Search
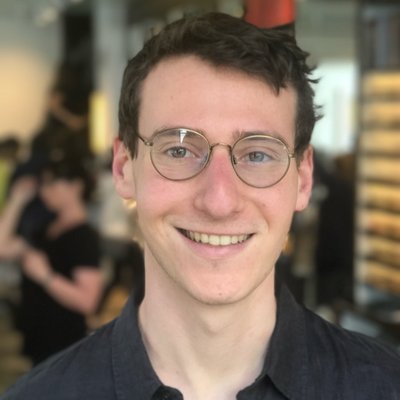
plotLinkeDOMSRRExample
@stevekrouse
An interactive, runnable TypeScript val by stevekrouse
HTTP
import * as Plot from "npm:@observablehq/plot@0.6.14";
import { d3 } from "npm:d3@7";
import { parseHTML } from "npm:linkedom@0.15";
export default async function() {
const document = parseHTML("<a>").document;
TanStackBlogToRSS
@vogelino
TanStack Blog to RSS Handy microservice/library to convert the TanStack Blog into an RSS Feed. Froked from curtcox/markdown_dowload.
The idea is to generate an RSS feed from a blog or page that doesn't provide its own. I takes the logic of the forked val, which is to convert any URL to markdown, and places it into an RSS feed for easy subscription in feed.ly. 👇 --- ORIGINAL README -- 👇 Introductory blog post: https://taras.glek.net/post/markdown.download/ Package: https://jsr.io/@tarasglek/markdown-download Features Apply readability Further convert article into markdown to simplify it Allow webpages to be viewable as markdown via curl Serve markdown converted to html to browsers Extract youtube subtitles Source https://github.com/tarasglek/markdown-download https://www.val.town/v/taras/markdown_download License: MIT Usage:
https://markdown.download/ + URL Dev:
https://val.markdown.download/ + URL
HTTP
import buildRSSFeedString from "https://esm.town/v/vogelino/buildRSSFeedString";
import { DOMParser } from "npm:linkedom@0.16.10";
function response(message: string, contentType = "text/markdown"): Response {
const headers = new Headers();
findPlates
@adnan
// URL of your RSS feed
Cron
import { DOMParser } from "https://esm.sh/linkedom@0.16.1";
import { blob } from "https://esm.town/v/std/blob";
// URL of your RSS feed
scrape2md
@taras
This is a deno/valtown port in progress of https://github.com/tarasglek/scrape2md License: MIT Handy script to scrape various data sources into markdown. Intended to feed llms in https://chatcraft.org Usage:
https://taras-scrape2md.web.val.run/ + URL_TO_SCRAPE Or just visit in browser and paste your url TODO https://chatcraft.org/api/share/tarasglek/IDYChVAilfePgVZb_T5pH POST from browser
https://www.val.town/v/nbbaier/valToGH sync to github Metadata for use with https://github.com/tarasglek/valtown2js: {
"typeCheck": false,
"mappings": {
"https://esm.sh/linkedom": {
"name": "linkedom",
"version": "^0.16.8"
}
},
"package": {
"name": "scrape2md",
"version": "1.0.0",
"devDependencies": {
"@types/turndown": "^5.0.4"
}
}
}
HTTP
"mappings": {
"https://esm.sh/linkedom": {
"name": "linkedom",
"version": "^0.16.8"
import { isProbablyReaderable, Readability } from "npm:@mozilla/readability@^0.5.0";
import { DOMParser } from "npm:linkedom@0.16.10";
import { marked } from "npm:marked@12.0.1";
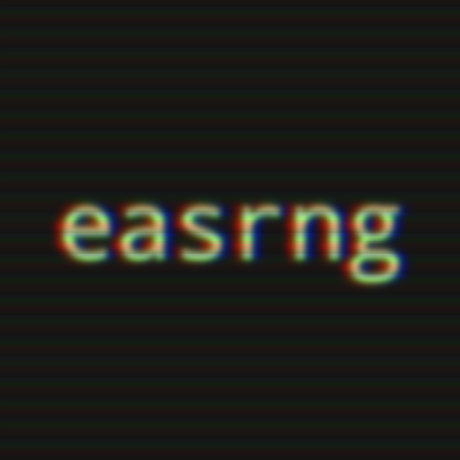
rssViewer
@easrng
rss viewer
a basic RSS reader
HTTP
import { type Component } from "npm:@easrng/elements@0.1.15";
import { stream } from "npm:@easrng/elements@0.1.15/server";
import { parseHTML } from "npm:linkedom@0.18.4";
const { document, Node } = parseHTML(`<!DOCTYPE html>`);
import { cohostSanitize } from "https://esm.town/v/easrng/cohostSanitize";
markdown_download
@curtcox
markdown.download Handy microservice/library to convert various data sources into markdown. Intended to make it easier to consume the web in ereaders Introductory blog post: https://taras.glek.net/post/markdown.download/ Package: https://jsr.io/@tarasglek/markdown-download Features Apply readability Further convert article into markdown to simplify it Allow webpages to be viewable as markdown via curl Serve markdown converted to html to browsers Extract youtube subtitles Source https://github.com/tarasglek/markdown-download https://www.val.town/v/taras/markdown_download License: MIT Usage:
