Public vals
8
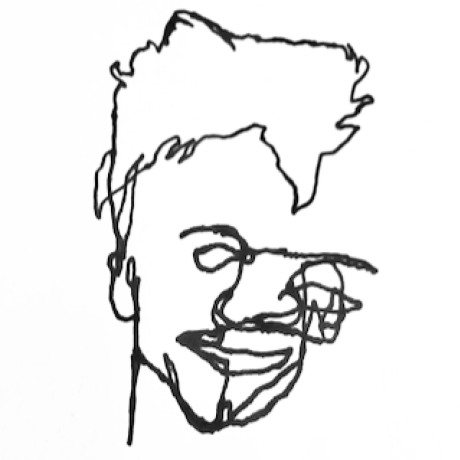
sqliteExplorerApp
@parkerdavis
HTTP
SQLite Explorer View and interact with your Val Town SQLite data. It's based off Steve's excellent SQLite Admin val, adding the ability to run SQLite queries directly in the interface. This new version has a revised UI and that's heavily inspired by LibSQL Studio by invisal . This is now more an SPA, with tables, queries and results showing up on the same page. Install Install the latest stable version (v66) by forking this val: Authentication Login to your SQLite Explorer with password authentication with your Val Town API Token as the password. Todos / Plans [ ] improve error handling [ ] improve table formatting [ ] sticky table headers [ ] add codemirror [ ] add loading indication to the run button (initial version shipped) [ ] add ability to favorite queries [ ] add saving of last query run for a table (started) [ ] add visible output for non-query statements [ ] add schema viewing [ ] add refresh to table list sidebar after CREATE/DROP/ALTER statements [ ] add automatic execution of initial select query on double click [ ] add views to the sidebar [ ] add triggers to sidebar [ ] add upload from SQL, CSV and JSON [ ] add ability to connect to a non-val town Turso database [x] fix wonky sidebar separator height problem (thanks to @stevekrouse) [x] make result tables scrollable [x] add export to CSV, and JSON (CSV and JSON helper functions written in this val . Thanks to @pomdtr for merging the initial version!) [x] add listener for cmd+enter to submit query
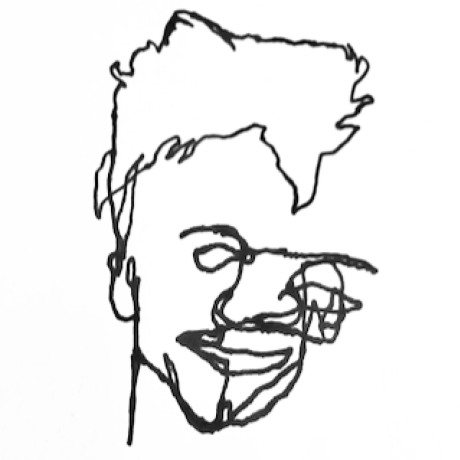
password_auth
@parkerdavis
Script
Password Auth Middleware Protect your vals behind a password. Use session cookies to persist authentication. Demo See @pomdtr/password_auth_test Usage If you want to use an api token to authenticate: import { passwordAuth } from "https://esm.town/v/pomdtr/password_auth";
export default passwordAuth(() => {
return new Response("OK");
}); Or if you prefer to use a string: import { passwordAuth } from "https://esm.town/v/pomdtr/password_auth";
export default passwordAuth(() => {
return new Response("OK");
}, { password: Deno.env.get("VAL_PASSWORD") }); You can also set multiple ones import { passwordAuth } from "https://esm.town/v/pomdtr/password_auth";
export default passwordAuth(() => {
return new Response("OK");
}, { password: [Deno.env.get("VAL_PASSWORD"), Deno.env.get("STEVE_PASSWORD")] }); Note that authenticating using your api token remain an option even after setting a password. TODO [x] allow to authenticate using a val town token [ ] add a way to send an email to ask a password from the val owner [ ] automatically extend the session [ ] automatically remove expired sessions FAQ How to sign out ? Navigate to <your-site>/signout .
