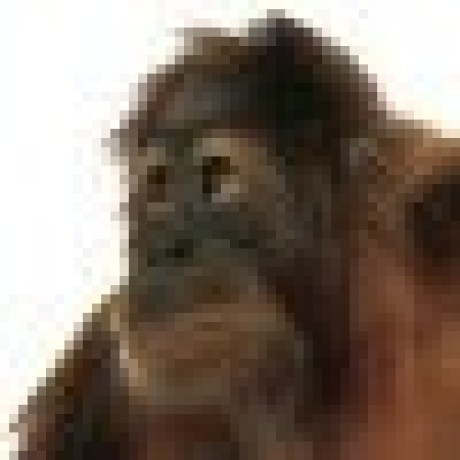
pomdtr
Val Town Basic Auth
Add basic auth on top of any http val
Usage
Wrap your HTTP handler in the basicAuth
middleware.
import { basicAuth } from "https://esm.town/v/pomdtr/basicAuth";
function handler(req: Request) {
return new Response("You are authenticated!");
}
export default basicAuth(handler);
To authenticate, paste an api token in the password prompt.
Lowdb Example
This val demonstrates the integration between valtown and lowdb.
Read the Lodash section if you want to give superpowers to your DB.
Augmented run api
This val is a wrapper on top of the val.town run api, improving it with additional features:
- basic auth
- content-type header in response based on url file extension
Usage
Custom Content-Type
The content-type will be inferred from the filename using the mime-types library.
If you use a .html
extension, the response will be interpreted as text/html
~ $ curl -v 'https://pomdtr-run.web.val.run/pomdtr/helloWorld.html' HTTP/1.1 200 OK ... Content-Type: text/html; charset=utf-8 ... Hello, World!
If you switch the extension to .txt
, the content-type header switch to text/raw
.
~ $ curl -v 'https://pomdtr-run.web.val.run/pomdtr/helloWorld.txt' HTTP/1.1 200 OK ... Content-Type: text/plain; charset=utf-8 ... Hello, World!
Passing arguments
The request is proxyed to the run api, so you can pass args to your vals via query params or body. See the run api docs for more details.
~ $ curl -X POST -d '{"args": ["pomdtr"]}' 'https://pomdtr-run.web.val.run/pomdtr/helloWorld.html' ... < content-type: text/html; charset=utf-8 ... Hello, pomdtr!
Basic Authentication
Just add your val town token as the username:
curl 'https://<val-token>@pomdtr-run.web.val.run/pomdtr/privateVal.txt'
Fetch the source of a val
This val was created before the introduction of https://esm.town
Usage
curl https://pomdtr-raw.web.val.run/<author>/<name>.<extension>[?v=<version>]
To see the code of this val, use https://pomdtr-raw.web.val.run/pomdtr/raw.ts
Examples
Fetching the val code
$ curl https://pomdtr-raw.web.val.run/pomdtr/add.tsx
You can also use js
, jsx
and ts
extension (only the content-type change, there is no transpilation).
Fetching private val
Pass an api token as an username
$ curl "https://<token>@pomdtr-raw.web.val.run/pomdtr/privateVal.ts"
Fetching the val README
$ curl https://pomdtr-raw.web.val.run/pomdtr/add.md
Getting an image
$ curl https://pomdtr-raw.web.val.run/pomdtr/add.png
Fetching a specific version of a val
$ curl https://pomdtr-raw.web.val.run/pomdtr/raw.ts?v=66
You need to be authenticated to use this method.
Fetching the val metadata
$ curl https://pomdtr-raw.web.val.run/pomdtr/add.json
Running vals locally using Deno
Create a new val.ts
file referencing the @pomdtr.add
import { add } from "https://pomdtr-raw.web.val.run/pomdtr/add.ts";
console.log(add(1, 2));
then use deno run
$ deno run ./val.ts
3
If you val accept a request and return a response, you can pass it to Deno.Serve
to run it locally!
import {raw} from "https://pomdtr-raw.web.val.run/pomdtr/raw.ts";
Deno.serve(raw);
If your val is private, you can set the DENO_AUTH_TOKENS env.
DENO_AUTH_TOKENS=<val-town-token>@pomdtr-raw.web.val.run
Usage

Serve prefixed blobs.
Usage
Create valimport { serveBlobs } from "https://esm.town/v/pomdtr/serve_blobs"
export default serveBlobs({
root: "public/"
})
All your blobs prefixed by public/
will be publicly accessible.
Ex: Go to https://pomdtr-public.web.val.run/example.json to view the blob public/example.json
from my account.
Awesome Val Town
An curated list of useful community vals. Feel free to create your own awesome list!
Apps
- @pomdtr/blob_editor
- @nbbaier/sqliteExplorerApp View and interact with your Val Town SQLite data.
- @pomdtr/http_client Attach a postman-like http client to your vals
- VS Code Extension
- vt
- Chrome Extension
Tooling
Authentication
Sqlite
Blob
Middleware
Testing
Api
Other
OpenAI
Web Components
SSR + Hydration Demo
Look at @pomdtr/island and @pomdtr/hydrate_islands to read the whole library source code (less than 50 rows!).
Email Auth for Val Town
⚠️ Require a pro account (needed to send email to users)
Usage
Create an http server, and wrap it in the emailAuth middleware.
Create valimport { emailAuth } from "https://www.val.town/v/pomdtr/email_auth"
export default emailAuth((req, ctx) => {
return new Response(`your mail is ${ctx.email}`);
});
When an user access the val, he will need to input his mail, then confirm it through a confirmation code.
You can limit how can access your vals through an allowList:
Create valimport { emailAuth } from "https://www.val.town/v/pomdtr/email_auth"
export default emailAuth((req, ctx) => {
return new Response(`your mail is ${ctx.email}`);
}, {
allowList: ["steve@val.town"]
});
If someone tries to access your val but is not in the allowlist, he will be blocked.
If you want to allow user to request for access, you can mix allowList
with allowSignup
:
Create valimport { emailAuth } from "https://www.val.town/v/pomdtr/email_auth"
export default emailAuth((req, ctx) => {
return new Response(`your mail is ${ctx.email}`);
}, {
allowList: ["steve@val.town"],
allowSignup: true
});
Each time a new user not present in the allowList try to login to a val, you will receive an email containing:
- the email of the user trying to log in
- the name of the val the he want to access
You can then just add the user to your whitelist to allow him in (and the user will not need to confirm his email again) !
Tips
If you don't want to put your email in clear text, you can just use an env variable:
Create valimport { emailAuth } from "https://www.val.town/v/pomdtr/email_auth"
export default emailAuth((req, ctx) => {
return new Response(`your mail is ${ctx.email}`);
}, {
allowList: [Deno.env.get("email")]
});
Or just setup a forward val (see @pomdtr/inbox):
Create valimport { emailAuth } from "https://www.val.town/v/pomdtr/email_auth"
export default emailAuth((req, ctx) => {
return new Response(`your mail is ${ctx.email}`);
}, {
allowList: ["pomdtr.inbox@valtown.email"]
});
TODO
- Add expiration for verification codes and session tokens
- use links instead of code for verification
- improve errors pages