https://markdown.download/ + URL Dev:
https://val.markdown.download/ + URL
HTTP
import { isProbablyReaderable, Readability } from "npm:@mozilla/readability@^0.5.0";
import { DOMParser } from "npm:linkedom@0.16.10";
import { marked } from "npm:marked@12.0.1";
import { getSubtitles } from "npm:youtube-captions-scraper@^2.0.1";
youthfulCyanSheep
@alexwein
Find a Big Word - Bogglelike This endpoint displays an randomly generated SVG of a 4x4 grid of letters.
The Grid is guaranteed to have word that uses every letter using standard Boggle-style word-finding rules. Thanks to Fil for the example using Observable Plot. | | |
|-----|-----|
| Web page | https://alexwein-fabwbogglelike.web.val.run |
| Observable Plot for image | https://observablehq.com/plot/ |
| wordnik from words | https://github.com/wordnik/wordlist |
HTTP
const Plot = await import("https://cdn.jsdelivr.net/npm/@observablehq/plot@0.6.14/+esm");
// Create a mock document object to prevent undefined errors
const { document } = await import("https://cdn.jsdelivr.net/npm/linkedom@0.16.1/+esm").then((m) =>
m.parseHTML(`<html><body></body></html>`)
// Ensure global is defined for server-side rendering

extractSchemaOrgJson
@aeaton
An interactive, runnable TypeScript val by aeaton
HTTP
import { DOMParser } from "https://esm.sh/linkedom";
export default async function server(request: Request): Promise<Response> {
if (request.method !== "GET") {
AtelierHarfangToRSS
@vogelino
Atelier Harfang to RSS Handy microservice/library to convert the projects of Atelier Harfang into an RSS Feed. Froked from curtcox/markdown_dowload.
The idea is to generate an RSS feed from a blog or page that doesn't provide its own. I takes the logic of the forked val, which is to convert any URL to markdown, and places it into an RSS feed for easy subscription in feed.ly. 👇 --- ORIGINAL README -- 👇 Introductory blog post: https://taras.glek.net/post/markdown.download/ Package: https://jsr.io/@tarasglek/markdown-download Features Apply readability Further convert article into markdown to simplify it Allow webpages to be viewable as markdown via curl Serve markdown converted to html to browsers Extract youtube subtitles Source https://github.com/tarasglek/markdown-download https://www.val.town/v/taras/markdown_download License: MIT Usage:
https://markdown.download/ + URL Dev:
https://val.markdown.download/ + URL
HTTP
import { altelierHarfanfLastItems } from "https://esm.town/v/vogelino/altelierHarfanfLastItems";
import buildRSSFeedString from "https://esm.town/v/vogelino/buildRSSFeedString";
import { DOMParser } from "npm:linkedom@0.16.10";
function response(message: string, contentType = "text/markdown"): Response {
const headers = new Headers();
semanticSearchBlogPostPlot
@janpaul123
An interactive, runnable TypeScript val by janpaul123
HTTP
const Plot = await import("https://cdn.jsdelivr.net/npm/@observablehq/plot@0.6.14/+esm");
const d3 = await import("https://cdn.jsdelivr.net/npm/d3@7/+esm");
const { document } = await import("https://cdn.jsdelivr.net/npm/linkedom@0.15/+esm").then((
{ parseHTML: p },
) => p(`<a>`));
fabwbogglelike
@alexwein
Find a Big Word - Bogglelike This endpoint displays an randomly generated SVG of a 4x4 grid of letters.