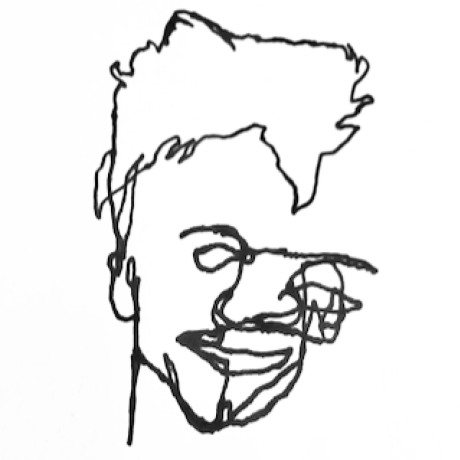
comments
@parkerdavis
HTTP
Comments (just add water) A self-contained comments system Val. Just fork this val and you have a complete (but extremely minimal) comment system! Call on the front-end using: const MY_FORKED_VAL_URL = "https://vez-comments.web.val.run";
const getComments = async () => {
const response = await fetch(MY_FORKED_VAL_URL);
const json = await response.json();
return json;
};
const addComment = async (str) => {
try {
const response = await fetch(MY_FORKED_VAL_URL, {
method: "POST",
body: JSON.stringify(str),
});
if (response.status >= 400 && response.status < 600) {
/* error */
return false;
} else {
/* success */
return true;
}
} catch (e) {
/* error */
return false;
}
};
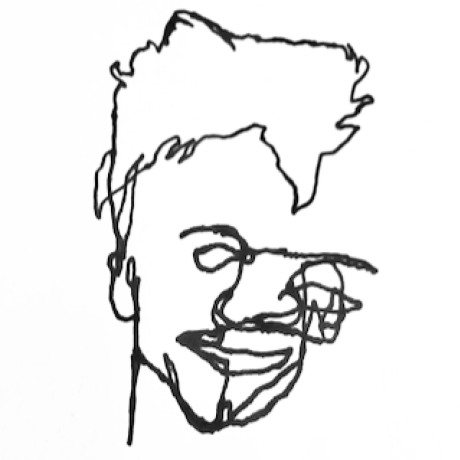
blob_admin
@parkerdavis
HTTP
Blob Admin This is a lightweight Blob Admin interface to view and debug your Blob data. Use this button to install the val: It uses basic authentication with your Val Town API Token as the password (leave the username field blank). TODO [x] /new - render a page to write a new blob key and value [x] /edit/:blob - render a page to edit a blob (prefilled with the existing content) [x] /delete/:blob - delete a blob and render success [ ] handle non-textual val properly [ ] add upload/download buttons [ ] merge edit and view pages [ ] add client side navigation using htmx [ ] use codemirror instead of a textarea for editing text blobs
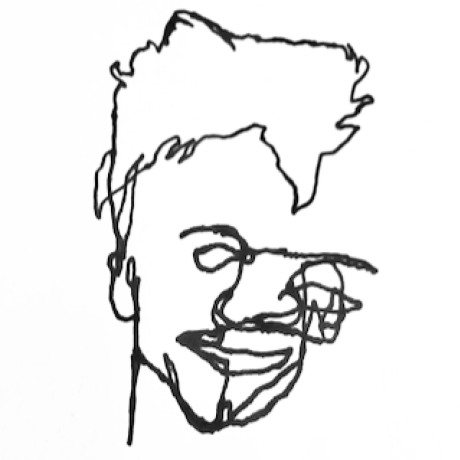
docFeedbackForm
@parkerdavis
HTTP
Val Town Docs Feedback Form & Handler Live form Val Town Docs YouTube tutorial that explains v17 of this val This feedback form is linked on our docs site. This val renders an HTML form, including pre-fills the user's email address if they've submitted the form in the past (via a cookie), and pre-fills the URL by grabbing it out of the query params. It handles form submissions, including parsing the form, saving the data into @stevekrouse.docsFeedback , a private JSON val, and then returns a thank you message, and set's the user's email address as a cookie, to save them some keystrokes the next time they fill out the form. Another val, @stevekrouse.formFeedbackAlert , polls on an interval for new form submissions, and if it finds any, forwards them on a private Val Town discord channel. There are a number of subtleties to the way each of some features are implemented. A user submitted three pieces of feedback in quick succession, so I thought it'd be nice if we remembered user's email addresses after their first form submissions. There are classically two ways to do this, cookies or localstorage. I choose cookies. It requires setting them in the response header and getting them out of the request header. I used a Deno library to parse the cookie but I set it manually because that seemed simpler. You may be wondering about how I'm getting the referrer out of the query params instead of from the HTTP Referrer header. I tried that at first, but it's increasingly difficult to get path data from it due to more restrictive security policies . So instead I decided to include the URL data in a query param. I get it there via this script in my blog's site: function updateFeedback(ref) {
let feedback = [...document.getElementsByTagName('a')].find(e => e.innerText == 'Feedback')
feedback.setAttribute('href', "https://stevekrouse-docfeedbackform.web.val.run/?ref=" + ref)
}
setTimeout(() => updateFeedback(document.location.href), 100);
navigation.addEventListener('navigate', e => updateFeedback(e.destination.url)); Finally, you may be wondering why I queue up feedback in @stevekrouse.docsFeedback , a private JSON val, and then process it via @stevekrouse.formFeedbackAlert instead of sending it along to Discord directly in this val. I tried that originally but it felt too slow to wait for the API call to Discord before returning the "Thanks for your feedback" message. This is where the context.waitUntil method (that Cloudflare workers and Vercel Edge Functions support) would really come in handy – those allow you to return a Response, and then continue to compute. Currently Val Town requires you to stop all compute with the returning of your Response, so the only way to compute afterwards is to queue it up for another val to take over, and that's what I'm doing here.