Back to packages list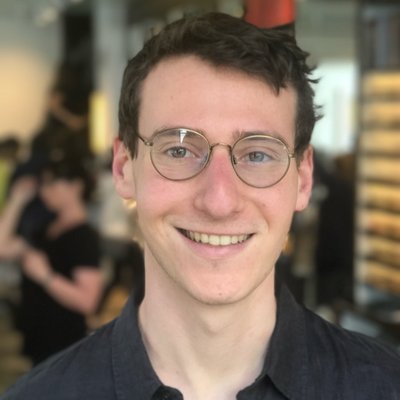
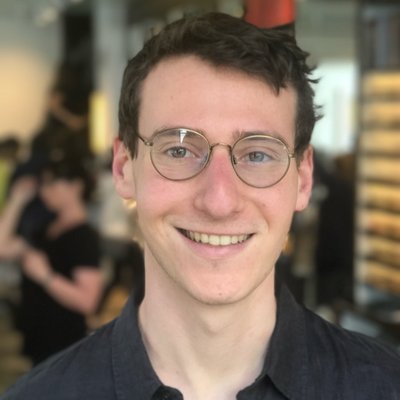
Vals using pako
Description from the NPM package:
zlib port to javascript - fast, modularized, with browser support
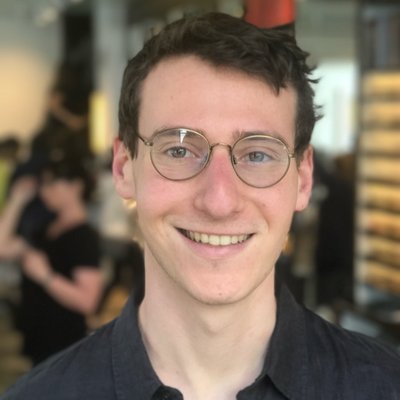
beigeSawfish
@stevekrouse
HTTP
Compress Response Isn't it cool that browsers can natively decompress stuff? There are a couple of supported compression algorithms: gzip (used below), compress , deflate , br . Learn more: https://developer.mozilla.org/en-US/docs/Web/HTTP/Headers/Content-Encoding If you don't add the content-encoding header, the gzip result looks like: ���������
�� �.���� �����������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������YDA��"�� Which is incredibly short for a 500kb string! (Which shouldn't be surprising because it's just "hi" 250k times)
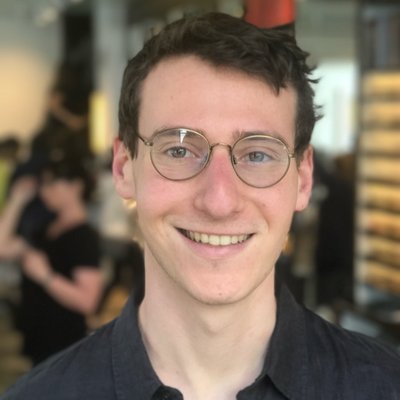
compress_response
@stevekrouse
HTTP
Compress Response Isn't it cool that browsers can natively decompress stuff? There are a couple of supported compression algorithms: gzip (used below), compress , deflate , br . Learn more: https://developer.mozilla.org/en-US/docs/Web/HTTP/Headers/Content-Encoding If you don't add the content-encoding header, the gzip result looks like: ���������
�� �.���� �����������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������������YDA��"�� Which is incredibly short for a 500kb string! (Which shouldn't be surprising because it's just "hi" 250k times)