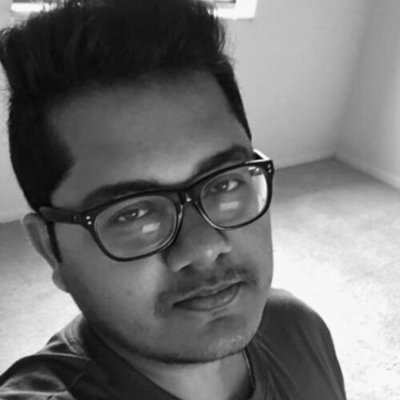
ramkarthik
Software engineer. He/Him. ManUtd fan. I build side projects (http://kramkarthik.com/projects). Recent project - https://dotnetjobs.co/.
Joined May 26, 2023
Public vals
12
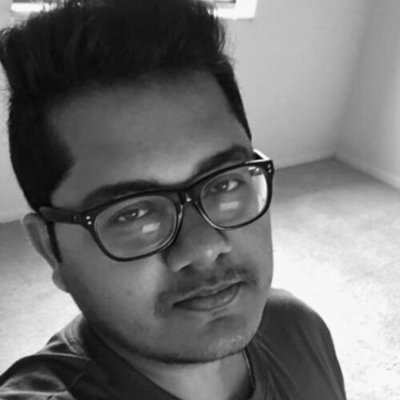
bookmark
@ramkarthik
HTTP
A minimal bookmarking tool This allows you to bookmark links and view them later. Completely powered by ValTown and SQLite. To set this up for yourself Fork the val From your ValTown settings page, add an environment variable named bookmarks_client_id and give it a value (you will be using this for saving) Add another environment variable named bookmarks_client_secret and give it a value (you will also be using this for saving) At first, the "bookmarks" table will not exist, so we need to save an article first, which will create the "bookmarks" table To do this, add a bookmarklet to your browser with this value (replace BOOKMARKS-CLIENT-ID and BOOKMARKS-CLIENT-SECRET with the values you added to the environment variables, and replace BOOKMARKS-URL with your VAL's URL): javascript:void(open('BOOKMARKS-URL/save?u='+encodeURIComponent(location.href)+'&t='+encodeURIComponent(document.title)+'&id=BOOKMARKS-CLIENT-ID&secret=BOOKMARKS-CLIENT-SECRET', 'Bookmark a link', 'width=400,height=450')) Click this bookmarklet to bookmark the URL of the current active tab Go to your VAL URL homepage to see the bookmark Demo Here are my bookmarks:
https://ramkarthik-bookmark.web.val.run/ Note Make sure you don't share bookmarks_client_id and bookmarks_client_secret . It is used for authentication before saving a bookmark.