Back to packages list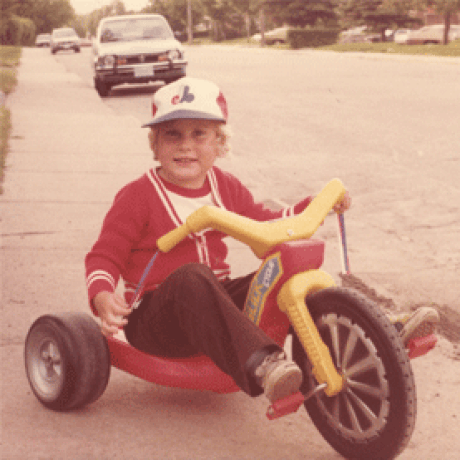
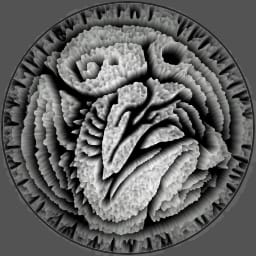
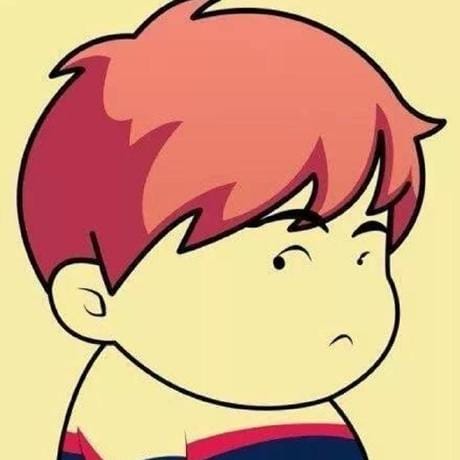
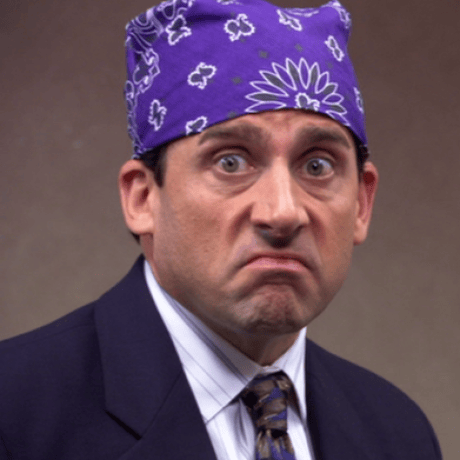
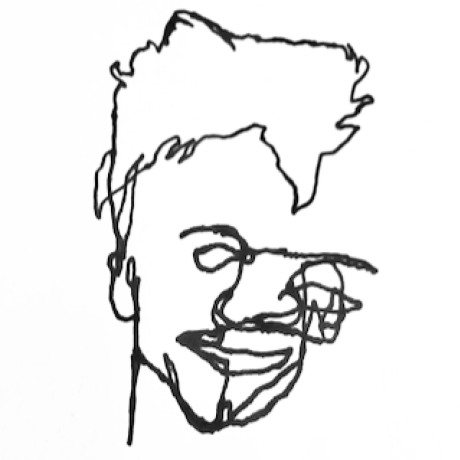
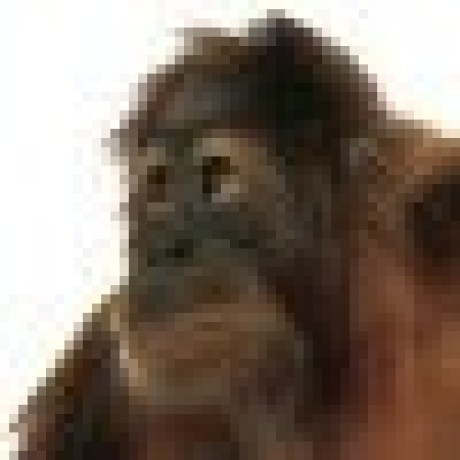
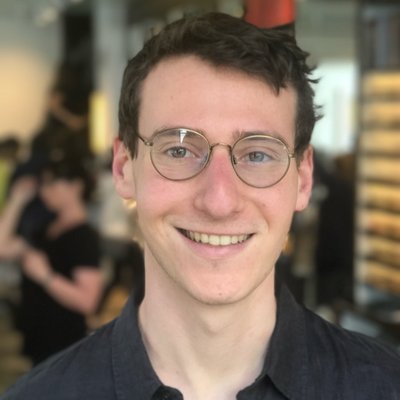
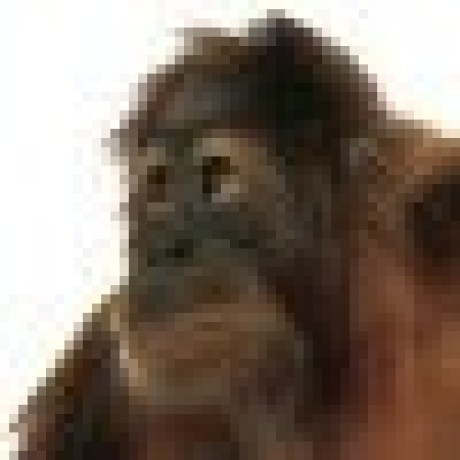
Vals using lodash-es
Description from the NPM package:
Lodash exported as ES modules.
getSpotifyTrackUrl
@dthyresson
Script
getSpotifyTrackUrl Get a Spotify Track Url using the Spotify Web API given an artist and a song title. Track info is cached by the query and also the spotify track id, so your popular queries won't have to fetch from Spotify over and over. Examples import { getSpotifyTrackUrl } from "https://esm.town/v/dthyresson/getSpotifyTrackUrl";
const reni = await getSpotifyTrackUrl("Stone Roses", "Fools Gold");
const ian = await getSpotifyTrackUrl("Joy Division", "Love Will Tear Us Apart");
const kim = await getSpotifyTrackUrl("Pixies", "Velouria");
console.log(reni)
console.log(ian)
console.log(kim) Info Uses getSpotifyAccessToken which requires you to set environment variables from your Spotify Developers account. SPOTIFY_CLIENT_ID
SPOTIFY_CLIENT_SECRET Your access token is cached by getSpotifyAccessToken to avoid fetching over and over.
password_auth
@kamek
Script
Password Auth Middleware Protect your vals behind a password. Use session cookies to persist authentication. Demo See @pomdtr/password_auth_test Usage If you want to use an api token to authenticate: import { passwordAuth } from "https://esm.town/v/pomdtr/password_auth";
export default passwordAuth(() => {
return new Response("OK");
}); Or if you prefer to use a string: import { passwordAuth } from "https://esm.town/v/pomdtr/password_auth";
