn.syndication.twimg.com/timeline/profile?screen_name=patttten",
{
redirect: "follow",
...(options || {}),
},
);
const text = await res.text();
return text;
// const timelineHTML = await @stevekrouse.parseHTML(text);
// const data = timelineHTML.getElementById("__NEXT_DATA__").textContent;
// return JSON.parse(data);
}
1
2
3
4
5
6
7
import { fetchJSON } from "https://esm.town/v/dpetrouk/fetchJSON";
export let untitled_pinkDamselfly = async () => {
return await fetchJSON(
"https://twitter.com/__drewface/status/1664273547764830208?s=20",
);
};
1
2
3
4
5
6
7
8
// View at https://stevekrouse-expressHTMLExample.express.val.run?name=Steve
export async function expressHTMLExample(
req: express.Request,
res: express.Response,
) {
return res.send(`<h1>Hi ${req.query.name || req.body.name}!</h1>`);
}
// Forked from @stevekrouse.expressHTMLExample
1
2
3
4
5
6
7
8
9
10
export let untitled_silverKrill = (name) => {
return `
<html>
<head></head>
<body>
<h1>${name}</h1>
</body>
</html>
`;
};
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
import { fetch } from "https://esm.town/v/std/fetch";
import { normalizeURL } from "https://esm.town/v/stevekrouse/normalizeURL?v=3";
export const fetchJSON = async (url: string, options?: any) => {
let f = await fetch(normalizeURL(url), {
redirect: "follow",
...options,
headers: {
"Content-Type": "application/json",
...(options?.headers || {}),
},
});
let t = await f.text();
try {
return JSON.parse(t);
}
catch (e) {
throw new Error(`fetchJSON error: ${e.message} in ${url}\n\n"${t}"`);
}
};
// Forked from @stevekrouse.fetchJSON
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
import { fetch } from "https://esm.town/v/std/fetch";
export const proxyFetch = async (req, res) => {
const { url, options } = req.body;
try {
const response = await fetch(url, options);
return res.status(response.status).send(await response.text());
}
catch (e) {
const errorMessage = e instanceof Error ? e.message : "Unknown error";
console.error("Failed to initiate fetch", e);
return res.status(500).send(`Failed to initiate fetch: ${errorMessage}`);
}
};
// Forked from @alp.proxyFetch