Search
aoc_2023_21_1
@robsimmons
An interactive, runnable TypeScript val by robsimmons
Script
const DISTANCE = 6;
const dusa = new Dusa(`
# AOC Day 21
#builtin NAT_SUCC s
# Input to start and node facts
aocDay1
@nbbaier
Advent of Code 2023 - Day 1 solutions
Script
// #folder:aoc2023
// @aoc2023
// @title Day 1 solutions
import getAocData from "https://esm.town/v/nbbaier/getAocData";
const data = await getAocData(1);
const input = (await data.text()).split("\n").slice(0, -1);
aocDay3
@nbbaier
// @title Day 3 solutions
Script
// #folder:aoc2023
// @aoc2023
// @title Day 3 solutions
import getAocData from "https://esm.town/v/nbbaier/getAocData";
const data = await (await getAocData(3)).text();
const input = data.split("\n");
aoc_2023_15_2
@robsimmons
An interactive, runnable TypeScript val by robsimmons
Script
focal: line[label.length] === '-' ? null : parseInt(line.slice(label.length + 1)),
const dusa = new Dusa(`
# AOC Day 15, Part 2
#builtin INT_PLUS plus
#builtin INT_TIMES times
aoc_2023_15_1
@robsimmons
An interactive, runnable TypeScript val by robsimmons
Script
.map((line) => ({ length: line.length, ascii: line.split("").map((ch) => ch.charCodeAt(0)) }));
const dusa = new Dusa(`
# AOC Day 15, Part 1
#builtin INT_PLUS plus
#builtin INT_TIMES times
getAocData
@nbbaier
Helper function to get Advent of Code data AOC_TOKEN is a session token
Script
## Helper function to get Advent of Code data
`AOC_TOKEN` is a session token
return await fetch(`https://adventofcode.com/${year}/day/${day}/input`, {
headers: {
"Cookie": Deno.env.get("AOC_TOKEN"),
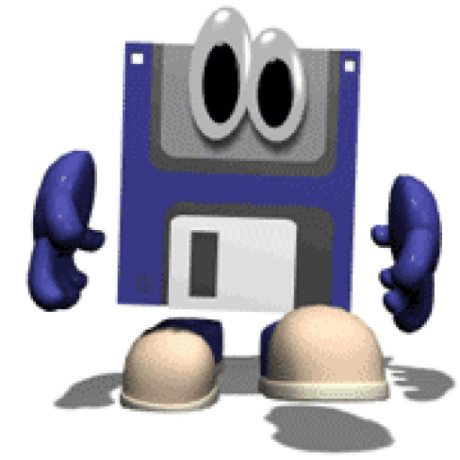
example_rust_http_val
@saolsen
Built from https://gist.github.com/saolsen/294683088bae9a8f9a8cf93e2b392729
See https://gist.github.com/saolsen/d273bb1baba5e912e4dc2b187511affa for how to build a rust val.
See https://www.val.town/v/saolsen/use_example_rust_http_val for how to use this.
Script
const bytes = base64.decodeBase64(
// base64 encoded wasm module
"AGFzbQEAAAABiwEUYAJ/fwF/YAJ/fwBgA39/fwF/YAF/AX9gA39/fwBgAX8AYAR/f39/AGAAAX9gBX9/f39/AGAEf39/fwF/YAZ/f39/f38Bf2AHf39/f39/fwF/YAZ+f39/f38BfmADfn9/AX9gB39/f39/f38AYAJ+fwBgA39+fgBgBH9+fn8AYAV/f39/fwF/YAAAAjcBGF9fd2JpbmRnZW5fcGxhY2Vob2xkZXJfXxpfX3diZ19sb2dfMThiMTE3NTRjNTc5M2MwNAABA4ABfwMFAAIKBAACAQAGCwAMDQQABQEAAAAHDgABBgQIDwYEBgEAAQUIEAUFBAQEBAEDAAAAAAEBABEBBgUEAQQCAQQEAAUSAxMBCQEFBwcEAAEFBQMDAQIAAAkEAgMCAAgAAAUEAQEBAAABAwADAwEDAAADAwMDAwECAgQEAAADAwUEBQFwASgoBQMBABEGCQF/AUGAgMAACwdxBgZtZW1vcnkCAAdoYW5kbGVyABgfX193YmluZGdlbl9hZGRfdG9fc3RhY2tfcG9pbnRlcgBxEV9fd2JpbmRnZW5fbWFsbG9jAEISX193YmluZGdlbl9yZWFsbG9jAEgPX193YmluZGdlbl9mcmVlAFkJLQEAQQELJykZcGBRXBY2f1Z/WhUxTmdmNX8+DTJQY2Q/aEoaJH9lb11qMBR/ZQqrmgJ/hSECD38BfiMAQRBrIgskAAJAAkACQAJAAkAgAEH1AU8EQEEIQQgQVyEGQRRBCBBXIQVBEEEIEFchAUEAQRBBCBBXQQJ0ayICQYCAfCABIAUgBmpqa0F3cUEDayIBIAEgAksbIABNDQUgAEEEakEIEFchBEHkk8EAKAIARQ0EQQAgBGshAwJ/QQAgBEGAAkkNABpBHyAEQf///wdLDQAaIARBBiAEQQh2ZyIAa3ZBAXEgAEEBdGtBPmoLIgZBAnRByJDBAGooAgAiAUUEQEEAIQBBACEFDAILIAQgBhBTdCEHQQAhAEEAIQUDQAJAIAEQciICIARJDQAgAiAEayICIANPDQAgASEFIAIiAw0AQQAhAyABIQAMBAsgAUEUaigCACICIAAgAiABIAdBHXZBBHFqQRBqKAIAIgFHGyAAIAIbIQAgB0EBdCEHIAENAAsMAQtBECAAQQRqQRBBCBBXQQVrIABLG0EIEFchBEHgk8EAKAIAIgEgBEEDdiIAdiICQQNxBEACQCACQX9zQQFxIABqIgNBA3QiAEHgkcEAaigCACIFQQhqKAIAIgIgAEHYkcEAaiIARwRAIAIgADYCDCAAIAI2AggMAQtB4JPBACABQX4gA3dxNgIACyAFIANBA3QQTyAFEH0hAwwFCyAEQeiTwQAoAgBNDQMCQAJAAkACQAJAAkAgAkUEQEHkk8EAKAIAIgBFDQogABBraEECdEHIkMEAaigCACIBEHIgBGshAyABEFIiAARAA0AgABByIARrIgIgAyACIANJIgIbIQMgACABIAIbIQEgABBSIgANAAsLIAEgBBB7IQUgARASQRBBCBBXIANLDQIgASAEEG0gBSADEFRB6JPBACgCACIADQEMBQsCQEEBIABBH3EiAHQQWyACIAB0cRBraCICQQN0IgBB4JHBAGooAgAiA0EIaigCACIBIABB2JHBAGoiAEcEQCABIAA2AgwgACABNgIIDAELQeCTwQBB4JPBACgCAEF+IAJ3cTYCAAsgAyAEEG0gAyAEEHsiBSACQQN0IARrIgIQVEHok8EAKAIAIgANAgwDCyAAQXhxQdiRwQBqIQdB8JPBACgCACEGAn9B4JPBACgCACICQQEgAEEDdnQiAHEEQCAHKAIIDAELQeCTwQAgACACcjYCACAHCyEAIAcgBjYCCCAAIAY2AgwgBiAHNgIMIAYgADYCCAwDCyABIAMgBGoQTwwDCyAAQXhxQdiRwQBqIQdB8JPBACgCACEGAn9B4JPBACgCACIBQQEgAEEDdnQiAHEEQCAHKAIIDAELQeCTwQAgACABcjYCACAHCyEAIAcgBjYCCCAAIAY2AgwgBiAHNgIMIAYgADYCCAtB8JPBACAFNgIAQeiTwQAgAjYCACADEH0hAwwGC0Hwk8EAIAU2AgBB6JPBACADNgIACyABEH0iA0UNAwwECyAAIAVyRQRAQQAhBUEBIAZ0EFtB5JPBACgCAHEiAEUNAyAAEGtoQQJ0QciQwQBqKAIAIQALIABFDQELA0AgACAFIAAQciIBIARPIAEgBGsiAiADSXEiARshBSACIAMgARshAyAAEFIiAA0ACwsgBUUNACAEQeiTwQAoAgAiAE0gAyAAIARrT3ENACAFIAQQeyEGIAUQEgJAQRBBCBBXIANNBEAgBSAEEG0gBiADEFQgA0GAAk8EQCAGIAMQEwwCCyADQXhxQdiRwQBqIQICf0Hgk8EAKAIAIgFBASADQQN2dCIAcQRAIAIoAggMAQtB4JPBACAAIAFyNgIAIAILIQAgAiAGNgIIIAAgBjYCDCAGIAI2AgwgBiAANgIIDAELIAUgAyAEahBPCyAFEH0iAw0BCwJAAkACQAJAAkACQAJAIARB6JPBACgCACIASwRAIARB7JPBACgCACIATwRAQQhBCBBXIARqQRRBCBBXakEQQQgQV2pBgIAEEFciAEEQdkAAIQEgC0EANgIIIAtBACAAQYCAfHEgAUF/RiIAGzYCBCALQQAgAUEQdCAAGzYCACALKAIAIghFBEBBACEDDAoLIAsoAgghDEH4k8EAIAsoAgQiCkH4k8EAKAIAaiIBNgIAQfyTwQBB/JPBACgCACIAIAEgACABSxs2AgACQAJAQfSTwQAoAgAEQEHIkcEAIQADQCAAEG4gCEYNAiAAKAIIIgANAAsMAgtBhJTBACgCACIARSAAIAhLcg0EDAkLIAAQdA0AIAAQdSAMRw0AIAAoAgAiAkH0k8EAKAIAIgFNBH8gAiAAKAIEaiABSwVBAAsNBAtBhJTBAEGElMEAKAIAIgAgCCAAIAhJGzYCACAIIApqIQFByJHBACEAAkACQANAIAEgACgCAEcEQCAAKAIIIgANAQwCCwsgABB0DQAgABB1IAxGDQELQfSTwQAoAgAhCUHIkcEAIQACQANAIAkgACgCAE8EQCAAEG4gCUsNAgsgACgCCCIADQALQQAhAAsgCSAAEG4iBkEUQQgQVyIPa0EXayIBEH0iAEEIEFcgAGsgAWoiACAAQRBBCBBXIAlqSRsiDRB9IQ4gDSAPEHshAEEIQQgQVyEDQRRBCBBXIQVBEEEIEFchAkH0k8EAIAggCBB9IgFBCBBXIAFrIgEQeyIHNgIAQeyTwQAgCkEIaiACIAMgBWpqIAFqayIDNgIAIAcgA0EBcjYCBEEIQQgQVyEFQRRBCBBXIQJBEEEIEFchASAHIAMQeyABIAIgBUEIa2pqNgIEQYCUwQBBgICAATYCACANIA8QbUHIkcEAKQIAIRAgDkEIakHQkcEAKQIANwIAIA4gEDcCAEHUkcEAIAw2AgBBzJHBACAKNgIAQciRwQAgCDYCAEHQkcEAIA42AgADQCAAQQQQeyAAQQc2AgQiAEEEaiAGSQ0ACyAJIA1GDQkgCSANIAlrIgAgCSAAEHsQTSAAQYACTwRAIAkgABATDAoLIABBeHFB2JHBAGohAgJ/QeCTwQAoAgAiAUEBIABBA3Z0IgBxBEAgAigCCAwBC0Hgk8EAIAAgAXI2AgAgAgshACACIAk2AgggACAJNgIMIAkgAjYCDCAJIAA2AggMCQsgACgCACEDIAAgCDYCACAAIAAoAgQgCmo2AgQgCBB9IgVBCBBXIQIgAxB9IgFBCBBXIQAgCCACIAVraiIGIAQQeyEHIAYgBBBtIAMgACABa2oiACAEIAZqayEEQfSTwQAoAgAgAEcEQCAAQfCTwQAoAgBGDQUgACgCBEEDcUEBRw0HAkAgABByIgVBgAJPBEAgABASDAELIABBDGooAgAiAiAAQQhqKAIAIgFHBEAgASACNgIMIAIgATYCCAwBC0Hgk8EAQeCTwQAoAgBBfiAFQQN2d3E2AgALIAQgBWohBCAAIAUQeyEADAcLQfSTwQAgBzYCAEHsk8EAQeyTwQAoAgAgBGoiADYCACAHIABBAXI2AgQgBhB9IQMMCQtB7JPBACAAIARrIgE2AgBB9JPBAEH0k8EAKAIAIgIgBBB7IgA2AgAgACABQQFyNgIEIAIgBBBtIAIQfSEDDAgLQfCTwQAoAgAhAkEQQQgQVyAAIARrIgFLDQMgAiAEEHshAEHok8EAIAE2AgBB8JPBACAANgIAIAAgARBUIAIgBBBtIAIQfSEDDAcLQYSUwQAgCDYCAAwECyAAIAAoAgQgCmo2AgRB7JPBACgCACAKaiEBQfSTwQAoAgAiACAAEH0iAEEIEFcgAGsiABB7IQNB7JPBACABIABrIgU2AgBB9JPBACADNgIAIAMgBUEBcjYCBEEIQQgQVyECQRRBCBBXIQFBEEEIEFchACADIAUQeyAAIAEgAkEIa2pqNgIEQYCUwQBBgICAATYCAAwEC0Hwk8EAIAc2AgBB6JPBAEHok8EAKAIAIARqIgA2AgAgByAAEFQgBhB9IQMMBAtB8JPBAEEANgIAQeiTwQAoAgAhAEHok8EAQQA2AgAgAiAAEE8gAhB9IQMMAwsgByAEIAAQTSAEQYACTwRAIAcgBBATIAYQfSEDDAMLIARBeHFB2JHBAGohAgJ/QeCTwQAoAgAiAUEBIARBA3Z0IgBxBEAgAigCCAwBC0Hgk8EAIAAgAXI2AgAgAgshACACIAc2AgggACAHNgIMIAcgAjYCDCAHIAA2AgggBhB9IQMMAgtBiJTBAEH/HzYCAEHUkcEAIAw2AgBBzJHBACAKNgIAQciRwQAgCDYCAEHkkcEAQdiRwQA2AgBB7JHBAEHgkcEANgIAQeCRwQBB2JHBADYCAEH0kcEAQeiRwQA2AgBB6JHBAEHgkcEANgIAQfyRwQBB8JHBADYCAEHwkcEAQeiRwQA2AgBBhJLBAEH4kcEANgIAQfiRwQBB8JHBADYCAEGMksEAQYCSwQA2AgBBgJLBAEH4kcEANgIAQZSSwQBBiJLBADYCAEGIksEAQYCSwQA2AgBBnJLBAEGQksEANgIAQZCSwQBBiJLBADYCAEGkksEAQZiSwQA2AgBBmJLBAEGQksEANgIAQaCSwQBBmJLBADYCAEGsksEAQaCSwQA2AgBBqJLBAEGgksEANgIAQbSSwQBBqJLBADYCAEGwksEAQaiSwQA2AgBBvJLBAEGwksEANgIAQbiSwQBBsJLBADYCAEHEksEAQbiSwQA2AgBBwJLBAEG4ksEANgIAQcySwQBBwJLBADYCAEHIksEAQcCSwQA2AgBB1JLBAEHIksEANgIAQdCSwQBByJLBADYCAEHcksEAQdCSwQA2AgBB2JLBAEHQksEANgIAQeSSwQBB2JLBADYCAEHsksEAQeCSwQA2AgBB4JLBAEHYksEANgIAQfSSwQBB6JLBADYCAEHoksEAQeCSwQA2AgBB/JLBAEHwksEANgIAQfCSwQBB6JLBADYCAEGEk8EAQfiSwQA2AgBB+JLBAEHwksEANgIAQYyTwQBBgJPBADYCAEGAk8EAQfiSwQA2AgBBlJPBAEGIk8EANgIAQYiTwQBBgJPBADYCAEGck8EAQZCTwQA2AgBBkJPBAEGIk8EANgIAQaSTwQBBmJPBADYCAEGYk8EAQZCTwQA2AgBBrJPBAEGgk8EANgIAQaCTwQBBmJPBADYCAEG0k8EAQaiTwQA2AgBBqJPBAEGgk8EANgIAQbyTwQBBsJPBADYCAEGwk8EAQaiTwQA2AgBBxJPBAEG4k8EANgIAQbiTwQBBsJPBADYCAEHMk8EAQcCTwQA2AgBBwJPBAEG4k8EANgIAQdSTwQBByJPBADYCAEHIk8EAQcCTwQA2AgBB3JPBAEHQk8EANgIAQdCTwQBByJPBADYCAEHYk8EAQdCTwQA2AgBBCEEIEFchBUEUQQgQVyECQRBBCBBXIQFB9JPBACAIIAgQfSIAQQgQVyAAayIAEHsiAzYCAEHsk8EAIApBCGogASACIAVqaiAAamsiBTYCACADIAVBAXI2AgRBCEEIEFchAkEUQQgQVyEBQRBBCBBXIQAgAyAFEHsgACABIAJBCGtqajYCBEGAlMEAQYCAgAE2AgALQQAhA0Hsk8EAKAIAIgAgBE0NAEHsk8EAIAAgBGsiATYCAEH0k8EAQfSTwQAoAgAiAiAEEHsiADYCACAAIAFBAXI2AgQgAiAEEG0gAhB9IQMLIAtBEGokACADC5EHAQV/IAAQfiIAIAAQciIBEHshAgJAAkAgABBzDQAgACgCACEDIAAQbEUEQCABIANqIQEgACADEHwiAEHwk8EAKAIARgRAIAIoAgRBA3FBA0cNAkHok8EAIAE2AgAgACABIAIQTQ8LIANBgAJPBEAgABASDAILIABBDGooAgAiBCAAQQhqKAIAIgVHBEAgBSAENgIMIAQgBTYCCAwCC0Hgk8EAQeCTwQAoAgBBfiADQQN2d3E2AgAMAQsgASADakEQaiEADAELAkAgAhBpBEAgACABIAIQTQwBCwJAAkACQEH0k8EAKAIAIAJHBEAgAkHwk8EAKAIARg0BIAIQciIDIAFqIQECQCADQYACTwRAIAIQEgwBCyACQQxqKAIAIgQgAkEIaigCACICRwRAIAIgBDYCDCAEIAI2AggMAQtB4JPBAEHgk8EAKAIAQX4gA0EDdndxNgIACyAAIAEQVCAAQfCTwQAoAgBHDQRB6JPBACABNgIADwtB9JPBACAANgIAQeyTwQBB7JPBACgCACABaiICNgIAIAAgAkEBcjYCBCAAQfCTwQAoAgBGDQEMAgtB8JPBACAANgIAQeiTwQBB6JPBACgCACABaiICNgIAIAAgAhBUDwtB6JPBAEEANgIAQfCTwQBBADYCAAsgAkGAlMEAKAIATQ0BQQhBCBBXIQBBFEEIEFchAkEQQQgQVyEDQQBBEEEIEFdBAnRrIgFBgIB8IAMgACACamprQXdxQQNrIgAgACABSxtFDQFB9JPBACgCAEUNAUEIQQgQVyEAQRRBCBBXIQJBEEEIEFchAUEAIQMCQEHsk8EAKAIAIgQgASACIABBCGtqaiIATQ0AIAQgAGtB//8DakGAgHxxIgRBgIAEayECQfSTwQAoAgAhAUHIkcEAIQACQANAIAEgACgCAE8EQCAAEG4gAUsNAgsgACgCCCIADQALQQAhAAsgABB0DQAgACgCDBoMAAsQF0EAIANrRw0BQeyTwQAoAgBBgJTBACgCAE0NAUGAlMEAQX82AgAPCyABQYACTwRAIAAgARATQYiUwQBBiJTBACgCAEEBayIANgIAIAANARAXGg8LIAFBeHFB2JHBAGohAgJ/QeCTwQAoAgAiA0EBIAFBA3Z0IgFxBEAgAigCCAwBC0Hgk8EAIAEgA3I2AgAgAgshAyACIAA2AgggAyAANgIMIAAgAjYCDCAAIAM2AggLC/gGAQh/AkACQCAAQQNqQXxxIgMgAGsiBiABSw0AIAEgBmsiB0EESQ0AIAdBA3EhCEEAIQECQCAAIANGDQAgBkEDcSEEAkAgAyAAQX9zakEDSQRAQQAhAwwBCyAGQXxxIQlBACEDA0AgASAAIANqIgIsAABBv39KaiACQQFqLAAAQb9/SmogAkECaiwAAEG/f0pqIAJBA2osAABBv39KaiEBIAkgA0EEaiIDRw0ACwsgBEUNACAAIANqIQIDQCABIAIsAABBv39KaiEBIAJBAWohAiAEQQFrIgQNAAsLIAAgBmohAwJAIAhFDQAgAyAHQXxxaiIALAAAQb9/SiEFIAhBAUYNACAFIAAsAAFBv39KaiEFIAhBAkYNACAFIAAsAAJBv39KaiEFCyAHQQJ2IQYgASAFaiEEA0AgAyEAIAZFDQJBwAEgBiAGQcABTxsiA0EDcSEFIANBAnQhCAJAIANB/AFxIgdFBEBBACECDAELIAAgB0ECdGohCUEAIQIgACEBA0AgAUUNASACIAEoAgAiAkF/c0EHdiACQQZ2ckGBgoQIcWogAUEEaigCACICQX9zQQd2IAJBBnZyQYGChAhxaiABQQhqKAIAIgJBf3NBB3YgAkEGdnJBgYKECHFqIAFBDGooAgAiAkF/c0EHdiACQQZ2ckGBgoQIcWohAiABQRBqIgEgCUcNAAsLIAYgA2shBiAAIAhqIQMgAkEIdkH/gfwHcSACQf+B/AdxakGBgARsQRB2IARqIQQgBUUNAAsCf0EAIABFDQAaIAAgB0ECdGoiACgCACIBQX9zQQd2IAFBBnZyQYGChAhxIgEgBUEBRg0AGiABIAAoAgQiAUF/c0EHdiABQQZ2ckGBgoQIcWoiASAFQQJGDQAaIAAoAggiAEF/c0EHdiAAQQZ2ckGBgoQIcSABagsiAUEIdkH/gRxxIAFB/4H8B3FqQYGABGxBEHYgBGohBAwBCyABRQRAQQAPCyABQQNxIQMCQCABQQRJBEAMAQsgAUF8cSEFA0AgBCAAIAJqIgEsAABBv39KaiABQQFqLAAAQb9/SmogAUECaiwAAEG/f0pqIAFBA2osAABBv39KaiEEIAUgAkEEaiICRw0ACwsgA0UNACAAIAJqIQEDQCAEIAEsAABBv39KaiEEIAFBAWohASADQQFrIgMNAAsLIAQLiAcBCH8CQAJAIAAoAgAiCiAAKAIIIgRyBEACQCAERQ0AIAEgAmohCSAAQQxqKAIAQQFqIQcgASEEA0ACQCAEIQMgB0EBayIHRQ0AIAMgCUYNAgJ/IAMsAAAiBUEATgRAIAVB/wFxIQUgA0EBagwBCyADLQABQT9xIQggBUEfcSEEIAVBX00EQCAEQQZ0IAhyIQUgA0ECagwBCyADLQACQT9xIAhBBnRyIQggBUFwSQRAIAggBEEMdHIhBSADQQNqDAELIARBEnRBgIDwAHEgAy0AA0E/cSAIQQZ0cnIiBUGAgMQARg0DIANBBGoLIgQgBiADa2ohBiAFQYCAxABHDQEMAgsLIAMgCUYNACADLAAAIgRBAE4gBEFgSXIgBEFwSXJFBEAgBEH/AXFBEnRBgIDwAHEgAy0AA0E/cSADLQACQT9xQQZ0IAMtAAFBP3FBDHRycnJBgIDEAEYNAQsCQAJAIAZFDQAgAiAGTQRAQQAhAyACIAZGDQEMAgtBACEDIAEgBmosAABBQEgNAQsgASEDCyAGIAIgAxshAiADIAEgAxshAQsgCkUNAiAAKAIEIQYCQCACQRBPBEAgASACEAMhAwwBCyACRQRAQQAhAwwBCyACQQNxIQcCQCACQQRJBEBBACEDQQAhBQwBCyACQXxxIQlBACEDQQAhBQNAIAMgASAFaiIELAAAQb9/SmogBEEBaiwAAEG/f0pqIARBAmosAABBv39KaiAEQQNqLAAAQb9/SmohAyAJIAVBBGoiBUcNAAsLIAdFDQAgASAFaiEEA0AgAyAELAAAQb9/SmohAyAEQQFqIQQgB0EBayIHDQALCyADIAZPDQFBACEEIAYgA2siAyEGAkACQAJAIAAtACBBAWsOAgABAgtBACEGIAMhBAwBCyADQQF2IQQgA0EBakEBdiEGCyAEQQFqIQMgAEEYaigCACEEIABBFGooAgAhBSAAKAIQIQACQANAIANBAWsiA0UNASAFIAAgBCgCEBEAAEUNAAtBAQ8LQQEhAwJAIABBgIDEAEYNACAFIAEgAiAEKAIMEQIADQBBACEDAn8DQCAGIAMgBkYNARogA0EBaiEDIAUgACAEKAIQEQAARQ0ACyADQQFrCyAGSSEDCyADDwsMAQsgACgCFCABIAIgAEEYaigCACgCDBECAA8LIAAoAhQgASACIABBGGooAgAoAgwRAgAL+wUBB38CfyABBEBBK0GAgMQAIAAoAhwiB0EBcSIBGyEKIAEgBWoMAQsgACgCHCEHQS0hCiAFQQFqCyEIAkAgB0EEcUUEQEEAIQIMAQsCQCADQRBPBEAgAiADEAMhAQwBCyADRQRAQQAhAQwBCyADQQNxIQkCQCADQQRJBEBBACEBDAELIANBfHEhDEEAIQEDQCABIAIgBmoiCywAAEG/f0pqIAtBAWosAABBv39KaiALQQJqLAAAQb9/SmogC0EDaiwAAEG/f0pqIQEgDCAGQQRqIgZHDQALCyAJRQ0AIAIgBmohBgNAIAEgBiwAAEG/f0pqIQEgBkEBaiEGIAlBAWsiCQ0ACwsgASAIaiEICwJAAkAgACgCAEUEQEEBIQEgAEEUaigCACIGIABBGGooAgAiACAKIAIgAxBEDQEMAgsgCCAAKAIEIgZPBEBBASEBIABBFGooAgAiBiAAQRhqKAIAIgAgCiACIAMQRA0BDAILIAdBCHEEQCAAKAIQIQsgAEEwNgIQIAAtACAhDEEBIQEgAEEBOgAgIABBFGooAgAiByAAQRhqKAIAIgkgCiACIAMQRA0BIAYgCGtBAWohAQJAA0AgAUEBayIBRQ0BIAdBMCAJKAIQEQAARQ0AC0EBDwtBASEBIAcgBCAFIAkoAgwRAgANASAAIAw6ACAgACALNgIQQQAhAQwBCyAGIAhrIgYhCAJAAkACQCAALQAgIgFBAWsOAwABAAILQQAhCCAGIQEMAQsgBkEBdiEBIAZBAWpBAXYhCAsgAUEBaiEBIABBGGooAgAhBiAAQRRqKAIAIQcgACgCECEAAkADQCABQQFrIgFFDQEgByAAIAYoAhARAABFDQALQQEPC0EBIQEgAEGAgMQARg0AIAcgBiAKIAIgAxBEDQAgByAEIAUgBigCDBECAA0AQQAhAQNAIAEgCEYEQEEADwsgAUEBaiEBIAcgACAGKAIQEQAARQ0ACyABQQFrIAhJDwsgAQ8LIAYgBCAFIAAoAgwRAgALtgsBBX8jAEEQayIDJAACQAJAAkACQAJAAkACQAJAAkACQCABDigFCAgICAgICAgBAwgIAggICAgICAgICAgICAgICAgICAgIBggICAgHAAsgAUHcAEYNAwwHCyAAQYAEOwEKIABCADcBAiAAQdzoATsBAAwHCyAAQYAEOwEKIABCADcBAiAAQdzkATsBAAwGCyAAQYAEOwEKIABCADcBAiAAQdzcATsBAAwFCyAAQYAEOwEKIABCADcBAiAAQdy4ATsBAAwECyAAQYAEOwEKIABCADcBAiAAQdzgADsBAAwDCyACQYCABHFFDQEgAEGABDsBCiAAQgA3AQIgAEHcxAA