export default passwordAuth(() => {
return new Response("OK");
}, { password: Deno.env.get("VAL_PASSWORD") }); You can also set multiple ones import { passwordAuth } from "https://esm.town/v/pomdtr/password_auth";
export default passwordAuth(() => {
return new Response("OK");
}, { password: [Deno.env.get("VAL_PASSWORD"), Deno.env.get("STEVE_PASSWORD")] }); Note that authenticating using your api token remain an option even after setting a password. TODO [x] allow to authenticate using a val town token [ ] add a way to send an email to ask a password from the val owner [ ] automatically extend the session [ ] automatically remove expired sessions FAQ How to sign out ? Navigate to <your-site>/signout .
TodoApp
@summerboys
HTTP
SSR React Mini & SQLite Todo App This Todo App is server rendered and client-hydrated React. This architecture is a lightweight alternative to NextJS, RemixJS, or other React metaframeworks with no compile or build step. The data is saved server-side in Val Town SQLite . SSR React Mini Framework This "framework" is currently 44 lines of code, so it's obviously not a true replacement for NextJS or Remix. The trick is client-side importing the React component that you're server rendering . Val Town is uniquely suited for this trick because it both runs your code server-side and exposes vals as modules importable by the browser. The tricky part is making sure that server-only code doesn't run on the client and vice-versa. For example, because this val colocates the server-side loader and action with the React component we have to be careful to do all server-only imports (ie sqlite) dynamically inside the loader and action , so they only run server-side.
