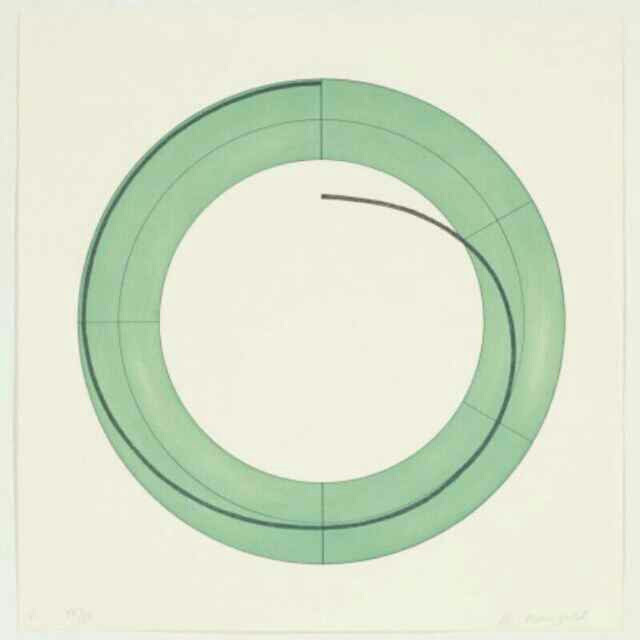
一个计算“QQ等级”的函数,用来自动更新签名还挺好玩的。
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
export function calc_qq_string(grade: number) {
const rest = grade.toString(4).split("").map(Number);
const [
star,
moon,
sun,
crown,
diamond,
heart,
fire,
rocket,
popper,
hundred,
] = rest.reverse();
// some of the emojies were picked by ChatGPT.
return `${"💯".repeat(hundred)}${"🎉".repeat(popper)}${"🚀".repeat(rocket)}${
"🔥".repeat(fire)
}${"❤️".repeat(heart)}${"💎".repeat(diamond)}${"👑".repeat(crown)}${
"🌞".repeat(sun)
}${"🌙".repeat(moon)}${"⭐".repeat(star)}`;
}
把 GitHub stars 数量转换为 QQ 等级 SVG 图片 Convert GitHub stars into SVG of QQ level
QQ is a popular icq alternative from China
用法 Usage
https://envl-qq_svg.web.val.run/:user/:repo?font_size=24&px=4&py=3
repo
选填 Optionalpx
选填 Optionalpy
选填 Optionalfont_size
选填 Optional
举例 Example:
https://envl-qq_svg.web.val.run/envl
https://envl-qq_svg.web.val.run/freecodecamp/freecodecamp
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
import { fetch } from "https://esm.town/v/std/fetch";
import { calc_qq_string } from "https://esm.town/v/envl/calc_qq_string";
export async function qq_svg(req: Request) {
const url = new URL(req.url);
const [user, repo] = url.pathname.substring(1).split("/");
const px = url.searchParams.get("px")
? Number(url.searchParams.get("px"))
: 4;
const py = url.searchParams.get("py")
? Number(url.searchParams.get("py"))
: 3;
const font_size = url.searchParams.get("font_size")
? Number(url.searchParams.get("font_size"))
: 14;
let stars = 0;
// stars for user
if (!repo) {
stars = await fetch(`https://api.github.com/users/${user}/repos`)
.then((r) => r.json())
.then((d) =>
d.reduce?.((count, repo) => count + repo.stargazers_count, 0)
);
}
// stars for repo
else {
const res = await fetch(
`https://api.github.com/search/repositories?q=${user}/${repo}`,
);
const data = await res.json();
stars = data.items[0].stargazers_count;
}
const qq_str = calc_qq_string(stars);
const qq_str_char_length = stars.toString(4).split("").reduce(
(acc, n_str) => Number(n_str) + acc,
0,
);
const svg_width = px * 2 + qq_str_char_length * (py * 2 + font_size);
const svg = `<svg width="${svg_width}" height="${
font_size + px * 2
}" viewBox="0 0 ${svg_width} ${
font_size + px * 2
}" xmlns="http://www.w3.org/2000/svg">
<text fill="black" x="4" y="${font_size}" font-size="${font_size}">${qq_str}</text>
</svg>`;
return new Response(svg, {
headers: {
"Content-Type": "image/svg+xml;charset=utf-8",
},
});
}
1
2
3
4
5
6
7
8
9
import { calc_qq_string } from "https://esm.town/v/envl/calc_qq_string";
export async function upgradeQQ(initial = 1688027887700) {
const unit = 1000 * 3600 * 12; // 这里取12小时做一个单位
// const initial = 1688027887700;
const elapsed = Date.now() - initial;
const grade = Math.ceil(elapsed / unit); // 一个时间单位升一级
return calc_qq_string(grade);
}
send me an instant message
GET https://envl-sendMsg.web.val.run/?msg=hi
- or
POST
tohttps://envl-sendMsg.web.val.run
withmsg
in JSON format
1
2
3
4
5
6
7
import { im } from "https://esm.town/v/envl/im";
export async function sendMsg(req: Request) {
const msg = new URL(req.url).searchParams.get("msg") ?? (await req.json()).msg;
await im(msg);
return new Response(`sent message: ${msg}`);
}
1
2
3
4
5
import { siteDB } from "https://esm.town/v/envl/siteDB";
export async function getProjects() {
return Response.json((await siteDB).projList);
}