Back to packages list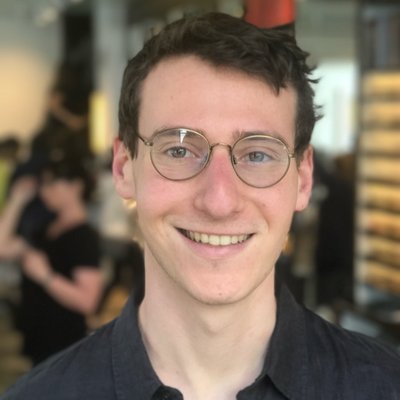
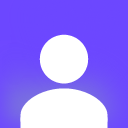
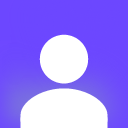
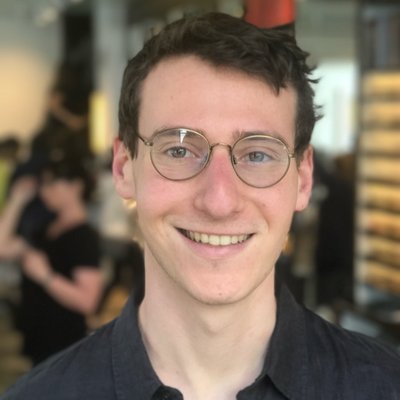
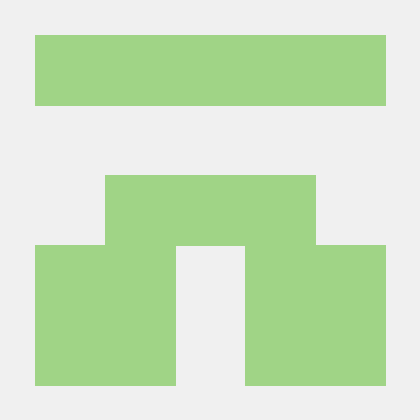
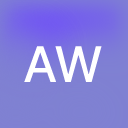
Vals using openai
Description from the NPM package:
The official TypeScript library for the OpenAI API
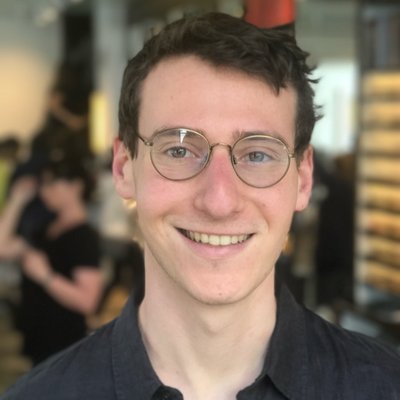
upgradeHTTPPreviewVals
@stevekrouse
Script
Auto-Upgrade for HTTP Preview This val is experimentally testing if we can use an LLM to determine if an old-style HTTP val
needs to be upgraded for the new HTTP runtime, currently in preview. You can read more about the breaking change and upgrade proccess here: https://blog.val.town/blog/http-preview/#breaking-changes In some light testing, it seems like ChatGPT 3.5 and 4o both are bad at this task,
so I'm pausing experimenting with this for now. Example output from 4o: [
{
"name": "harlequinChickadee",
"probabilityUpgradeNeeded": true,
"reason": "The current code structure has several functions and program logic outside the main handler, including word selection, game state management, and SVG generation. These parts would not re-run with the new runtime, potentially affecting functionality. They need to be moved inside the handler to ensure consistent behavior across requests."
},
{
"name": "redElephant",
"probabilityUpgradeNeeded": "100%",
"reason": "The initialization of `fs` and `vscode` objects should occur \n inside the handler in the new runtime in order to ensure that they are \n freshly created for each request. This is critical since the new runtime \n does not rerun code outside of the handler for each request.."
},
{
"name": "untitled_indigoNightingale",
"probabilityUpgradeNeeded": false,
"reason": "The code initializes and configures the Hono app outside of the handler, but it does not appear to have any stateful logic that would need to be re-calculated with each request. Each request will call the handler provided by Hono and should behave the same in the new runtime."
},
{
"name": "untitled_pinkRoundworm",
"probabilityUpgradeNeeded": true,
"reason": "The functions `addComment` and `getComments` as well as the initialization \nof the KEY variable perform actions that are intended to be run per request. These need to be moved \ninside the relevant HTTP handler to ensure the behavior remains consistent in the new runtime."
},
{
"name": "untitled_harlequinIguana",
"probabilityUpgradeNeeded": false,
"reason": "The provided code defines the handler directly without any side effects or additional code outside the handler. The behavior should remain the same in the new runtime."
},
{
"name": "untitled_moccasinHeron",
"probabilityUpgradeNeeded": false,
"reason": "The code outside the handler is just a constant string declaration, which does not change behavior between requests. The handler itself handles requests correctly and independently."
},
{
"name": "untitled_maroonSwallow",
"probabilityUpgradeNeeded": false,
"reason": "All the code, including the check for authentication,\n is inside the handler function. This means the behavior will stay \n the same with the new runtime."
},
{
"name": "wikiOG",
"probabilityUpgradeNeeded": true,
"reason": "The function `getWikipediaInfo` defined outside of the handler makes network requests and processes data for each request. In the new runtime, this function would only be executed once and cached. To ensure the same behavior in the new runtime, this function should be moved into the handler."
},
{
"name": "parsePostBodyExample",
"probabilityUpgradeNeeded": false,
"reason": "All the code is inside the handler, so the behavior will remain consistent in the new runtime."
},
{
"name": "discordEventReceiver",
"probabilityUpgradeNeeded": false,
"reason": "All the relevant code for handling requests and logging input is inside the handler.\n No code needs to be moved for the new runtime to function correctly."