TodoApp
@mjweaver01
HTTP
SSR React Mini & SQLite Todo App This Todo App is server rendered and client-hydrated React. This architecture is a lightweight alternative to NextJS, RemixJS, or other React metaframeworks with no compile or build step. The data is saved server-side in Val Town SQLite . SSR React Mini Framework This "framework" is currently 44 lines of code, so it's obviously not a true replacement for NextJS or Remix. The trick is client-side importing the React component that you're server rendering . Val Town is uniquely suited for this trick because it both runs your code server-side and exposes vals as modules importable by the browser. The tricky part is making sure that server-only code doesn't run on the client and vice-versa. For example, because this val colocates the server-side loader and action with the React component we have to be careful to do all server-only imports (ie sqlite) dynamically inside the loader and action , so they only run server-side.
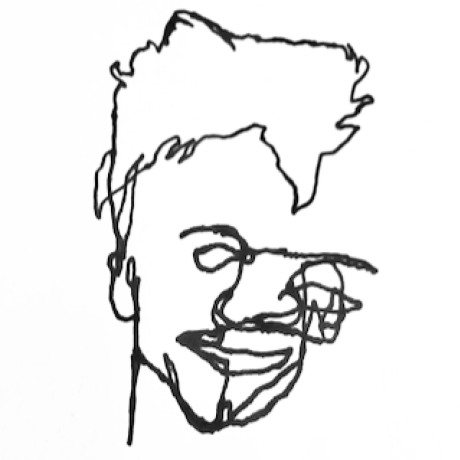
password_auth
@parkerdavis
Script
Password Auth Middleware Protect your vals behind a password. Use session cookies to persist authentication. Demo See @pomdtr/password_auth_test Usage If you want to use an api token to authenticate: import { passwordAuth } from "https://esm.town/v/pomdtr/password_auth";
export default passwordAuth(() => {
return new Response("OK");
}); Or if you prefer to use a string: import { passwordAuth } from "https://esm.town/v/pomdtr/password_auth";
export default passwordAuth(() => {
return new Response("OK");
}, { password: Deno.env.get("VAL_PASSWORD") }); You can also set multiple ones import { passwordAuth } from "https://esm.town/v/pomdtr/password_auth";
export default passwordAuth(() => {
return new Response("OK");
}, { password: [Deno.env.get("VAL_PASSWORD"), Deno.env.get("STEVE_PASSWORD")] }); Note that authenticating using your api token remain an option even after setting a password. TODO [x] allow to authenticate using a val town token [ ] add a way to send an email to ask a password from the val owner [ ] automatically extend the session [ ] automatically remove expired sessions FAQ How to sign out ? Navigate to <your-site>/signout .
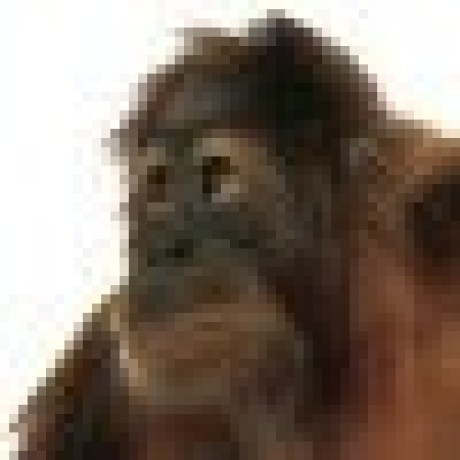
email_auth
@pomdtr
Script
Email Auth for Val Town ⚠️ Require a pro account (needed to send email to users) Usage Create an http server, and wrap it in the emailAuth middleware. import { emailAuth } from "https://esm.town/v/pomdtr/email_auth"
export default emailAuth((req) => {
return new Response(`your mail is ${req.headers.get("X-User-Email")}`);
}, {
verifyEmail: (email) => { return email == "steve@val.town" }
}); 💡 If you do not want to put your email in clear text, just move it to an env var (ex: Deno.env.get("email") ) If you want to allow anyone to access your val, just use: import { emailAuth } from "https://esm.town/v/pomdtr/email_auth"
