Back to APIs list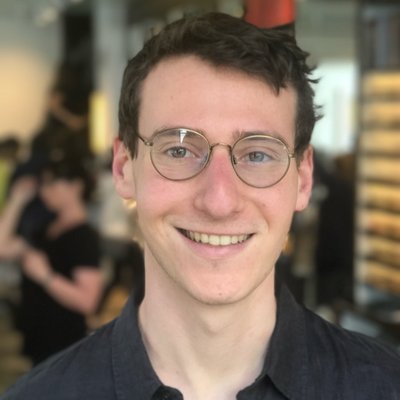
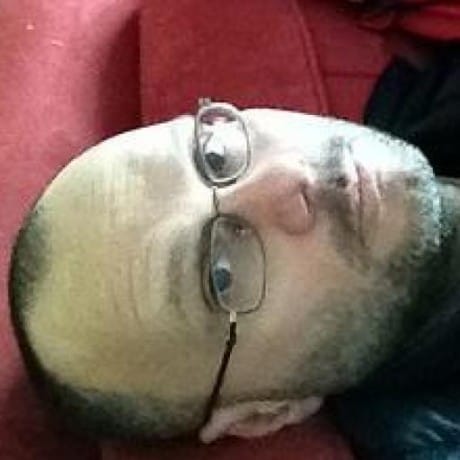
Slack API examples & templates
Use these vals as a playground to view and fork Slack API examples and templates on Val Town. Run any example below or find templates that can be used as a pre-built solution.
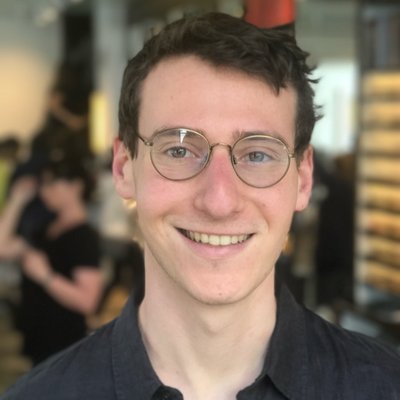
slack_cleaner
@stevekrouse
HTTP
Paste messages from Slack, get clean markdown This little webapp is intended to make it easier to copy messages from Slack into other places for sharing or archival, with cleaned-up, readable formatting. I hacked it together on valtown with copious help from an LLM over a weekend; please propose changes or edits, as I'm sure I missed many use-cases and edge-cases. This app runs entirely in your browser and does not send your data anywhere.
reply_to_slack_message
@curtcox
HTTP
This val provides a way of readily having lots of different Slack bots that do different things and support different Slack workspaces without needing a bunch of Val Town accounts. If you only need a single bot on a single workspace, just go with the approach in the Val Town docs . This bot is essentially the one described in the Val Town docs , but without any of the details. Those are externalized by the SlackConfig and SlackFunction interfaces. It is just glue. In order to make it work, you will need the following additional glue: a public HTTP val to handle requests like this one a private val to supply any info missing from the public one like this one Actually, those could both be public or all in the same val, but the whole point of this scheme is to allow you to hide anything you want.