The Grid is guaranteed to have word that uses every letter using standard Boggle-style word-finding rules. Thanks to Fil for the example using Observable Plot. | | |
|-----|-----|
| Web page | https://alexwein-fabwbogglelike.web.val.run |
| Observable Plot for image | https://observablehq.com/plot/ |
| wordnik from words | https://github.com/wordnik/wordlist |
HTTP
const Plot = await import("https://cdn.jsdelivr.net/npm/@observablehq/plot@0.6.14/+esm");
// Create a mock document object to prevent undefined errors
const { document } = await import("https://cdn.jsdelivr.net/npm/linkedom@0.16.1/+esm").then((m) =>
m.parseHTML(`<html><body></body></html>`)
// Ensure global is defined for server-side rendering
markdown_download
@taras
markdown.download Handy microservice/library to convert various data sources into markdown. Intended to make it easier to consume the web in ereaders Introductory blog post: https://taras.glek.net/post/markdown.download/ Package: https://jsr.io/@tarasglek/markdown-download Features Apply readability Further convert article into markdown to simplify it Allow webpages to be viewable as markdown via curl Serve markdown converted to html to browsers Extract youtube subtitles Can paste htmlContent in curl 'https://val.markdown.download/' -H 'content-type: application/x-www-form-urlencoded' --data-urlencode "htmlContent@$HOME/Downloads/oai-reference.html" Source https://github.com/tarasglek/markdown-download https://www.val.town/v/taras/markdown_download License: MIT Usage:
https://markdown.download/ + URL Dev:
https://val.markdown.download/ + URL
HTTP
import { isProbablyReaderable, Readability } from "npm:@mozilla/readability@^0.5.0";
import { DOMParser } from "npm:linkedom@0.16.10";
import { marked } from "npm:marked@12.0.1";
import { getSubtitles } from "npm:youtube-captions-scraper@^2.0.1";
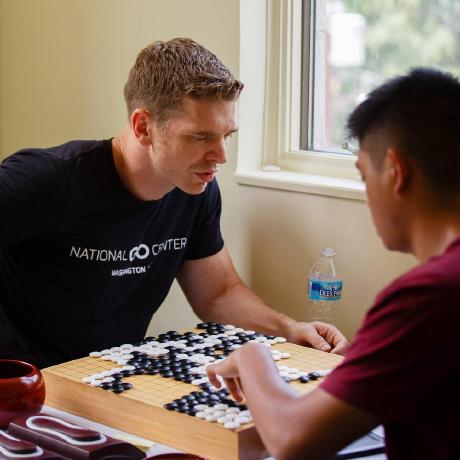
webscrapeBareBonesTiki
@hurricanenate
Check Bare Bones Tiki to see when their sea light swizzles become available.
Cron
import { DOMParser, Node } from "https://esm.sh/linkedom@0.16.1";
import { email } from "https://esm.town/v/std/email?v=9";
import { fetchText } from "https://esm.town/v/stevekrouse/fetchText?v=5";
musicalPeachCatfish
@alexwein
Find a Big Word - Bogglelike This endpoint displays an randomly generated SVG of a 4x4 grid of letters.
The Grid is guaranteed to have word that uses every letter using standard Boggle-style word-finding rules. Thanks to Fil for the example using Observable Plot. | | |
|-----|-----|
| Web page | https://alexwein-fabwbogglelike.web.val.run |
| Observable Plot for image | https://observablehq.com/plot/ |
| wordnik from words | https://github.com/wordnik/wordlist |
HTTP
const Plot = await import("https://cdn.jsdelivr.net/npm/@observablehq/plot@0.6.14/+esm");
// Create a mock document object to prevent undefined errors
const { document } = await import("https://cdn.jsdelivr.net/npm/linkedom@0.16.1/+esm").then((m) =>
m.parseHTML(`<html><body></body></html>`)
// Ensure global is defined for server-side rendering
AriaSnapshotFromUrl
@fgeierst
compare https://github.com/microsoft/playwright/blob/main/packages/playwright-core/src/server/injected/ariaSnapshot.ts
HTTP
import { parseHTML } from 'https://esm.sh/linkedom@0.16.0';
export default async function server(request: Request): Promise<Response> {
// Parse the URL from the request query parameters