}
] Feel free to fork this and try it yourself!
If you could get it working, it'd be a big help for us as we upgrade the thousands of HTTP vals. Future ideas: Better LLM (Claude 3.5) Better prompt More examples JSON mode having it reply with upgraded code send pull requests to users with public vals that probably need upgrading
ReadmeWriter
@willthereader
Script
Val Town AI Readme Writer This val provides a class ReadmeWriter for generating readmes for vals with OpenAI. It can both draft readmes and update them directly PRs welcome! See Todos below for some ideas I have. Usage To draft a readme for a given code, use the draftReadme method: import { ReadmeWriter } from "https://esm.town/v/nbbaier/readmeGPT";
const readmeWriter = new ReadmeWriter({});
const val = "https://www.val.town/v/:username/:valname";
const generatedReadme = await readmeWriter.draftReadme(val); To write and update a readme for a given code, use the writeReadme method: import { ReadmeWriter } from "https://esm.town/v/nbbaier/readmeGPT";
const readmeWriter = new ReadmeWriter({});
const val = "https://www.val.town/v/:username/:valname";
const successMessage = await readmeWriter.writeReadme(val); API Reference Class: ReadmeWriter The ReadmeWriter class represents a utility for generating and updating README files. Constructor Creates an instance of the ReadmeWriter class. Parameters: model (optional): The model to be used for generating the readme. Defaults to "gpt-3.5-turbo". apiKey (optional): An OpenAI API key. Defaults to Deno.env.get("OPENAI_API_KEY") . Methods draftReadme(val: string): Promise<string> : Generates a readme for the given val. Parameters: val : URL of the code repository. Returns: A promise that resolves to the generated readme. writeReadme(val: string): Promise<string> : Generates and updates a readme for the given val. Parameters: val : URL of the code repository. Returns: A promise that resolves to a success message if the update is successful. Todos [ ] Additional options to pass to the OpenAI model [ ] Ability to pass more instructions to the prompt to modify how the readme is constructed
OpenAI
@wangqiao1234
Script
OpenAI - Docs ↗ Use OpenAI's chat completion API with std/openai . This integration enables access to OpenAI's language models without needing to acquire API keys. For free Val Town users, all calls are sent to gpt-3.5-turbo . Streaming is not yet supported. Upvote the HTTP response streaming feature request if you need it! Usage import { OpenAI } from "https://esm.town/v/std/openai";
const openai = new OpenAI();
const completion = await openai.chat.completions.create({
messages: [
{ role: "user", content: "Say hello in a creative way" },
],
model: "gpt-4",
max_tokens: 30,
});
console.log(completion.choices[0].message.content); Limits While our wrapper simplifies the integration of OpenAI, there are a few limitations to keep in mind: Usage Quota : We limit each user to 10 requests per minute. Features : Chat completions is the only endpoint available. If these limits are too low, let us know! You can also get around the limitation by using your own keys: Create your own API key on OpenAI's website Create an environment variable named OPENAI_API_KEY Use the OpenAI client from npm:openai : import { OpenAI } from "npm:openai";
const openai = new OpenAI(); 📝 Edit docs
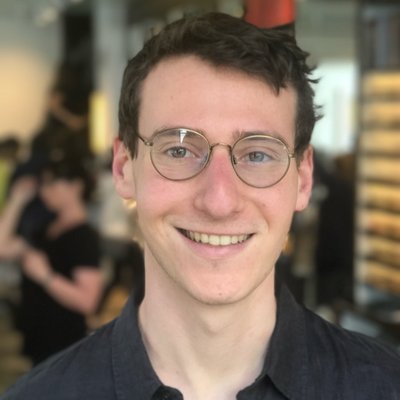
webgen
@stevekrouse
HTTP
Made on Val Town livestream. This project is a kind of a mini tribute to Websim . To-dos: Spruce up styles a bit Write this README ~Add a cache!~ ~Try moving style tag to the bottom by prompting so content appears immediately and then becomes styled~ didn't work b/c CSS parsing isn't progressive Need more prompting to get the model not to generate placeholder-y content Better root URL page / index page with links to some good sample generations
openAIStreaming
@xuybin
HTTP
OpenAI Streaming - Assistant and Threads An example of using OpenAI to stream back a chat with an assistant. This example sends two messages to the assistant and streams back the responses when they come in. Example response: user > What should I build today?
................
assistant > Here are a few fun Val ideas you could build on Val Town:
1. **Random Joke Generator:** Fetch a random joke from an API and display it.
2. **Daily Weather Update:** Pull weather data for your location using an API and create a daily summary.
3. **Mini Todo List:** Create a simple to-do list app with add, edit, and delete functionalities.
4. **Chuck Norris Facts:** Display a random Chuck Norris fact sourced from an API.
5. **Motivational Quote of the Day:** Fetch and display a random motivational quote each day.
Which one sounds interesting to you?
user > Cool idea, can you make it even cooler?
...................
assistant > Sure, let's add some extra flair to make it even cooler!
How about creating a **Motivational Quote of the Day** app with these features:
1. **Random Color Theme:** Each day, the background color/theme changes randomly.
2. **Quote Sharing:** Add an option to share the quote on social media.
3. **Daily Notifications:** Send a daily notification with the quote of the day.
4. **User Preferences:** Allow users to choose categories (e.g., success, happiness, perseverance) for the quotes they receive.
Would you like some code snippets or guidance on implementing any of these features?
webgen
@triptych
HTTP
To-dos: Spruce up styles a bit Write this README ~Add a cache!~ ~Try moving style tag to the bottom by prompting so content appears immediately and then becomes styled~ didn't work b/c CSS parsing isn't progressive Need more prompting to get the model not to generate placeholder-y content Better root URL page / index page with links to some good sample generations