Back to packages list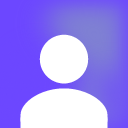
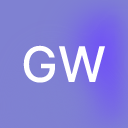
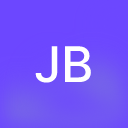
Vals using axios
Description from the NPM package:
Promise based HTTP client for the browser and node.js
sendNotification
@doublelotus
Script
Push Notification Sender This val can be used in other vals to send notifications to a segment using OneSignal's REST API This is really handy if you want to send push notifications to your phone without building a native app! I built a barebones React PWA that asks for a password then loads the OneSignal Web SDK that I deployed to Netlify for free. OneSignal has easy to follow docs so you can build this functionality into a React, Angular, Vue app or even Wordpress! Then install the PWA on your platform of choice and you're off to the races! Setup Save your ONESIGNAL_TOKEN and SEGMENT_APPID from OneSignal to your Val Town environment variables Import into another val! import sendNotification from "https://esm.town/v/gwoods22/sendNotification";
sendNotification
@gwoods22
Script
Push Notification Sender This val can be used in other vals to send notifications to a segment using OneSignal's REST API This is really handy if you want to send push notifications to your phone without building a native app! I built a barebones React PWA that asks for a password then loads the OneSignal Web SDK that I deployed to Netlify for free. OneSignal has easy to follow docs so you can build this functionality into a React, Angular, Vue app or even Wordpress! Then install the PWA on your platform of choice and you're off to the races! Setup Save your ONESIGNAL_TOKEN and SEGMENT_APPID from OneSignal to your Val Town environment variables Import into another val! import sendNotification from "https://esm.town/v/gwoods22/sendNotification";
zohoDeskApi
@julbrs
Script
Zoho Desk API A set of method to easily grab information on Zoho Desk! Official API Documentation is here . Notes It's needed to create a Self Client Application as described here to use this val. You also need to find your org id (under Setup > API > Zoho Service Communication (ZSC) Key > OrgId). Methods As of today here is the methods in this val: refreshAccessToken to generate a valid Access Token based on client id, client secret and a refresh token. Follow the step here to register a client and generate a refresh token. notAssignedTickets to extract the currently not assigned tickets in a specified department