Notion API examples & templates
Use these vals as a playground to view and fork Notion API examples and templates on Val Town. Run any example below or find templates that can be used as a pre-built solution.
charmaine
NotionJsSDK
Notion SDK for JavaScript A simple and easy to use client for the Notion API This template shows you how to use the Notion SDK. This starter template was ported from this one on GitHub . To run it: Click Fork on this val Get your Notion API key at https://www.notion.so/profile/integrations Add it to your Environment Variables as NOTION_TOKEN Click Run on this script val
Script
michaelfromyeg
notion2wallabag
Notion2Wallabag Intended to be run with Notion's webhooks. When fired, tries to save a Notion database item with a "Link" property to a Wallabag instance. Cannot be re-used for other workspaces, since it requires various Wallabag secrets and a Notion-specific token. Feel free to fork!
HTTP
supersayan
getAllNotionDbRows
Get All Rows of a Database in Notion Reference: Query a Database How to get an access token: https://developers.notion.com/reference/create-a-token
Script
nerdymomocat
add_to_notion_from_todoist
Use todoist for quick notes to add to notion. Uses project to decide which project to fetch to add stuff to notion. Can add to page or database based on config below. Demarkation using sections in the todoist project. Extracts date for page blocks that are added as callouts.
Cron
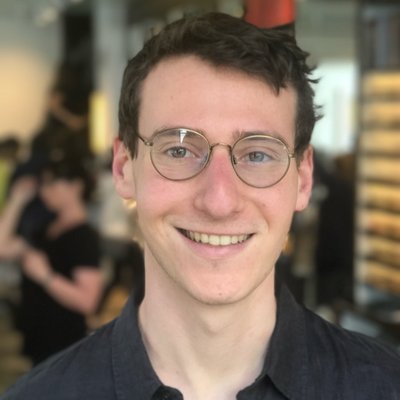
stevekrouse
notionGetDatabase
Get all the pages in a notion database Usage Find your databaseId : https://developers.notion.com/reference/retrieve-a-database Get auth by setting up an internal integration: https://developers.notion.com/docs/authorization#internal-integration-auth-flow-set-up Example usage: @stevekrouse.dateMeNotionDatabase deno-notion-sdk docs: https://github.com/cloudydeno/deno-notion_sdk
Script
nerdymomocat
add_to_notion_w_ai
Uses instructor and open ai (with gpt-4-turbo) to process any content into a notion database entry. Use addToNotion with any database id and content. await addToNotion(
"DB_ID_GOES_HERE",
"CONTENT_GOES HERE"//"for example: $43.28 ordered malai kofta and kadhi (doordash) [me and mom] jan 3 2024"
); Prompts are created based on your database name, database description, property name, property type, property description, and if applicable, property options (and their descriptions). Supports: checkbox, date, multi_select, number, rich_text, select, status, title, url, email Uses NOTION_API_KEY , OPENAI_API_KEY stored in env variables and uses Valtown blob storage to store information about the database. Use get_notion_db_info to use the stored blob if exists or create one, use get_and_save_notion_db_info to create a new blob (and replace an existing one if exists).
Script
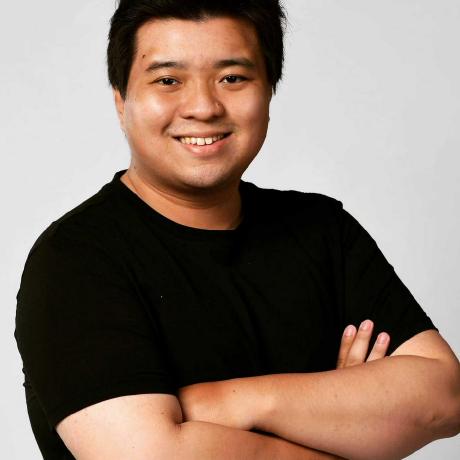
thomasatflexos
redirect
This is a handler for when Notion redirects during OAuth 2.0 workflow
Express (deprecated)
nerdymomocat
add_to_notion_w_ai_webpage
Example usage of the add_to_notion_w_ai val Try with the money database . Read and watch the demo run here
HTTP
bao
notionDbCalendarFeed
Publishes a Notion database with a date property to a calendar feed. Create a Notion integration in http://notion.so/profile/integrations Go to the Notion database -> click ••• from top right -> Connections -> Connect to, select the integration from last step Fork this val Set NOTION_API_TOKEN and NOTION_EVENTS_DATABASE_ID in https://www.val.town/settings/environment-variables Update datePropertyName and maxEventAgeInMonths if necessary
HTTP