export default emailAuth((req) => {
return new Response(`your mail is ${req.headers.get("X-User-Email")}`);
}, {
verifyEmail: (_email) => true
}); Each time someone tries to access your val but is not allowed, you will get an email with: the email of the user trying to log in the name of the val the he want to access You can then just add the user to your whitelist to allow him in (and the user will not need to confirm his email again) ! TODO [ ] Add expiration for verification codes and session tokens [ ] use links instead of code for verification [ ] improve errors pages
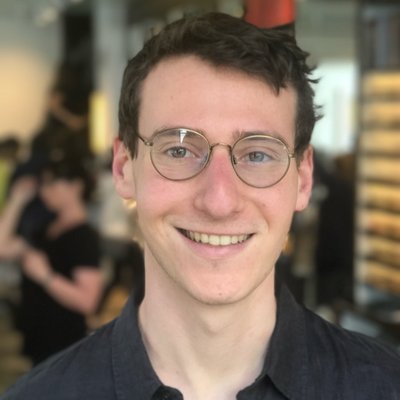
password_auth
@stevekrouse
Script
Password Auth Middleware Protect your vals behind a password. Use session cookies to persist authentication. Demo See @pomdtr/password_auth_test Usage If you want to use an api token to authenticate: import { passwordAuth } from "https://esm.town/v/pomdtr/password_auth";
export default passwordAuth(() => {
return new Response("OK");
}); Or if you prefer to use a string: import { passwordAuth } from "https://esm.town/v/pomdtr/password_auth";
export default passwordAuth(() => {
return new Response("OK");
}, { password: Deno.env.get("MY_PASSWORD") }); Or if you want to share your val with someone without sharing your main password, you can set multiple ones import { passwordAuth } from "https://esm.town/v/pomdtr/password_auth";
export default passwordAuth(() => {
return new Response("OK");
}, { password: [Deno.env.get("MY_PASSWORD"), Deno.env.get("STEVE_PASSWORD")] }); Note that authenticating using your api token is always an option. TODO [x] allow to authenticate using a val town token [ ] add a way to send an email to ask a password from the val owner [ ] automatically extend the session [ ] automatically remove expired sessions FAQ How to sign out ? Navigate to <your-site>/signout .
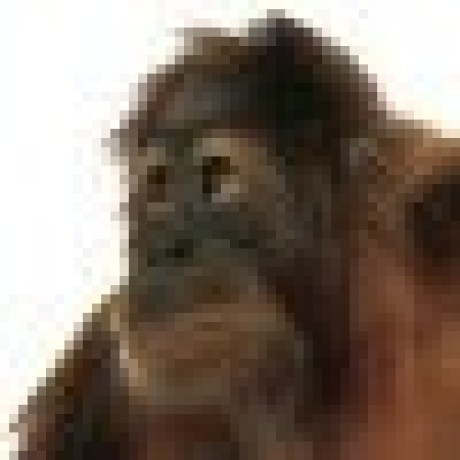
password_auth
@pomdtr
Script
Password Auth Middleware Protect your vals behind a password. Use session cookies to persist authentication. Demo See @pomdtr/password_auth_test Usage import { passwordAuth } from "https://esm.town/v/pomdtr/password_auth?v=84";
export default passwordAuth(() => {
return new Response("OK");
}, { verifyPassword: (password) => password == Deno.env.get("VAL_PASSWORD") }); If you want to use an api token to authenticate: import { passwordAuth } from "https://esm.town/v/pomdtr/password_auth?v=84";
import { verifyToken } from "https://esm.town/v/pomdtr/verifyToken";
export default passwordAuth(() => {
return new Response("OK");
}, { verifyPassword: verifyToken }); TODO [x] allow to authenticate using a val town token [ ] add a way to send an email to ask a password from the val owner [ ] automatically extend the session [ ] automatically remove expired sessions FAQ How to sign out ? Navigate to <your-site>/signout .