7AQAMAgsgAkGAAnFFDQAgAEGABDsBCiAAQgA3AQIgAEHczgA7AQAMAQsCQAJAAkACQCACQQFxBEACfyABQQt0IQZBISEFQSEhAgJAA0ACQAJAQX8gBUEBdiAEaiIFQQJ0QbCJwQBqKAIAQQt0IgcgBkcgBiAHSxsiB0EBRgRAIAUhAgwBCyAHQf8BcUH/AUcNASAFQQFqIQQLIAIgBGshBSACIARLDQEMAgsLIAVBAWohBAsCfwJAAn8CQCAEQSBNBEAgBEECdCIFQbCJwQBqKAIAQRV2IQIgBEEgRw0BQdcFIQVBHwwCCyAEQSFBuIjBABAqAAsgBUG0icEAaigCAEEVdiEFIARFDQEgBEEBawtBAnRBsInBAGooAgBB////AHEMAQtBAAshBAJAAkAgBSACQX9zakUNACABIARrIQdB1wUgAiACQdcFTRshBiAFQQFrIQVBACEEA0AgAiAGRg0CIAQgAkG0isEAai0AAGoiBCAHSw0BIAUgAkEBaiICRw0ACyAFIQILIAJBAXEMAQsgBkHXBUHIiMEAECoACw0BCwJ/AkAgAUEgSQ0AAkACf0EBIAFB/wBJDQAaIAFBgIAESQ0BAkAgAUGAgAhPBEAgAUGwxwxrQdC6K0kgAUHLpgxrQQVJciABQZ70C2tB4gtJIAFB4dcLa0GfGElyciABQX5xQZ7wCkYgAUGinQtrQQ5JcnINBCABQWBxQeDNCkcNAQwECyABQZT9wABBLEHs/cAAQcQBQbD/wABBwgMQDAwEC0EAIAFBuu4Ka0EGSQ0AGiABQYCAxABrQfCDdEkLDAILIAFB8oLBAEEoQcKDwQBBnwJB4YXBAEGvAhAMDAELQQALRQ0BIAAgATYCBCAAQYABOgAADAQLIANBCGpBADoAACADQQA7AQYgA0H9ADoADyADIAFBD3FB2IjBAGotAAA6AA4gAyABQQR2QQ9xQdiIwQBqLQAAOgANIAMgAUEIdkEPcUHYiMEAai0AADoADCADIAFBDHZBD3FB2IjBAGotAAA6AAsgAyABQRB2QQ9xQdiIwQBqLQAAOgAKIAMgAUEUdkEPcUHYiMEAai0AADoACSABQQFyZ0ECdkECayIBQQtPDQEgA0EGaiABaiICQZSJwQAvAAA7AAAgAkECakGWicEALQAAOgAAIAAgAykBBjcAACAAQQhqIANBDmovAQA7AAAgAEEKOgALIAAgAToACgwDCyADQQhqQQA6AAAgA0EAOwEGIANB/QA6AA8gAyABQQ9xQdiIwQBqLQAAOgAOIAMgAUEEdkEPcUHYiMEAai0AADoADSADIAFBCHZBD3FB2IjBAGotAAA6AAwgAyABQQx2QQ9xQdiIwQBqLQAAOgALIAMgAUEQdkEPcUHYiMEAai0AADoACiADIAFBFHZBD3FB2IjBAGotAAA6AAkgAUEBcmdBAnZBAmsiAUELTw0BIANBBmogAWoiAkGUicEALwAAOwAAIAJBAmpBlonBAC0AADoAACAAIAMpAQY3AAAgAEEIaiADQQ5qLwEAOwAAIABBCjoACyAAIAE6AAoMAgsgAUEKQYSJwQAQKwALIAFBCkGEicEAECsACyADQRBqJAAL3RwDCn8HfgF8IwBB4ABrIgYkAAJAAkACQAJAAkACQAJAAkAgAC0AAEEBaw4FAQIDBgQACyABKAIAQcKBwABBBBBiDAQLIAEoAgBBvoHAAEG5gcAAIAAtAAEiABtBBEEFIAAbEGIMAwsCQAJAAkAgACgCCEEBaw4CAQIACyAGQShqIQNBFCECAkAgAEEQaikDACIMQpDOAFQEQCAMIQ0MAQsDQCACIANqIgBBBGsgDCAMQpDOAIAiDUKQzgB+faciBEH//wNxQeQAbiIFQQF0QZCTwABqLwAAOwAAIABBAmsgBCAFQeQAbGtB//8DcUEBdEGQk8AAai8AADsAACACQQRrIQIgDEL/wdcvViANIQwNAAsLIA2nIgBB4wBKBEAgAyACQQJrIgJqIAAgAEHkAG4iAEHkAGxrQQF0QZCTwABqLwAAOwAACyAGQQhqIQQCQCAAQQpOBEAgAyACQQJrIgJqIABBAXRBkJPAAGovAAA7AAAMAQsgAyACQQFrIgJqIABBMGo6AAALIARBFCACazYCBCAEIAIgA2o2AgAgASgCACAGKAIIIAYoAgwQYgwECyAGQShqIQNBFCECAkAgAEEQaikDACIOQj+HIgwgDoUgDH0iDEKQzgBUBEAgDCENDAELA0AgAiADaiIAQQRrIAwgDEKQzgCAIg1CkM4Afn2nIgRB//8DcUHkAG4iBUEBdEGQk8AAai8AADsAACAAQQJrIAQgBUHkAGxrQf//A3FBAXRBkJPAAGovAAA7AAAgAkEEayECIAxC/8HXL1YgDSEMDQALCyANpyIAQeMASgRAIAMgAkECayICaiAAIABB5ABuIgBB5ABsa0EBdEGQk8AAai8AADsAAAsCQCAAQQpOBEAgAyACQQJrIgJqIABBAXRBkJPAAGovAAA7AAAMAQsgAyACQQFrIgJqIABBMGo6AAALIA5CAFMEQCADIAJBAWsiAmpBLToAAAsgBkEQaiIAQRQgAms2AgQgACACIANqNgIAIAEoAgAgBigCECAGKAIUEGIMAwsgAEEQaisDACITIBNiBH9BAAVBAUECQQQgE70iDEKAgICAgICA+P8AgyINUCIAGyANQoCAgICAgID4/wBRG0EDQQQgABsgDEL/////////B4NQGwtB/wFxQQJPBEAgBkEoaiEDIwBBEGsiBSQAIBO9IgxC/////////weDIQ0gDEIAUwRAIANBLToAAEEBIQQLAkACfwJAAkACQAJAAkACQAJAAkACQAJAIA1CAFIiAiAMQjSIp0H/D3EiAHIEQCACIABBAklyIQogDUKAgICAgICACIQgDSAAGyIMQgKGIQ4gDEIBgyERAkACQAJAIABBtQhrQcx3IAAbIgBBAEgEQEEBIQIgACAAQYWiU2xBFHZBACAAayILQQFLayIIaiEHIAwgCyAIayIAQQR0Qbi/wABqIAggAEHPpsoAbEETdmtB/ABqIAUgBUEIaiAKEA4hDyAFKQMIIQwgBSkDACENIAhBAkkNASAIQT9JDQIMBQtBACECIAwgAEHB6ARsQRJ2IABBA0trIgdBBHRB2JTAAGogByAAayAHQc+mygBsQRN2akH9AGogBSAFQQhqIAoQDiEPIAUpAwghDCAFKQMAIQ0gB0EWSQ0CDAULIA0gEX0hDSAKIBFQcSEJDAULIA5CfyAIrYZCf4WDUCECDAMLQQAgDqdrIA5CBYCnQXtsRgRAQX8hAANAIABBAWohACAOQs2Zs+bMmbPmTH4iDkK05syZs+bMmTNUDQALIAAgB08hAgwDCyARUEUEQCAOQgKEIQ5BfyEAA0AgAEEBaiEAIA5CzZmz5syZs+ZMfiIOQrTmzJmz5syZM1QNAAsgDSAAIAdPrX0hDQwDCyAKrUJ/hSAOfCEOQX8hAANAIABBAWohACAOQs2Zs+bMmbPmTH4iDkK05syZs+bMmTNUDQALIAAgB08hCQwBCyADIARqIgBB4OnAAC8AADsAACAAQQJqQeLpwAAtAAA6AAAgDEI/iKdBA2ohAAwLC0EAIQILIAkNACACRQ0BC0EAIQggDUIKgCIQIAxCCoAiElYNAUEAIQAgDCEOIA8hDQwCC0EAIQAgDULkAIAiDiAMQuQAgCIQVg0CIAwhECANIQ4gDyEMQQAhAgwFC0EAIQADQCAJQQAgDKdrIBIiDqdBdmxGcSEJIABBAWohACACIAhB/wFxRXEhAiAPpyAPQgqAIg2nQXZsaiEIIA0hDyAQQgqAIhAgDiIMQgqAIhJWDQALCyAJRQ0BQQAgDqdrIA5CCoAiD6dBdmxHDQEDQCAAQQFqIQAgAiAIQf8BcUVxIQIgDacgDUIKgCIMp0F2bGohCCAMIQ1BACAPp2sgDyIOQgqAIg+nQXZsRg0ACwwCCyAPpyAPQuQAgCIMp0Gcf2xqQTFLIQJBAiEADAILIA0hDAsgEacgCUF/c3IgDCAOUXFBBEEFIAxCAYNQGyAIIAhB/wFxQQVGGyAIIAIbQf8BcUEES3IMAQsgDkIKgCIOIBBCCoAiDVYEfwNAIABBAWohACAMIg9CCoAhDCAOQgqAIg4gDSIQQgqAIg1WDQALIA+nIAynQXZsakEESwUgAgsgDCAQUXILIQICQAJAAkAgACAHaiIHQQBOIAcCf0ERIAwgAq18IgxC//+D/qbe4RFWDQAaQRAgDEL//5mm6q/jAVYNABpBDyAMQv//6IOx3hZWDQAaQQ4gDEL/v8rzhKMCVg0AGkENIAxC/5+UpY0dVg0AGkEMIAxC/8/bw/QCVg0AGkELIAxC/8evoCVWDQAaQQogDEL/k+vcA1YNABpBCSAMQv/B1y9WDQAaQQggDEL/rOIEVg0AGkEHIAxCv4Q9Vg0AGkEGIAxCn40GVg0AGkEFIAxCj84AVg0AGkEEIAxC5wdWDQAaQQMgDELjAFYNABpBAkEBIAxCCVYbCyIAaiICQRFIcUUEQCACQQFrIgdBEEkNASACQQRqQQVJDQIgAyAEaiIIQQFqIQIgAEEBRw0DIAJB5QA6AAAgCCAMp0EwajoAACAHIAMgBEECciIAahAjIABqIQAMBAsgDCADIAAgBGpqIggQHiAAIAJIBEAgCEEwIAcQegsgAyACIARqIgBqQa7gADsAACAAQQJqIQAMAwsgDCADIAAgBEEBaiIHaiIAahAeIAMgBGogAyAHaiACEHkgAyACIARqakEuOgAADAILIAMgBGoiCEGw3AA7AABBAiACayEHIAJBAEgEQCAIQQJqQTBBAyAHIAdBA0wbQQJrEHoLIAwgAyAAIARqIAdqIgBqEB4MAQsgDCAAIARqIgAgA2pBAWoiBBAeIAggAi0AADoAACACQS46AAAgBEHlADoAACAHIAMgAEECaiIAahAjIABqIQALIAVBEGokACABKAIAIAMgABBiDAMLIAEoAgBBwoHAAEEEEGIMAgsgBkHYAGogASAAKAIEIABBDGooAgAQOUEAIQAgBi0AWEEERg0DIAYgBikDWDcDKCAGQShqEEUhAAwDCyAAQQRqIgIoAgghBCABQRBqQQA6AABBASEAIAFBDGoiAyADKAIAIgNBAWo2AgAgASgCACIFQZOCwABBARBiIARFBEAgASADNgIMIAVBkYLAAEEBEGJBACEACyAGQShqIQMCfyACKAIAIgVFBEBBACECQQAMAQsgAyAFNgIYIANBADYCFCADIAU2AgggA0EANgIEIAMgAigCBCIFNgIcIAMgBTYCDCACKAIIIQJBAQshBSADIAI2AiAgAyAFNgIQIAMgBTYCACAGQSBqIAMQLgJAAkAgBigCICIDBEAgBigCJCEEIAMhAgNAIAEoAgAiBUGQgsAAQZSCwAAgAEH/AXFBAUYiABtBAUECIAAbEGIgASgCDCIABEAgASgCCCEHIAEoAgQhCANAIAUgCCAHEGIgAEEBayIADQALCyAGQdAAaiABIAIoAgAgAigCCBA5IAYtAFBBBEcEQCAGIAYpA1A3A1ggBkHYAGoQRSEADAcLIAVBloLAAEECEGIgBCABEAciAA0GIAFBAToAECAGQRhqIAZBKGoQLkECIQAgBigCHCEEIAYoAhgiAg0ACyABIAEoAgxBAWsiADYCDCADRQ0BIAEoAgAiAkGQgsAAQQEQYiAARQ0CIAEoAgghAyABKAIEIQEDQCACIAEgAxBiIABBAWsiAA0ACwwCCyAERQ0CIAEgASgCDEEBazYCDAsgASgCACECCyACQZGCwABBARBiC0EAIQAMAQsjAEEgayIDJAAgA0EQaiICIABBBGoiACgCACIENgIAIAIgBCAAKAIIQRhsajYCBCADIAMpAxA3AxggA0EIaiIAQQE2AgAgACADQRhqIgAoAgQgACgCAGtBGG42AgQgAygCDCEEIAMoAgghBSABQRBqQQA6AABBASEAIAFBDGoiAiACKAIAIgdBAWo2AgAgASgCACICQZKCwABBARBiAkACQAJAAkACQCAFRSAEckUEQCABIAc2AgwgAkGYgsAAQQEQYkEAIQAgAygCGCIEIAMoAhwiBUcNAQwECyADKAIYIgQgAygCHCIFRg0BCwNAIAEoAgAiAkGQgsAAQZSCwAAgAEH/AXFBAUYiABtBAUECIAAbEGIgASgCDCIABEAgASgCCCEHIAEoAgQhCANAIAIgCCAHEGIgAEEBayIADQALCyAEIAEQByIADQQgAUEBOgAQQQIhACAEQRhqIgQgBUcNAAsgASABKAIMQQFrIgA2AgwgAyAFNgIYIAEoAgAiAkGQgsAAQQEQYiAARQ0BIAEoAgghBCABKAIEIQEDQCACIAEgBBBiIABBAWsiAA0ACwwBCyABIAc2AgwgAyAFNgIYCyACQZiCwABBARBiC0EAIQALIANBIGokAAsgBkHgAGokACAAC4MFAQp/IwBBMGsiAyQAIANBIGogATYCACADQQM6ACggA0EgNgIYIANBADYCJCADIAA2AhwgA0EANgIQIANBADYCCAJ/AkACQCACKAIQIgpFBEAgAkEMaigCACIARQ0BIAIoAgghASAAQQN0IQUgAEEBa0H/////AXFBAWohByACKAIAIQADQCAAQQRqKAIAIgQEQCADKAIcIAAoAgAgBCADKAIgKAIMEQIADQQLIAEoAgAgA0EIaiABQQRqKAIAEQAADQMgAUEIaiEBIABBCGohACAFQQhrIgUNAAsMAQsgAkEUaigCACIARQ0AIABBBXQhCyAAQQFrQf///z9xQQFqIQcgAigCACEAA0AgAEEEaigCACIBBEAgAygCHCAAKAIAIAEgAygCICgCDBECAA0DCyADIAUgCmoiAUEQaigCADYCGCADIAFBHGotAAA6ACggAyABQRhqKAIANgIkIAFBDGooAgAhBiACKAIIIQhBACEJQQAhBAJAAkACQCABQQhqKAIAQQFrDgIAAgELIAZBA3QgCGoiDCgCBEEhRw0BIAwoAgAoAgAhBgtBASEECyADIAY2AgwgAyAENgIIIAFBBGooAgAhBAJAAkACQCABKAIAQQFrDgIAAgELIARBA3QgCGoiBigCBEEhRw0BIAYoAgAoAgAhBAtBASEJCyADIAQ2AhQgAyAJNgIQIAggAUEUaigCAEEDdGoiASgCACADQQhqIAEoAgQRAAANAiAAQQhqIQAgCyAFQSBqIgVHDQALCyACKAIEIAdLBEAgAygCHCACKAIAIAdBA3RqIgAoAgAgACgCBCADKAIgKAIMEQIADQELQQAMAQtBAQsgA0EwaiQAC80EAQR/IAAgARB7IQICQAJAAkAgABBzDQAgACgCACEDIAAQbEUEQCABIANqIQEgACADEHwiAEHwk8EAKAIARgRAIAIoAgRBA3FBA0cNAkHok8EAIAE2AgAgACABIAIQTQ8LIANBgAJPBEAgABASDAILIABBDGooAgAiBCAAQQhqKAIAIgVHBEAgBSAENgIMIAQgBTYCCAwCC0Hgk8EAQeCTwQAoAgBBfiADQQN2d3E2AgAMAQsgASADakEQaiEADAELIAIQaQRAIAAgASACEE0MAgsCQEH0k8EAKAIAIAJHBEAgAkHwk8EAKAIARg0BIAIQciIDIAFqIQECQCADQYACTwRAIAIQEgwBCyACQQxqKAIAIgQgAkEIaigCACICRwRAIAIgBDYCDCAEIAI2AggMAQtB4JPBAEHgk8EAKAIAQX4gA0EDdndxNgIACyAAIAEQVCAAQfCTwQAoAgBHDQNB6JPBACABNgIADAILQfSTwQAgADYCAEHsk8EAQeyTwQAoAgAgAWoiATYCACAAIAFBAXI2AgQgAEHwk8EAKAIARw0BQeiTwQBBADYCAEHwk8EAQQA2AgAPC0Hwk8EAIAA2AgBB6JPBAEHok8EAKAIAIAFqIgE2AgAgACABEFQPCw8LIAFBgAJPBEAgACABEBMPCyABQXhxQdiRwQBqIQICf0Hgk8EAKAIAIgNBASABQQN2dCIBcQRAIAIoAggMAQtB4JPBACABIANyNgIAIAILIQEgAiAANgIIIAEgADYCDCAAIAI2AgwgACABNgIIC98CAQV/QRBBCBBXIABLBEBBEEEIEFchAAtBCEEIEFchA0EUQQgQVyECQRBBCBBXIQQCQEEAQRBBCBBXQQJ0ayIFQYCAfCAEIAIgA2pqa0F3cUEDayIDIAMgBUsbIABrIAFNDQAgAEEQIAFBBGpBEEEIEFdBBWsgAUsbQQgQVyIDakEQQQgQV2pBBGsQASICRQ0AIAIQfiEBAkAgAEEBayIEIAJxRQRAIAEhAAwBCyACIARqQQAgAGtxEH4hAkEQQQgQVyEEIAEQciACIABBACACIAFrIARNG2oiACABayICayEEIAEQbEUEQCAAIAQQSSABIAIQSSABIAIQCQwBCyABKAIAIQEgACAENgIEIAAgASACajYCAAsCQCAAEGwNACAAEHIiAkEQQQgQVyADak0NACAAIAMQeyEBIAAgAxBJIAEgAiADayIDEEkgASADEAkLIAAQfSEGIAAQbBoLIAYL3QIBBX8gACgCACIEQYwCaiIIIAAoAggiAEEMbGohBQJAIABBAWoiBiAELwGSAyIHSwRAIAUgASkCADcCACAFQQhqIAFBCGooAgA2AgAMAQsgCCAGQQxsaiAFIAcgAGsiCEEMbBB5IAVBCGogAUEIaigCADYCACAFIAEpAgA3AgAgBCAGQRhsaiAEIABBGGxqIAhBGGwQeQsgBCAAQRhsaiIBIAIpAwA3AwAgAUEQaiACQRBqKQMANwMAIAFBCGogAkEIaikDADcDACAEQZgDaiEBIABBAmoiAiAHQQJqIgVJBEAgASACQQJ0aiABIAZBAnRqIAcgAGtBAnQQeQsgASAGQQJ0aiADNgIAIAQgB0EBajsBkgMgBSAGSwRAIAdBAWohAiAAQQJ0IARqQZwDaiEBA0AgASgCACIDIABBAWoiADsBkAMgAyAENgKIAiABQQRqIQEgACACRw0ACwsL2QIBB39BASEJAkACQCACRQ0AIAEgAkEBdGohCiAAQYD+A3FBCHYhCyAAQf8BcSENA0AgAUECaiEMIAcgAS0AASICaiEIIAsgAS0AACIBRwRAIAEgC0sNAiAIIQcgDCIBIApGDQIMAQsCQAJAIAcgCE0EQCAEIAhJDQEgAyAHaiEBA0AgAkUNAyACQQFrIQIgAS0AACABQQFqIQEgDUcNAAtBACEJDAULIAcgCEGE/cAAEC0ACyAIIARBhP3AABAsAAsgCCEHIAwiASAKRw0ACwsgBkUNACAFIAZqIQMgAEH//wNxIQEDQCAFQQFqIQACQCAFLQAAIgLAIgRBAE4EQCAAIQUMAQsgACADRwRAIAUtAAEgBEH/AHFBCHRyIQIgBUECaiEFDAELQdj1wABBK0H0/MAAEEAACyABIAJrIgFBAEgNASAJQQFzIQkgAyAFRw0ACwsgCUEBcQuQBAEFfyMAQRBrIgMkACAAKAIAIQACQAJ/AkAgAUGAAU8EQCADQQA2AgwgAUGAEEkNASABQYCABEkEQCADIAFBP3FBgAFyOgAOIAMgAUEMdkHgAXI6AAwgAyABQQZ2QT9xQYABcjoADUEDDAMLIAMgAUE/cUGAAXI6AA8gAyABQQZ2QT9xQYABcjoADiADIAFBDHZBP3FBgAFyOgANIAMgAUESdkEHcUHwAXI6AAxBBAwCCyAAKAIIIgIgACgCBEYEQCMAQSBrIgQkAAJAAkAgAkEBaiICRQ0AQQggAEEEaigCACIGQQF0IgUgAiACIAVJGyICIAJBCE0bIgVBf3NBH3YhAgJAIAYEQCAEIAY2AhggBEEBNgIUIAQgACgCADYCEAwBCyAEQQA2AhQLIAQgAiAFIARBEGoQISAEKAIEIQIgBCgCAEUEQCAAIAI2AgAgAEEEaiAFNgIADAILIAJBgYCAgHhGDQEgAkUNACACIARBCGooAgAQdgALEEYACyAEQSBqJAAgACgCCCECCyAAIAJBAWo2AgggACgCACACaiABOgAADAILIAMgAUE/cUGAAXI6AA0gAyABQQZ2QcABcjoADEECCyEBIAEgACgCBCAAKAIIIgJrSwRAIAAgAiABEBwgACgCCCECCyAAKAIAIAJqIANBDGogARB4GiAAIAEgAmo2AggLIANBEGokAEEAC50CAgF/BH4jAEGQAWsiBiQAIAZBEGogASkDACIIIABCAoYiAEIChCIHECcgBkHgAGogASkDCCIKIAcQJyAGIAZBGGopAwAiByAGKQNgfCIJIAZB6ABqKQMAIAcgCVatfCACQUBrQf8AcSIBEDcgAyAGKQMANwMAIAZBMGogCCAAIAWtQn+FfCIHECcgBkHwAGogCiAHECcgBkEgaiAGQThqKQMAIgcgBikDcHwiCSAGQfgAaikDACAHIAlWrXwgARA3IAQgBikDIDcDACAGQdAAaiAIIAAQJyAGQYABaiAKIAAQJyAGQUBrIAZB2ABqKQMAIgAgBikDgAF8IgggBkGIAWopAwAgACAIVq18IAEQNyAGKQNAIAZBkAFqJAALwAICBX8BfiMAQTBrIgUkAEEnIQMCQCAAQpDOAFQEQCAAIQgMAQsDQCAFQQlqIANqIgRBBGsgACAAQpDOAIAiCEKQzgB+faciBkH//wNxQeQAbiIHQQF0Qb73wABqLwAAOwAAIARBAmsgBiAHQeQAbGtB//8DcUEBdEG+98AAai8AADsAACADQQRrIQMgAEL/wdcvViAIIQANAAsLIAinIgRB4wBLBEAgA0ECayIDIAVBCWpqIAinIgQgBEH//wNxQeQAbiIEQeQAbGtB//8DcUEBdEG+98AAai8AADsAAAsCQCAEQQpPBEAgA0ECayIDIAVBCWpqIARBAXRBvvfAAGovAAA7AAAMAQsgA0EBayIDIAVBCWpqIARBMGo6AAALIAIgAUHY9cAAQQAgBUEJaiADakEnIANrEAUgBUEwaiQAC7gCAQd/IwBBMGsiAyQAIAIgASgCACIFLwGSAyIHIAEoAggiBkF/c2oiATsBkgMgA0EQaiAFQYwCaiIIIAZBDGxqIglBCGooAgA2AgAgA0EgaiAFIAZBGGxqIgRBCGopAwA3AwAgA0EoaiAEQRBqKQMANwMAIAMgCSkCADcDCCADIAQpAwA3AxgCQCABQQxJBEAgByAGQQFqIgRrIAFHDQEgAkGMAmogCCAEQQxsaiABQQxsEHgaIAIgBSAEQRhsaiABQRhsEHgaIAUgBjsBkgMgACADKQMINwMAIABBCGogA0EQaigCADYCACAAIAMpAxg3AxAgAEEYaiADQSBqKQMANwMAIABBIGogA0EoaikDADcDACADQTBqJAAPCyABQQtB5I/AABAsAAtBrI/AAEEoQdSPwAAQQAALuAIBA38jAEGAAWsiBCQAAn8CQAJAIAEoAhwiAkEQcUUEQCACQSBxDQEgADUCAEEBIAEQDwwDCyAAKAIAIQBBACECA0AgAiAEakH/AGpBMEHXACAAQQ9xIgNBCkkbIANqOgAAIAJBAWshAiAAQRBJIABBBHYhAEUNAAsMAQsgACgCACEAQQAhAgNAIAIgBGpB/wBqQTBBNyAAQQ9xIgNBCkkbIANqOgAAIAJBAWshAiAAQRBJIABBBHYhAEUNAAsgAkGAAWoiAEGBAU8EQCAAQYABQaz3wAAQKwALIAFBAUG898AAQQIgAiAEakGAAWpBACACaxAFDAELIAJBgAFqIgBBgQFPBEAgAEGAAUGs98AAECsACyABQQFBvPfAAEECIAIgBGpBgAFqQQAgAmsQBQsgBEGAAWokAAu7AgEFfyAAKAIYIQMCQAJAIAAgACgCDEYEQCAAQRRBECAAQRRqIgEoAgAiBBtqKAIAIgINAUEAIQEMAgsgACgCCCICIAAoAgwiATYCDCABIAI2AggMAQsgASAAQRBqIAQbIQQDQCAEIQUgAiIBQRRqIgIgAUEQaiACKAIAIgIbIQQgAUEUQRAgAhtqKAIAIgINAAsgBUEANgIACwJAIANFDQACQCAAIAAoAhxBAnRByJDBAGoiAigCAEcEQCADQRBBFCADKAIQIABGG2ogATYCACABDQEMAgsgAiABNgIAIAENAEHkk8EAQeSTwQAoAgBBfiAAKAIcd3E2AgAPCyABIAM2AhggACgCECICBEAgASACNgIQIAIgATYCGAsgAEEUaigCACIARQ0AIAFBFGogADYCACAAIAE2AhgLC6ACAQR/IABCADcCECAAAn9BACABQYACSQ0AGkEfIAFB////B0sNABogAUEGIAFBCHZnIgJrdkEBcSACQQF0a0E+agsiAzYCHCADQQJ0QciQwQBqIQICQAJAAkACQEHkk8EAKAIAIgRBASADdCIFcQRAIAIoAgAhAiADEFMhAyACEHIgAUcNASACIQMMAgtB5JPBACAEIAVyNgIAIAIgADYCAAwDCyABIAN0IQQDQCACIARBHXZBBHFqQRBqIgUoAgAiA0UNAiAEQQF0IQQgAyICEHIgAUcNAAsLIAMoAggiASAANgIMIAMgADYCCCAAIAM2AgwgACABNgIIIABBADYCGA8LIAUgADYCAAsgACACNgIYIAAgADYCCCAAIAA2AgwLtwIBB38jAEEQayICJABBASEHAkACQCABKAIUIgRBJyABQRhqKAIAKAIQIgURAAANACACIAAoAgBBgQIQBgJAIAItAABBgAFGBEAgAkEIaiEGQYABIQMDQAJAIANBgAFHBEAgAi0ACiIAIAItAAtPDQQgAiAAQQFqOgAKIABBCk8NBiAAIAJqLQAAIQEMAQtBACEDIAZBADYCACACKAIEIQEgAkIANwMACyAEIAEgBREAAEUNAAsMAgtBCiACLQAKIgEgAUEKTRshACACLQALIgMgASABIANJGyEGA0AgASAGRg0BIAIgAUEBaiIDOgAKIAAgAUYNAyABIAJqIQggAyEBIAQgCC0AACAFEQAARQ0ACwwBCyAEQScgBREAACEHCyACQRBqJAAgBw8LIABBCkGYicEAECoAC6YCAQJ/IwBBEGsiAiQAIAAoAgAhAAJAAn8CQCABQYABTwRAIAJBADYCDCABQYAQSQ0BIAFBgIAESQRAIAIgAUE/cUGAAXI6AA4gAiABQQx2QeABcjoADCACIAFBBnZBP3FBgAFyOgANQQMMAwsgAiABQT9xQYABcjoADyACIAFBBnZBP3FBgAFyOgAOIAIgAUEMdkE/cUGAAXI6AA0gAiABQRJ2QQdxQfABcjoADEEEDAILIAAoAggiAyAAKAIERgR/IAAgAxA8IAAoAggFIAMLIAAoAgBqIAE6AAAgACAAKAIIQQFqNgIIDAILIAIgAUE/cUGAAXI6AA0gAiABQQZ2QcABcjoADEECCyEBIAAgAkEMaiIAIAAgAWoQPQsgAkEQaiQAQQALnwIBAn8jAEEQayICJAACQAJ/AkAgAUGAAU8EQCACQQA2AgwgAUGAEEkNASABQYCABEkEQCACIAFBP3FBgAFyOgAOIAIgAUEMdkHgAXI6AAwgAiABQQZ2QT9xQYABcjoADUEDDAMLIAIgAUE/cUGAAXI6AA8gAiABQQZ2QT9xQYABcjoADiACIAFBDHZBP3FBgAFyOgANIAIgAUESdkEHcUHwAXI6AAxBBAwCCyAAKAIIIgMgACgCBEYEfyAAIAMQPCAAKAIIBSADCyAAKAIAaiABOgAAIAAgACgCCEEBajYCCAwCCyACIAFBP3FBgAFyOgANIAIgAUEGdkHAAXI6AAxBAgshASAAIAJBDGoiACAAIAFqED0LIAJBEGokAEEAC10BDH9B0JHBACgCACICBEBByJHBACEGA0AgAiIBKAIIIQIgASgCBCEDIAEoAgAhBCABKAIMGiABIQYgBUEBaiEFIAINAAsLQYiUwQBB/x8gBSAFQf8fTRs2AgAgCAu1CgEGfyMAQTBrIgckACAHQRhqIAEgAhBBIAcoAhwhASAHKAIYIQogB0EQaiADIAQQQSAHKAIUIQIgBygCECEDIAdBCGogBSAGEEEgBygCDCAHKAIIIQwgB0EgaiEGIwBB0AFrIgQkAEGZgsAAQRsQACAEQdAAaiIIEGEgBEE4akEHEDQgBCgCPCEJIAQoAjgiBUG0gsAAKAAANgAAIAVBA2pBt4LAACgAADYAACAEQQc2AsgBIAQgCTYCxAEgBCAFNgLAASAEQTBqQRoQNCAEKAI0IQkgBCgCMCIFQbuCwAApAAA3AAAgBUEQakHLgsAAKQAANwAAIAVBCGpBw4LAACkAADcAACAFQRhqQdOCwAAvAAA7AAAgBEEaNgK0ASAEIAk2ArABIAQgBTYCrAEgBEEDOgCoASAEQZABaiAIIARBwAFqIARBqAFqEBsgBC0AkAFBBkcEQCAEQZABahBDCyAEQShqQQcQNCAEKAIsIQggBCgCKCIFQduCwAAoAAA2AAAgBUEDakHegsAAKAAANgAAIARBBzYCaCAEIAg2AmQgBCAFNgJgIARBgAFqIggQYSAEQSBqQQMQNCAEKAIkIQkgBCgCICIFQeKCwAAvAAA7AAAgBUECakHkgsAALQAAOgAAIARBAzYCyAEgBCAJNgLEASAEIAU2AsABIARBGGogAhA0IAQoAhwhBSAEKAIYIAMgAhB4IQkgBEEDOgCoASAEIAI2ArQBIAQgBTYCsAEgBCAJNgKsASAEIAQvAJABOwCpASAEIARBkgFqIgktAAA6AKsBIARBkAFqIAggBEHAAWogBEGoAWoQGyAELQCQAUEGRwRAIARBkAFqEEMLIARBEGpBBhA0IAQoAhQhCCAEKAIQIgVB5YLAACgAADYAACAFQQRqQemCwAAvAAA7AAAgBEEGNgLIASAEIAg2AsQBIAQgBTYCwAEgBEEIaiABEDQgBCgCDCEFIAQoAgggCiABEHghCCAEQQM6AKgBIAQgATYCtAEgBCAFNgKwASAEIAg2AqwBIAQgBC8AkAE7AKkBIAQgCS0AADoAqwEgBEGQAWogBEGAAWogBEHAAWogBEGoAWoQGyAELQCQAUEGRwRAIARBkAFqEEMLIARBtAFqIgUgBEGIAWooAgA2AgAgBCAEKQOAATcCrAEgBEEFOgCoASAEQZABaiAEQdAAaiAEQeAAaiAEQagBahAbIAQtAJABQQZHBEAgBEGQAWoQQwsgBSAEQdgAaigCADYCACAEIAQpA1A3AqwBIARBBToAqAEgBEGAARA0IARBADYCyAEgBCAEKQMANwPAASAEQZABaiIIQQRyIgVBADoADCAFQgI3AgQgBUHAisAANgIAIAQgBEHAAWo2ApABAkACQCAEQagBaiAIEAciBQRAIARBwAFqEFEMAQsgBCgCxAEhBSAEKALAASIIDQELIAQgBTYCkAFB1IHAAEErIARBkAFqQYCCwABB7ILAABAmAAsgBiAEKALIATYCCCAGIAU2AgQgBiAINgIAIARBqAFqEEMgBEHQAWokACAHKAIoIQYgBygCJCEEIAcoAiAhBQRAIAwQAgsgAgRAIAMQAgsgAQRAIAoQAgsCfyAFRQRAQQEhAkEAIQNBAAwBCyAHIAY2AiggByAENgIkIAcgBTYCICAHQSBqIgEQOiAHIAEoAgg2AgQgByABKAIANgIAIAcoAgQhA0EAIQRBACECIAcoAgALIQEgACACNgIMIAAgBDYCCCAAIAM2AgQgACABNgIAIAdBMGokAAvwCAEEfyMAQYABayIDJAAgACgCACEFIANBADYCUCADQgE3A0ggA0HYAGoiAEEDOgAgIABBIDYCECAAQQA2AhwgACADQcgAajYCFCAAQQA2AgggAEEANgIAIABBGGpBhITAADYCAAJ/AkACQAJAAkACQAJAAkACQAJAAkACQAJAAkACQAJAAkACQAJAAkACQAJAAkACQAJAAkAgBSgCAEEBaw4YAQIDBAUGBwgJCgsMDQ4PEBESExQVFhcYAAsgACAFKAIEIAVBCGooAgAQVQwYCwJ/IwBBQGoiAiQAAkACQAJAAkACQAJAIAVBBGoiBC0AAEEBaw4DAQIDAAsgAiAEKAIENgIEQY2QwQAtAAAaQRRBARBfIgRFDQQgBEEQakHE8sAAKAAANgAAIARBCGpBvPLAACkAADcAACAEQbTywAApAAA3AAAgAkKUgICAwAI3AgwgAiAENgIIIAJBNGpCAjcCACACQSRqQQ82AgAgAkEDNgIsIAJBrPDAADYCKCACQRA2AhwgAiACQRhqNgIwIAIgAkEEajYCICACIAJBCGo2AhggACACQShqEDMhACACKAIMRQ0DIAIoAggQAgwDCyAELQABIQQgAkE0akIBNwIAIAJBATYCLCACQajqwAA2AiggAkERNgIMIAIgBEECdCIEQcjywABqKAIANgIcIAIgBEHs88AAaigCADYCGCACIAJBCGo2AjAgAiACQRhqNgIIIAAgAkEoahAzIQAMAgsgBCgCBCIEKAIAIAQoAgQgABB3IQAMAQsgBCgCBCIEKAIAIAAgBCgCBCgCEBEAACEACyACQUBrJAAgAAwBC0EBQRQQdgALDBcLIABBwIXAAEEYEFUMFgsgAEHYhcAAQRsQVQwVCyAAQfOFwABBGhBVDBQLIABBjYbAAEEZEFUMEwsgAEGmhsAAQQwQVQwSCyAAQbKGwABBExBVDBELIABBxYbAAEETEFUMEAsgAEHYhsAAQQ4QVQwPCyAAQeaGwABBDhBVDA4LIABB9IbAAEEMEFUMDQsgAEGAh8AAQQ4QVQwMCyAAQY6HwABBDhBVDAsLIABBnIfAAEETEFUMCgsgAEGvh8AAQRoQVQwJCyAAQcmHwABBPhBVDAgLIABBh4jAAEEUEFUMBwsgAEGbiMAAQTQQVQwGCyAAQc+IwABBLBBVDAULIABB+4jAAEEkEFUMBAsgAEGficAAQQ4QVQwDCyAAQa2JwABBExBVDAILIABBwInAAEEcEFUMAQsgAEHcicAAQRgQVQtFBEAgA0FAayADQdAAaigCADYCACADQTRqQQM2AgAgA0EsakEDNgIAIANBFGpCAzcCACADIAMpA0g3AzggA0EENgIkIANBBDYCDCADQZCKwAA2AgggAyAFQRBqNgIwIAMgBUEMajYCKCADIANBOGo2AiAgAyADQSBqNgIQIAEgA0EIahAzIAMoAjwEQCADKAI4EAILIANBgAFqJAAPC0GchMAAQTcgA0EgakHUhMAAQbCFwAAQJgAL+gECBH8BfiMAQTBrIgIkACABQQRqIQQgASgCBEUEQCABKAIAIQMgAkEoaiIFQQA2AgAgAkIBNwMgIAIgAkEgajYCLCACQSxqQZDqwAAgAxAIGiACQRhqIAUoAgAiAzYCACACIAIpAyAiBjcDECAEQQhqIAM2AgAgBCAGNwIACyACQQhqIgMgBEEIaigCADYCACABQQxqQQA2AgAgBCkCACEGIAFCATcCBEGNkMEALQAAGiACIAY3AwBBDEEEEF8iAUUEQEEEQQwQdgALIAEgAikDADcCACABQQhqIAMoAgA2AgAgAEHc8cAANgIEIAAgATYCACACQTBqJAAL9BUCGH8BfiMAQUBqIgckACMAQRBrIgQkAAJAIAEoAgAiC0UEQCAHQQA2AhAgByABNgIMIAcgAikCADcCACAHQQhqIAJBCGooAgA2AgAMAQsgASgCBCEGIwBBIGsiCiQAIAogBjYCHCAKIAs2AhggCkEQaiAKQRhqIAIQICAKKAIUIQUCQCAKKAIQBEADQCAGRQRAIAQgCzYCBCAEQQxqIAU2AgAgBEEIakEANgIAQQEhCQwDCyALIAVBAnRqQZgDaigCACELIAogBkEBayIGNgIcIAogCzYCGCAKQQhqIApBGGogAhAgIAooAgwhBSAKKAIIDQALCyAEIAs2AgQgBEEMaiAFNgIAIARBCGogBjYCAAsgBCAJNgIAIApBIGokACAEKAIABEAgByABNgIMIAcgBCkCBDcCECAHIAIpAgA3AgAgB0EIaiACQQhqKAIANgIAIAdBGGogBEEMaigCADYCAAwBCyAHIAQpAgQ3AgQgB0EANgIAIAdBEGogATYCACAHQQxqIARBDGooAgA2AgAgAigCBEUNACACKAIAEAILIARBEGokAAJAIAcoAgAEQCAHQThqIAdBGGooAgA2AgAgB0EwaiAHQRBqKQMANwMAIAdBKGogB0EIaikDADcDACAHIAcpAwA3AyAjAEEwayIBJAACQCAHQSBqIgooAhBFBEAgCigCDCEEEEwiAkEBOwGSAyACQQA2AogCIAIgCikCADcCjAIgAkGUAmogCkEIaigCADYCACACIAMpAwA3AwAgAkEIaiADQQhqKQMANwMAIAJBEGogA0EQaikDADcDACAEQoCAgIAQNwIEIAQgAjYCAAwBCyABQQhqIApBEGoiAkEIaigCADYCACABIAIpAgA3AwAgAUEYaiAKQQhqKAIANgIAIAEgCikCADcDECABQSBqIRIgAUEQaiEEIApBDGohFyMAQbABayILJAAgC0EoaiEJIwBB0ABrIgIkAAJAIAEoAgAiBi8BkgMiDEELTwRAIAJBGGoiBSABKAIIEDggAiAGNgIIIAIgAigCGDYCECACQSBqKAIAIQYgAigCHCEMIAIgASgCBDYCDBBMIghBADsBkgMgCEEANgKIAiAFIAJBCGoiDSAIEBAgBUE0akEANgIAIAUgCDYCMCAFIA0pAgA3AyggAkHIAGogAkFAayAMGygCACIFQYwCaiIOIAZBDGxqIQggAkHMAGogAkHEAGogDBsoAgAhDwJAIAZBAWoiDCAFLwGSAyINSwRAIAggBCkCADcCACAIQQhqIARBCGooAgA2AgAMAQsgDiAMQQxsaiAIIA0gBmsiDkEMbBB5IAhBCGogBEEIaigCADYCACAIIAQpAgA3AgAgBSAMQRhsaiAFIAZBGGxqIA5BGGwQeQsgBSAGQRhsaiIEQRBqIANBEGopAwA3AwAgBCADKQMANwMAIARBCGogA0EIaikDADcDACAFIA1BAWo7AZIDIAkgAkEYakE4EHgiA0FAayAGNgIAIANBPGogDzYCACADIAU2AjgMAQsgBkGMAmoiDiABKAIIIgVBDGxqIQggASgCBCEPAkAgDCAFQQFqIg1JBEAgCCAEKQIANwIAIAhBCGogBEEIaigCADYCAAwBCyAOIA1BDGxqIAggDCAFayIOQQxsEHkgCEEIaiAEQQhqKAIANgIAIAggBCkCADcCACAGIA1BGGxqIAYgBUEYbGogDkEYbBB5CyAGIAVBGGxqIgRBEGogA0EQaikDADcDACAJIAY2AjggCUEGOgAQIAlBQGsgBTYCACAJQTxqIA82AgAgBCADKQMANwMAIARBCGogA0EIaikDADcDACAGIAxBAWo7AZIDCyACQdAAaiQAIAtB6ABqKAIAIRggC0HkAGooAgAhGSALKAJgIRoCQAJAAkAgCy0AOEEGRg0AIAsgC0EoakEoEHgiAigCXCEFIAIoAlghBiACKAJQIgMoAogCIgkEQCACKAJUIQQgAkEQaiEIA0AgAiAJNgJwIAIgAy8BkAM2AnggAiAEQQFqNgJ0IAJBkAFqIAJBCGooAgA2AgAgAiACKQMANwOIASACQagBaiAIQRBqKQMANwMAIAJBoAFqIAhBCGopAwA3AwAgAiAIKQMANwOYASACQShqIQ0gAkGIAWohDiACQZgBaiEPIwBB4ABrIgMkAAJAIAUgAkHwAGoiBCgCBCIJQQFrRgRAAkAgBCgCACIFLwGSA0ELTwRAIANBGGoiFCAEKAIIEDggAyAJNgIMIAMgBTYCCCADIAMoAhg2AhAgA0EgaigCACEbIAMoAhwhFSMAQTBrIgwkACADQQhqIgQoAgAiFi8BkgMhEBBLIgVBADsBkgMgBUEANgKIAiAMQQhqIAQgBRAQIAUvAZIDIglBAWohEQJAAkAgCUEMSQRAIBEgECAEKAIIIhBrIhNHDQEgBUGYA2ogEEECdCAWakGcA2ogE0ECdBB4IRAgBCgCBCERQQAhBANAAkAgECAEQQJ0aigCACITIAQ7AZADIBMgBTYCiAIgBCAJTw0AIAQgBCAJSWoiBCAJTQ0BCwsgFCAMQQhqQSgQeCIEQTRqIBE2AgAgBCAFNgIwIARBLGogETYCACAEIBY2AiggDEEwaiQADAILIBFBDEH0j8AAECwAC0Gsj8AAQShB1I/AABBAAAsgAyAbNgJYIAMgA0HMAGogA0HEAGogFRsoAgA2AlQgAyADQcgAaiADQUBrIBUbKAIANgJQIANB0ABqIA4gDyAGEAsgDSAUQTgQeBoMAQsgBCAOIA8gBhALIA1BBjoAEAsgA0HgAGokAAwBC0GEkMAAQTVBvJDAABBAAAsgAi0AOEEGRg0CIAIgAkEoakEoEHgiAygCXCEFIAMoAlghBiADKAJUIQQgAygCUCIDKAKIAiIJDQALCyACQShqIAJBKBB4GiAXKAIAIgQoAgAiCUUNASAEKAIEIQgQSyIDIAk2ApgDIANBADsBkgMgA0EANgKIAiAEIAhBAWoiCDYCBCAEIAM2AgAgCUEAOwGQAyAJIAM2AogCIAIgCDYChAEgAiADNgKAASACQZABaiACQQhqKAIANgIAIAIgAikDADcDiAEgAkGoAWogAkHIAGopAwA3AwAgAkGgAWogAkFAaykDADcDACACIAIpAzg3A5gBIAJBiAFqIQkgAkGYAWohAwJAIAUgAkGAAWoiAigCBEEBa0YEQCACKAIAIgIvAZIDIgRBCk0NAUHgjcAAQSBB3I7AABBAAAtB7I7AAEEwQZyPwAAQQAALIAIgBEEBaiIFOwGSAyACIARBDGxqIghBlAJqIAlBCGooAgA2AgAgCEGMAmogCSkCADcCACACIARBGGxqIgQgAykDADcDACAEQQhqIANBCGopAwA3AwAgBEEQaiADQRBqKQMANwMAIAIgBUECdGpBmANqIAY2AgAgBiAFOwGQAyAGIAI2AogCCyASIBg2AgggEiAZNgIEIBIgGjYCACALQbABaiQADAELQcKMwABBK0HQjcAAEEAACyABKAIgGiABKAIoGiAKKAIMIgIgAigCCEEBajYCCAsgAUEwaiQAIABBBjoAAAwBCyAHKAIEIAdBDGooAgBBGGxqIgEpAwAhHCABIAMpAwA3AwAgACAcNwMAIAFBCGoiAikDACEcIAIgA0EIaikDADcDACAAQQhqIBw3AwAgAUEQaiIBKQMAIRwgASADQRBqKQMANwMAIABBEGogHDcDAAsgB0FAayQAC8oBAQJ/IwBBIGsiAyQAAkACQCABIAEgAmoiAUsNAEEIIABBBGooAgAiAkEBdCIEIAEgASAESRsiASABQQhNGyIEQX9zQR92IQECQCACBEAgAyACNgIYIANBATYCFCADIAAoAgA2AhAMAQsgA0EANgIUCyADIAEgBCADQRBqECEgAygCBCEBIAMoAgBFBEAgACABNgIAIABBBGogBDYCAAwCCyABQYGAgIB4Rg0BIAFFDQAgASADQQhqKAIAEHYACxBGAAsgA0EgaiQAC/0BAQJ/IwBBIGsiBSQAQcSQwQBBxJDBACgCACIGQQFqNgIAAkACQCAGQQBIDQBBkJTBAC0AAA0AQZCUwQBBAToAAEGMlMEAQYyUwQAoAgBBAWo2AgAgBSACNgIUIAVBpPLAADYCDCAFQajqwAA2AgggBSAEOgAYIAUgAzYCEEG0kMEAKAIAIgJBAEgNAEG0kMEAIAJBAWo2AgBBtJDBAEG8kMEAKAIABH8gBSAAIAEoAhARAQAgBSAFKQMANwMIQbyQwQAoAgAgBUEIakHAkMEAKAIAKAIUEQEAQbSQwQAoAgBBAWsFIAILNgIAQZCUwQBBADoAACAEDQELAAsAC8EDAQV/AkAgAEKAgICAEFQEQCABIQMMAQsgAUEIayIDIAAgAEKAwtcvgCIAQoC+qNAPfnynIgJBkM4AbiIEQZDOAHAiBUHkAG4iBkEBdEGY6MAAai8AADsAACABQQRrIAIgBEGQzgBsayICQf//A3FB5ABuIgRBAXRBmOjAAGovAAA7AAAgAUEGayAFIAZB5ABsa0H//wNxQQF0QZjowABqLwAAOwAAIAFBAmsgAiAEQeQAbGtB//8DcUEBdEGY6MAAai8AADsAAAsgAyECAkAgAKciAUGQzgBJBEAgASEDDAELIAJBBGshAgNAIAIgAUGQzgBuIgNB8LF/bCABaiIEQeQAbiIFQQF0QZjowABqLwAAOwAAIAJBAmogBCAFQeQAbGtBAXRBmOjAAGovAAA7AAAgAkEEayECIAFB/8HXL0sgAyEBDQALIAJBBGohAgsCQCADQeMATQRAIAMhAQwBCyACQQJrIgIgAyADQf//A3FB5ABuIgFB5ABsa0H//wNxQQF0QZjowABqLwAAOwAACwJAIAFBCU0EQCACQQFrIAFBMGo6AAAMAQsgAkECayABQQF0QZjowABqLwAAOwAACwvfAgEDfyMAQSBrIgQkAAJAIAIgA2oiAyACSQ0AQQggASgCBCIFQQF0IgIgAyACIANLGyICIAJBCE0bIgNBf3NBH3YhAgJAIAUEQCAEIAU2AhggBEEBNgIUIAQgASgCADYCEAwBCyAEQQA2AhQLIARBEGohBiAEAn8CQAJ/AkACQCACBEAgA0EASA0BIAYoAgQEQCAGQQhqKAIAIgUEQCAGKAIAIAUgAiADEFgMBQsLIANFDQJBjZDBAC0AABogAyACEF8MAwsgBEEANgIEIARBCGogAzYCAAwDCyAEQQA2AgQMAgsgAgsiBQRAIAQgBTYCBCAEQQhqIAM2AgBBAAwCCyAEIAI2AgQgBEEIaiADNgIAC0EBCzYCACAEKAIEIQUgBCgCAARAIARBCGooAgAhAwwBCyABIAM2AgQgASAFNgIAQYGAgIB4IQULIAAgAzYCBCAAIAU2AgAgBEEgaiQAC/ABAQx/IAEoAgAiAy8BkgMiC0EMbCEBQX8hBCADQYwCaiEFIAIoAgghBiACKAIAIQxBASEJAkADQCABRQRAIAshBAwCCyAFKAIIIQcgBSgCACEDIARBAWohBCABQQxrIQEgBUEMaiEFIAwhAkEAIQgCQCAGIAcgBiAHSRsiCkUNAANAIAItAAAiDSADLQAAIg5GBEAgAkEBaiECIANBAWohAyAKQQFrIgoNAQwCCwsgDSAOayEIC0F/IAggBiAHayAIGyICQQBHIAJBAEgbIgJBAUYNAAsgAkH/AXENAEEAIQkLIAAgBDYCBCAAIAk2AgALrAEBAX8CQAJAIAEEQCACQQBIDQECfyADKAIEBEACQCADQQhqKAIAIgRFBEAMAQsgAygCACAEIAEgAhBYDAILCyABIAJFDQAaQY2QwQAtAAAaIAIgARBfCyIDBEAgACADNgIEIABBCGogAjYCACAAQQA2AgAPCyAAIAE2AgQgAEEIaiACNgIADAILIABBADYCBCAAQQhqIAI2AgAMAQsgAEEANgIECyAAQQE2AgAL+wQCC38CfiMAQRBrIgUkAAJAAkACQAJAIAEoAiAiA0UEQCABKAIAIAFBADYCAEUNAyABKAIMIQQgASgCCCEDIAEoAgQiAUUEQCAERQ0CA0AgAygCmAMhAyAEQQFrIgQNAAsMAgsgAyECDAILIAEgA0EBazYCICABEC8iCARAIwBBQGoiAiQAIAJBIGogCEEIaiIMKAIANgIAIAIgCCkCADcDGCACQShqIQQjAEEQayIGJAAgAkEYaiIBKAIEIQcCQAJAIAEoAggiCSABKAIAIgEvAZIDSQ0AA0ACQCAGIAEgBxA7IAYoAgAiAUUNACAGKAIEIQcgBigCCCIJIAEvAZIDTw0BDAILCyAEQQA2AgAMAQsgCUEBaiEKAkAgB0UEQCABIQMMAQsgASAKQQJ0akGYA2ooAgAhA0EAIQogB0EBayILRQ0AA0AgAygCmAMhAyALQQFrIgsNAAsLIAQgCTYCFCAEIAc2AhAgBCABNgIMIAQgCjYCCCAEQQA2AgQgBCADNgIACyAGQRBqJAAgAigCKEUEQEHCjMAAQStBrJHAABBAAAsgAkEQaiACQThqKQMANwMAIAJBCGogAkEwaikDACINNwMAIAIgAikDKCIONwMAIAwgDT4CACAIIA43AgAgACACKQIMNwIAIABBCGogAkEUaigCADYCACACQUBrJAAMBAtBzJHAAEErQYCTwAAQQAALQQAhBCADIQELIAUgBDYCCCAFIAI2AgQgBSABNgIAIwBBEGsiASQAIAEgBSgCACAFKAIEEDsDQCABKAIAIgMEQCABIAMgASgCBBA7DAELCyABQRBqJAALIABBADYCAAsgBUEQaiQAC5QBAQJ/IABBAE4EfyAABSABQS06AAAgAUEBaiEBQQAgAGsLIgJB4wBMBEAgAkEJTARAIAEgAkEwajoAACAAQR92QQFqDwsgASACQQF0QZjowABqLwAAOwAAIABBH3ZBAnIPCyABIAJB5ABuIgNBMGo6AAAgASACIANB5ABsa0EBdEGY6MAAai8AADsAASAAQR92QQNqC5YBAgN/AX4jAEEgayICJAAgAUEEaiEDIAEoAgRFBEAgASgCACEBIAJBGGoiBEEANgIAIAJCATcDECACIAJBEGo2AhwgAkEcakGQ6sAAIAEQCBogAkEIaiAEKAIAIgE2AgAgAiACKQMQIgU3AwAgA0EIaiABNgIAIAMgBTcCAAsgAEHc8cAANgIEIAAgAzYCACACQSBqJAALdwEDfwJAAkACQAJAIAAtAAAOBQMDAwECAAsgAEEEahAoDAILIABBCGooAgBFDQEgACgCBBACDwsgACgCBCECIABBDGooAgAiAwRAIAIhAQNAIAEQJSABQRhqIQEgA0EBayIDDQALCyAAQQhqKAIARQ0AIAIQAgsLfQEBfyMAQUBqIgUkACAFIAE2AgwgBSAANgIIIAUgAzYCFCAFIAI2AhAgBUEkakICNwIAIAVBPGpBIjYCACAFQQI2AhwgBUGA98AANgIYIAVBIzYCNCAFIAVBMGo2AiAgBSAFQRBqNgI4IAUgBUEIajYCMCAFQRhqIAQQRwALYgEEfiAAIAJC/////w+DIgMgAUL/////D4MiBH4iBSADIAFCIIgiBn4iAyAEIAJCIIgiAn58IgFCIIZ8IgQ3AwAgACAEIAVUrSACIAZ+IAEgA1StQiCGIAFCIIiEfHw3AwgL3gEBBX8jAEEwayIBJAACfyAAKAIAIgJFBEBBACEAQQAMAQsgASACNgIgIAFBADYCHCABIAI2AhAgAUEANgIMIAEgACgCBCICNgIkIAEgAjYCFCAAKAIIIQBBAQshAiABIAA2AiggASACNgIYIAEgAjYCCCMAQRBrIgAkACAAIAFBCGoiAxAiIAAoAgAiAgRAA0AgAiAAKAIIIgRBDGxqIgVBkAJqKAIABEAgBUGMAmooAgAQAgsgAiAEQRhsahAlIAAgAxAiIAAoAgAiAg0ACwsgAEEQaiQAIAFBMGokAAtwAQN/AkACQAJAIAAoAgAiACgCAA4CAAECCyAAQQhqKAIARQ0BIAAoAgQQAgwBCyAALQAEQQNHDQAgAEEIaigCACIBKAIAIgMgASgCBCICKAIAEQUAIAIoAgQEQCACKAIIGiADEAILIAEQAgsgABACC2wBAX8jAEEwayIDJAAgAyABNgIEIAMgADYCACADQRRqQgI3AgAgA0EsakEDNgIAIANBAjYCDCADQez2wAA2AgggA0EDNgIkIAMgA0EgajYCECADIAM2AiggAyADQQRqNgIgIANBCGogAhBHAAtsAQF/IwBBMGsiAyQAIAMgADYCACADIAE2AgQgA0EUakICNwIAIANBLGpBAzYCACADQQI2AgwgA0Hc+cAANgIIIANBAzYCJCADIANBIGo2AhAgAyADQQRqNgIoIAMgAzYCICADQQhqIAIQRwALbAEBfyMAQTBrIgMkACADIAA2AgAgAyABNgIEIANBFGpCAjcCACADQSxqQQM2AgAgA0ECNgIMIANB/PnAADYCCCADQQM2AiQgAyADQSBqNgIQIAMgA0EEajYCKCADIAM2AiAgA0EIaiACEEcAC2wBAX8jAEEwayIDJAAgAyAANgIAIAMgATYCBCADQRRqQgI3AgAgA0EsakEDNgIAIANBAjYCDCADQbD6wAA2AgggA0EDNgIkIAMgA0EgajYCECADIANBBGo2AiggAyADNgIgIANBCGogAhBHAAvSAgEHfyMAQRBrIgYkAAJAIAEoAiAiAkUEQEEAIQEMAQsgASACQQFrNgIgIAEQLyIEBEAgBkEIaiEIIAQoAgQhAwJAAkACQCAEKAIIIgUgBCgCACIBLwGSA0kEQCABIQIMAQsDQCABKAKIAiICRQ0CIANBAWohAyABLwGQAyEFIAUgAiIBLwGSA08NAAsLIAVBAWohBwJAIANFBEAgAiEBDAELIAIgB0ECdGpBmANqKAIAIQFBACEHIANBAWsiA0UNAANAIAEoApgDIQEgA0EBayIDDQALCyAEIAc2AgggBEEANgIEIAQgATYCACAIIAIgBUEYbGo2AgQgCCACIAVBDGxqQYwCajYCAAwBC0HCjMAAQStBvJHAABBAAAsgBigCDCECIAYoAgghAQwBC0HMkcAAQStB2JLAABBAAAsgACACNgIEIAAgATYCACAGQRBqJAALagEDfyAAQQRqIQICQCAAKAIAIgEEQCAAKAIERQ0BCyACQQAgARsPCyAAQQhqKAIAIQEgAEEMaigCACIDBEADQCABKAKYAyEBIANBAWsiAw0ACwsgAEIANwIIIAAgATYCBCAAQQE2AgAgAgtuAQN/IwBBIGsiAiQAAn9BASAAIAEQEQ0AGiABQRhqKAIAIQMgASgCFCEEIAJCADcCFCACQdj1wAA2AhAgAkEBNgIMIAJBoPbAADYCCEEBIAQgAyACQQhqEAgNABogAEEEaiABEBELIAJBIGokAAtdAQF/IwBBIGsiAiQAIAAoAgAhACACQRhqIAFBEGopAgA3AwAgAkEQaiABQQhqKQIANwMAIAIgASkCADcDCCACIAA2AgQgAkEEakHoksAAIAJBCGoQCCACQSBqJAALXQEBfyMAQSBrIgIkACAAKAIAIQAgAkEYaiABQRBqKQIANwMAIAJBEGogAUEIaikCADcDACACIAEpAgA3AwggAiAANgIEIAJBBGpBkOrAACACQQhqEAggAkEgaiQAC1YBAn8jAEEgayICJAAgAEEYaigCACEDIAAoAhQgAkEYaiABQRBqKQIANwMAIAJBEGogAUEIaikCADcDACACIAEpAgA3AwggAyACQQhqEAggAkEgaiQAC1ABAn8CQAJAAkAgAUUEQEEBIQIMAQsgAUEATiIDRQ0BQY2QwQAtAAAaIAEgAxBfIgJFDQILIAAgATYCBCAAIAI2AgAPCxBGAAsgAyABEHYAC2cAIwBBMGsiACQAQYyQwQAtAAAEQCAAQRRqQgE3AgAgAEECNgIMIABB6PDAADYCCCAAQQM2AiQgACABNgIsIAAgAEEgajYCECAAIABBLGo2AiAgAEEIakGQ8cAAEEcACyAAQTBqJAALVgEBfyMAQSBrIgIkACACIAA2AgQgAkEYaiABQRBqKQIANwMAIAJBEGogAUEIaikCADcDACACIAEpAgA3AwggAkEEakHoksAAIAJBCGoQCCACQSBqJAALVgEBfgJAIANBwABxRQRAIANFDQEgAkEAIANrQT9xrYYgASADQT9xrSIEiIQhASACIASIIQIMAQsgAiADQT9xrYghAUIAIQILIAAgATcDACAAIAI3AwgLWwECf0EEIQICQCABQQVJDQAgASECAkACQCABQQVrDgICAQALIAFBB2shAUEBIQNBBiECDAELQQAhAUEBIQNBBSECCyAAIAM2AgQgACACNgIAIABBCGogATYCAAv0BAEOfyMAQRBrIggkACABKAIAIgxBuIHAAEEBEGIgCEEIaiENIwBBEGsiBiQAIAJBAWshDiADQX9zIQ8gAiADaiEQIAEoAgAhCSACIQoDQAJAIAUhAUEAIQQCQAJAAkADQCAQIAQgCmoiBUYEQCABIANHBEAgCSABIAJqIAEEfyABIANPDQQgASACaiwAAEG/f0wNBCADIAFrBSADCxBiCyANQQQ6AAAgBkEQaiQADAULIARBAWohBCAFLQAAIgtBworAAGotAAAiB0UNAAsgASAEaiIFQQFrIhEgAU0NAgJAIAFFDQAgASADTwRAIAEgA0YNAQwDCyABIAJqLAAAQUBIDQILAkAgAyARTQRAIAUgD2oNAwwBCyABIA5qIARqLAAAQb9/TA0CCyAJIAEgAmogBEEBaxBiDAILIAIgAyABIANBmIHAABBeAAsgAiADIAEgASAEakEBa0GogcAAEF4ACyAEIApqIQogCQJ/AkACQAJAAkACQCAHQe0ATQRAAkACQAJAIAdB4gBrDgUCBAQEAQALQcaBwAAgB0EiRg0IGiAHQdwARw0DQciBwAAMCAtBzIHAAAwHC0HKgcAADAYLIAdB7gBrDggEAAAAAwACAQALQYCAwABBKEGIgcAAEEAACyAGQdzqwYEDNgAKIAYgC0EPcUGwisAAai0AADoADyAGIAtBBHZBsIrAAGotAAA6AA4gCSAGQQpqQQYQYgwFC0HSgcAADAILQdCBwAAMAQtBzoHAAAtBAhBiDAELCwJAIAgtAAhBBEYEQCAMQbiBwABBARBiIABBBDoAAAwBCyAAIAgpAwg3AgALIAhBEGokAAuGAgEGfyMAQRBrIgQkAAJAAkAgACgCCCICIAAoAgRPDQAgBEEIaiEFIwBBIGsiASQAAkAgAiAAKAIEIgNNBEACf0GBgICAeCADRQ0AGiAAKAIAIQYCQCACRQRAQQEhAyAGEAIMAQtBASAGIANBASACEFgiA0UNARoLIAAgAjYCBCAAIAM2AgBBgYCAgHgLIQAgBSACNgIEIAUgADYCACABQSBqJAAMAQsgAUEUakIANwIAIAFBATYCDCABQaCDwAA2AgggAUH8gsAANgIQIAFBCGpB9IPAABBHAAsgBCgCCCIAQYGAgIB4Rg0AIABFDQEgACAEKAIMEHYACyAEQRBqJAAPCxBGAAtAAQN/IAEhAyACIQQgASgCiAIiBQRAIAEvAZADIQQgAkEBaiEDCyABEAIgACAFNgIAIAAgA60gBK1CIIaENwIEC0gBAX8jAEEQayICJAAgAkEIaiAAIAFBARAfAkAgAigCCCIAQYGAgIB4RwRAIABFDQEgACACKAIMEHYACyACQRBqJAAPCxBGAAuEAQECfyACIAFrIgQgACgCBCAAKAIIIgJrSwRAIwBBEGsiAyQAIANBCGogACACIAQQHwJAAkAgAygCCCICQYGAgIB4RwRAIAJFDQEgAiADKAIMEHYACyADQRBqJAAMAQsQRgALIAAoAgghAgsgACgCACACaiABIAQQeBogACACIARqNgIIC0YBAX8gAiAAKAIAIgAoAgQgACgCCCIDa0sEQCAAIAMgAhAcIAAoAgghAwsgACgCACADaiABIAIQeBogACACIANqNgIIQQALTQECf0GNkMEALQAAGiABKAIEIQIgASgCACEDQQhBBBBfIgFFBEBBBEEIEHYACyABIAI2AgQgASADNgIAIABB7PHAADYCBCAAIAE2AgALRwEBfyMAQSBrIgMkACADQQxqQgA3AgAgA0EBNgIEIANB2PXAADYCCCADIAE2AhwgAyAANgIYIAMgA0EYajYCACADIAIQRwALRQEBfyMAQRBrIgMkACADIAI2AgggAyACNgIEIAMgATYCACADEDogAygCACEBIAAgAygCCDYCBCAAIAE2AgAgA0EQaiQACzcAAkAgAWlBAUdBgICAgHggAWsgAElyDQAgAARAQY2QwQAtAAAaIAAgARBfIgFFDQELIAEPCwALaQECfwJAAkACQAJAIAAtAAAOBQEBAQIDAAsgAEEEahAoCw8LIABBBGoQUQ8LIABBBGoiACgCCCICBEAgACgCACEBA0AgARAlIAFBGGohASACQQFrIgINAAsLIAAoAgQEQCAAKAIAEAILCzkAAkACfyACQYCAxABHBEBBASAAIAIgASgCEBEAAA0BGgsgAw0BQQALDwsgACADIAQgASgCDBECAAs+AQF+QY2QwQAtAAAaIAApAgAhAUEUQQQQXyIARQRAQQRBFBB2AAsgAEIANwIMIAAgATcCBCAAQQE2AgAgAAs/AQF/IwBBIGsiACQAIABBFGpCADcCACAAQQE2AgwgAEHA9cAANgIIIABBkPXAADYCECAAQQhqQcj1wAAQRwALugIBAn8jAEEgayICJAAgAiAANgIUIAJBqPbAADYCDCACQdj1wAA2AgggAkEBOgAYIAIgATYCECMAQRBrIgAkAAJAIAJBCGoiASgCCCICBEAgASgCDCIDRQ0BIAAgAjYCCCAAIAE2AgQgACADNgIAIwBBEGsiASQAIAAoAgAiAkEMaigCACEDAkACfwJAAkAgAigCBA4CAAEDCyADDQJBACECQajqwAAMAQsgAw0BIAIoAgAiAygCBCECIAMoAgALIQMgASACNgIEIAEgAzYCACABQfzxwAAgACgCBCIBKAIMIAAoAgggAS0AEBAdAAsgAUEANgIEIAEgAjYCACABQZDywAAgACgCBCIBKAIMIAAoAgggAS0AEBAdAAtB4+nAAEErQbzxwAAQQAALQePpwABBK0HM8cAAEEAACy0AAkAgA2lBAUdBgICAgHggA2sgAUlyRQRAIAAgASADIAIQWCIADQELAAsgAAsnACAAIAAoAgRBAXEgAXJBAnI2AgQgACABaiIAIAAoAgRBAXI2AgQLIAEBfwJAIAAoAgQiAUUNACAAQQhqKAIARQ0AIAEQAgsLJAEBf0GNkMEALQAAGkHIA0EIEF8iAARAIAAPC0EIQcgDEHYACyQBAX9BjZDBAC0AABpBmANBCBBfIgAEQCAADwtBCEGYAxB2AAsjACACIAIoAgRBfnE2AgQgACABQQFyNgIEIAAgAWogATYCAAseACAAKAIAIgCtQgAgAKx9IABBAE4iABsgACABEA8LHgAgACABQQNyNgIEIAAgAWoiACAAKAIEQQFyNgIECxQAIABBBGooAgAEQCAAKAIAEAILCxEAIAAoAgQEQCAAKAIAEAILCxkBAX8gACgCECIBBH8gAQUgAEEUaigCAAsLEgBBGSAAQQF2a0EAIABBH0cbCxYAIAAgAUEBcjYCBCAAIAFqIAE2AgALGQAgACgCFCABIAIgAEEYaigCACgCDBECAAscACABKAIUQaiJwQBBBSABQRhqKAIAKAIMEQIACxAAIAAgAWpBAWtBACABa3ELgQYBBn8CfyAAIQUCQAJAAkACQAJAIAJBCU8EQCACIAMQCiIHDQFBAAwGC0EIQQgQVyEAQRRBCBBXIQFBEEEIEFchAkEAQRBBCBBXQQJ0ayIEQYCAfCACIAAgAWpqa0F3cUEDayIAIAAgBEsbIANNDQNBECADQQRqQRBBCBBXQQVrIANLG0EIEFchAiAFEH4iACAAEHIiBBB7IQECQAJAAkACQAJAAkAgABBsRQRAIAIgBE0NBCABQfSTwQAoAgBGDQYgAUHwk8EAKAIARg0DIAEQaQ0JIAEQciIGIARqIgggAkkNCSAIIAJrIQQgBkGAAkkNASABEBIMAgsgABByIQEgAkGAAkkNCCABIAJrQYGACEkgAkEEaiABTXENBCABIAAoAgAiAWpBEGohBCACQR9qQYCABBBXIQIMCAsgAUEMaigCACIJIAFBCGooAgAiAUcEQCABIAk2AgwgCSABNgIIDAELQeCTwQBB4JPBACgCAEF+IAZBA3Z3cTYCAAtBEEEIEFcgBE0EQCAAIAIQeyEBIAAgAhBJIAEgBBBJIAEgBBAJIAANCQwHCyAAIAgQSSAADQgMBgtB6JPBACgCACAEaiIEIAJJDQUCQEEQQQgQVyAEIAJrIgFLBEAgACAEEElBACEBQQAhBAwBCyAAIAIQeyIEIAEQeyEGIAAgAhBJIAQgARBUIAYgBigCBEF+cTYCBAtB8JPBACAENgIAQeiTwQAgATYCACAADQcMBQtBEEEIEFcgBCACayIBSw0AIAAgAhB7IQQgACACEEkgBCABEEkgBCABEAkLIAANBQwDC0Hsk8EAKAIAIARqIgQgAksNAQwCCyAHIAUgASADIAEgA0kbEHgaIAUQAgwCCyAAIAIQeyEBIAAgAhBJIAEgBCACayICQQFyNgIEQeyTwQAgAjYCAEH0k8EAIAE2AgAgAA0CCyADEAEiAUUNACABIAUgABByQXhBfCAAEGwbaiIAIAMgACADSRsQeCAFEAIMAgsgBwwBCyAAEGwaIAAQfQsLCwAgAQRAIAAQAgsLEgAgACgCACABIAEgAmoQPUEACw8AIABBAXQiAEEAIABrcgsPACAAIAEgASACahA9QQALFAAgACgCACABIAAoAgQoAgwRAAALjgkBBX8jAEHwAGsiBSQAIAUgAzYCDCAFIAI2AggCQAJAIAFBgQJPBEACf0GAAiAALACAAkG/f0oNABpB/wEgACwA/wFBv39KDQAaQf4BIAAsAP4BQb9/Sg0AGkH9AQsiBiAAaiwAAEG/f0wNASAFIAY2AhQgBSAANgIQQQUhB0HA+sAAIQYMAgsgBSABNgIUIAUgADYCEEHY9cAAIQYMAQsgACABQQAgBiAEEF4ACyAFIAc2AhwgBSAGNgIYAkACQAJAAkAgASACSSIHIAEgA0lyRQRAAn8CQAJAIAIgA00EQAJAAkAgAkUNACABIAJNBEAgASACRg0BDAILIAAgAmosAABBQEgNAQsgAyECCyAFIAI2AiAgAiABIgNJBEAgAkEBaiIHIAJBA2siA0EAIAIgA08bIgNJDQYCQCADIAdGDQAgACAHaiAAIANqIghrIQcgACACaiIJLAAAQb9/SgRAIAdBAWshBgwBCyACIANGDQAgCUEBayICLAAAQb9/SgRAIAdBAmshBgwBCyACIAhGDQAgAkEBayICLAAAQb9/SgRAIAdBA2shBgwBCyACIAhGDQAgAkEBayICLAAAQb9/SgRAIAdBBGshBgwBCyACIAhGDQAgB0EFayEGCyADIAZqIQMLIAMEfwJAIAEgA00EQCABIANGDQEMCgsgACADaiwAAEG/f0wNCQsgASADawUgAQtFDQYCQCAAIANqIgEsAAAiAEEASARAIAEtAAFBP3EhBiAAQR9xIQIgAEFfSw0BIAJBBnQgBnIhAgwECyAFIABB/wFxNgIkQQEMBAsgAS0AAkE/cSAGQQZ0ciEGIABBcE8NASAGIAJBDHRyIQIMAgsgBUHkAGpBIzYCACAFQdwAakEjNgIAIAVB1ABqQQM2AgAgBUE8akIENwIAIAVBBDYCNCAFQdD7wAA2AjAgBUEDNgJMIAUgBUHIAGo2AjggBSAFQRhqNgJgIAUgBUEQajYCWCAFIAVBDGo2AlAgBSAFQQhqNgJIDAcLIAJBEnRBgIDwAHEgAS0AA0E/cSAGQQZ0cnIiAkGAgMQARg0ECyAFIAI2AiRBASACQYABSQ0AGkECIAJBgBBJDQAaQQNBBCACQYCABEkbCyEAIAUgAzYCKCAFIAAgA2o2AiwgBUE8akIFNwIAIAVB7ABqQSM2AgAgBUHkAGpBIzYCACAFQdwAakEkNgIAIAVB1ABqQSU2AgAgBUEFNgI0IAVBhPvAADYCMCAFQQM2AkwgBSAFQcgAajYCOCAFIAVBGGo2AmggBSAFQRBqNgJgIAUgBUEoajYCWCAFIAVBJGo2AlAgBSAFQSBqNgJIDAQLIAUgAiADIAcbNgIoIAVBPGpCAzcCACAFQdwAakEjNgIAIAVB1ABqQSM2AgAgBUEDNgI0IAVBiPzAADYCMCAFQQM2AkwgBSAFQcgAajYCOCAFIAVBGGo2AlggBSAFQRBqNgJQIAUgBUEoajYCSAwDCyADIAdBvPzAABAtAAtB2PXAAEErIAQQQAALIAAgASADIAEgBBBeAAsgBUEwaiAEEEcACxkAAn8gAUEJTwRAIAEgABAKDAELIAAQAQsL7gYCD38BfgJ/IAAoAgAhByAAKAIIIQMjAEEgayICJABBASENAkACQCABKAIUIgpBIiABQRhqKAIAIg4oAhAiCxEAAA0AAkAgA0UEQEEAIQFBACEDDAELIAMgB2ohD0EAIQEgByEAAkACQANAAkAgACIJLAAAIgRBAE4EQCAJQQFqIQAgBEH/AXEhBQwBCyAJLQABQT9xIQAgBEEfcSEFIARBX00EQCAFQQZ0IAByIQUgCUECaiEADAELIAktAAJBP3EgAEEGdHIhCCAJQQNqIQAgBEFwSQRAIAggBUEMdHIhBQwBCyAFQRJ0QYCA8ABxIAAtAABBP3EgCEEGdHJyIgVBgIDEAEYNAyAJQQRqIQALIAIgBUGBgAQQBgJAAkAgAi0AAEGAAUYNACACLQALIAItAAprQf8BcUEBRg0AIAEgBksNAwJAIAFFDQAgASADTwRAIAEgA0YNAQwFCyABIAdqLAAAQUBIDQQLAkAgBkUNACADIAZNBEAgAyAGRg0BDAULIAYgB2osAABBv39MDQQLIAogASAHaiAGIAFrIA4oAgwRAgANBiACQRhqIgwgAkEIaigCADYCACACIAIpAwAiETcDEAJAIBGnQf8BcUGAAUYEQEGAASEEA0ACQCAEQYABRwRAIAItABoiCCACLQAbTw0EIAIgCEEBajoAGiAIQQpPDQYgAkEQaiAIai0AACEBDAELQQAhBCAMQQA2AgAgAigCFCEBIAJCADcDEAsgCiABIAsRAABFDQALDAgLQQogAi0AGiIBIAFBCk0bIQggAi0AGyIEIAEgASAESRshDANAIAEgDEYNASACIAFBAWoiBDoAGiABIAhGDQMgAkEQaiABaiEQIAQhASAKIBAtAAAgCxEAAEUNAAsMBwsCf0EBIAVBgAFJDQAaQQIgBUGAEEkNABpBA0EEIAVBgIAESRsLIAZqIQELIAYgCWsgAGohBiAAIA9HDQEMAwsLIAhBCkGYicEAECoACyAHIAMgASAGQZj5wAAQXgALIAFFBEBBACEBDAELAkAgASADTwRAIAEgA0YNAQwECyABIAdqLAAAQb9/TA0DCyADIAFrIQMLIAogASAHaiADIA4oAgwRAgANACAKQSIgCxEAACENCyACQSBqJAAgDQwBCyAHIAMgASADQYj5wAAQXgALCxAAIABBADYCCCAAQQA2AgALDQAgACABIAEgAmoQPQshACAAQraoobu2yeqRJDcDCCAAQr6ctpScpZ64tn83AwALIAAgAELk3seFkNCF3n03AwggAELB9/nozJOy0UE3AwALIQAgAEKl0eXJut/Ojt0ANwMIIABC5aviuKXm/+d4NwMACxAAIAAoAgAgACgCBCABEHcLEAAgACgCACAAKAIIIAEQdwsTACAAQezxwAA2AgQgACABNgIACw0AIAAtAARBAnFBAXYLEAAgASAAKAIAIAAoAgQQBAsKAEEAIABrIABxCwsAIAAtAARBA3FFCwwAIAAgAUEDcjYCBAsNACAAKAIAIAAoAgRqCw4AIAAoAgAaA0AMAAsACw0AIAA1AgBBASABEA8LCwAgACMAaiQAIwALCgAgACgCBEF4cQsKACAAKAIEQQFxCwoAIAAoAgxBAXELCgAgACgCDEEBdgsZACAAIAFBsJDBACgCACIAQRIgABsRAQAACwoAIAIgACABEAQLuAIBB38CQCACIgRBD00EQCAAIQIMAQsgAEEAIABrQQNxIgNqIQUgAwRAIAAhAiABIQYDQCACIAYtAAA6AAAgBkEBaiEGIAJBAWoiAiAFSQ0ACwsgBSAEIANrIghBfHEiB2ohAgJAIAEgA2oiA0EDcQRAIAdBAEwNASADQQN0IgRBGHEhCSADQXxxIgZBBGohAUEAIARrQRhxIQQgBigCACEGA0AgBSAGIAl2IAEoAgAiBiAEdHI2AgAgAUEEaiEBIAVBBGoiBSACSQ0ACwwBCyAHQQBMDQAgAyEBA0AgBSABKAIANgIAIAFBBGohASAFQQRqIgUgAkkNAAsLIAhBA3EhBCADIAdqIQELIAQEQCACIARqIQMDQCACIAEtAAA6AAAgAUEBaiEBIAJBAWoiAiADSQ0ACwsgAAuaBQEHfwJAAn8CQCACIgMgACABa0sEQCABIANqIQUgACADaiECIAAgA0EPTQ0CGiACQXxxIQBBACACQQNxIgZrIQcgBgRAIAEgA2pBAWshBANAIAJBAWsiAiAELQAAOgAAIARBAWshBCAAIAJJDQALCyAAIAMgBmsiBkF8cSIDayECQQAgA2shAyAFIAdqIgVBA3EEQCADQQBODQIgBUEDdCIEQRhxIQcgBUF8cSIIQQRrIQFBACAEa0EYcSEJIAgoAgAhBANAIABBBGsiACAEIAl0IAEoAgAiBCAHdnI2AgAgAUEEayEBIAAgAksNAAsMAgsgA0EATg0BIAEgBmpBBGshAQNAIABBBGsiACABKAIANgIAIAFBBGshASAAIAJLDQALDAELAkAgA0EPTQRAIAAhAgwBCyAAQQAgAGtBA3EiBWohBCAFBEAgACECIAEhAANAIAIgAC0AADoAACAAQQFqIQAgAkEBaiICIARJDQALCyAEIAMgBWsiA0F8cSIGaiECAkAgASAFaiIFQQNxBEAgBkEATA0BIAVBA3QiAEEYcSEHIAVBfHEiCEEEaiEBQQAgAGtBGHEhCSAIKAIAIQADQCAEIAAgB3YgASgCACIAIAl0cjYCACABQQRqIQEgBEEEaiIEIAJJDQALDAELIAZBAEwNACAFIQEDQCAEIAEoAgA2AgAgAUEEaiEBIARBBGoiBCACSQ0ACwsgA0EDcSEDIAUgBmohAQsgA0UNAiACIANqIQADQCACIAEtAAA6AAAgAUEBaiEBIAJBAWoiAiAASQ0ACwwCCyAGQQNxIgBFDQEgAyAFaiEFIAIgAGsLIQAgBUEBayEBA0AgAkEBayICIAEtAAA6AAAgAUEBayEBIAAgAkkNAAsLC5wBAQJ/IAJBD0sEQCAAQQAgAGtBA3EiA2ohBCADBEADQCAAIAE6AAAgAEEBaiIAIARJDQALCyAEIAIgA2siAkF8cSIDaiEAIANBAEoEQCABQf8BcUGBgoQIbCEDA0AgBCADNgIAIARBBGoiBCAASQ0ACwsgAkEDcSECCyACBEAgACACaiECA0AgACABOgAAIABBAWoiACACSQ0ACwsLBwAgACABagsHACAAIAFrCwcAIABBCGoLBwAgAEEIawsDAAELC+qNARsAQYCAwAAL5QppbnRlcm5hbCBlcnJvcjogZW50ZXJlZCB1bnJlYWNoYWJsZSBjb2RlL1VzZXJzL3N0ZXZlLy5jYXJnby9yZWdpc3RyeS9zcmMvaW5kZXguY3JhdGVzLmlvLTZmMTdkMjJiYmExNTAwMWYvc2VyZGVfanNvbi0xLjAuMTA4L3NyYy9zZXIucnMAKAAQAF8AAAALBgAAEgAAACgAEABfAAAALggAAC4AAAAoABAAXwAAACEIAAA7AAAAImZhbHNldHJ1ZW51bGxcIlxcXGJcZlxuXHJcdGNhbGxlZCBgUmVzdWx0Ojp1bndyYXAoKWAgb24gYW4gYEVycmAgdmFsdWUAAQAAAAQAAAAEAAAAAgAAAAp9W3ssCjogXUhhbmRsaW5nIHJlcXVlc3QgZnJvbSBSdXN0IW1lc3NhZ2VIZWxsbyBmcm9tIFJ1c3QsIGl0IHdvcmtzIXZhbC5yc3JlcXVlc3R1cmxtZXRob2QAVQEQAAYAAAAXAAAAMAAAAFRyaWVkIHRvIHNocmluayB0byBhIGxhcmdlciBjYXBhY2l0eXwBEAAkAAAAL3J1c3RjLzU2ODBmYTE4ZmVhYTg3ZjNmZjA0MDYzODAwYWVjMjU2YzNkNGI0YmUvbGlicmFyeS9hbGxvYy9zcmMvcmF3X3ZlYy5yc6gBEABMAAAArgEAAAkAAAAFAAAADAAAAAQAAAAGAAAABwAAAAgAAABhIERpc3BsYXkgaW1wbGVtZW50YXRpb24gcmV0dXJuZWQgYW4gZXJyb3IgdW5leHBlY3RlZGx5AAkAAAAAAAAAAQAAAAoAAAAvcnVzdGMvNTY4MGZhMThmZWFhODdmM2ZmMDQwNjM4MDBhZWMyNTZjM2Q0YjRiZS9saWJyYXJ5L2FsbG9jL3NyYy9zdHJpbmcucnMAZAIQAEsAAADdCQAADgAAAEVPRiB3aGlsZSBwYXJzaW5nIGEgbGlzdEVPRiB3aGlsZSBwYXJzaW5nIGFuIG9iamVjdEVPRiB3aGlsZSBwYXJzaW5nIGEgc3RyaW5nRU9GIHdoaWxlIHBhcnNpbmcgYSB2YWx1ZWV4cGVjdGVkIGA6YGV4cGVjdGVkIGAsYCBvciBgXWBleHBlY3RlZCBgLGAgb3IgYH1gZXhwZWN0ZWQgaWRlbnRleHBlY3RlZCB2YWx1ZWV4cGVjdGVkIGAiYGludmFsaWQgZXNjYXBlaW52YWxpZCBudW1iZXJudW1iZXIgb3V0IG9mIHJhbmdlaW52YWxpZCB1bmljb2RlIGNvZGUgcG9pbnRjb250cm9sIGNoYXJhY3RlciAoXHUwMDAwLVx1MDAxRikgZm91bmQgd2hpbGUgcGFyc2luZyBhIHN0cmluZ2tleSBtdXN0IGJlIGEgc3RyaW5naW52YWxpZCB2YWx1ZTogZXhwZWN0ZWQga2V5IHRvIGJlIGEgbnVtYmVyIGluIHF1b3Rlc2Zsb2F0IGtleSBtdXN0IGJlIGZpbml0ZSAoZ290IE5hTiBvciArLy1pbmYpbG9uZSBsZWFkaW5nIHN1cnJvZ2F0ZSBpbiBoZXggZXNjYXBldHJhaWxpbmcgY29tbWF0cmFpbGluZyBjaGFyYWN0ZXJzdW5leHBlY3RlZCBlbmQgb2YgaGV4IGVzY2FwZXJlY3Vyc2lvbiBsaW1pdCBleGNlZWRlZEVycm9yKCwgbGluZTogLCBjb2x1bW46ICkAAAD0BBAABgAAAPoEEAAIAAAAAgUQAAoAAAAMBRAAAQAAADAxMjM0NTY3ODlhYmNkZWYgIHV1dXV1dXV1YnRudWZydXV1dXV1dXV1dXV1dXV1dXV1AAAiAEGei8AACwFcAEHCjMAAC5cIY2FsbGVkIGBPcHRpb246OnVud3JhcCgpYCBvbiBhIGBOb25lYCB2YWx1ZS9ydXN0Yy81NjgwZmExOGZlYWE4N2YzZmYwNDA2MzgwMGFlYzI1NmMzZDRiNGJlL2xpYnJhcnkvYWxsb2Mvc3JjL2NvbGxlY3Rpb25zL2J0cmVlL21hcC9lbnRyeS5ycwAAAG0GEABgAAAAcAEAADYAAABhc3NlcnRpb24gZmFpbGVkOiBpZHggPCBDQVBBQ0lUWS9ydXN0Yy81NjgwZmExOGZlYWE4N2YzZmYwNDA2MzgwMGFlYzI1NmMzZDRiNGJlL2xpYnJhcnkvYWxsb2Mvc3JjL2NvbGxlY3Rpb25zL2J0cmVlL25vZGUucnMAAAcQAFsAAACgAgAACQAAAGFzc2VydGlvbiBmYWlsZWQ6IGVkZ2UuaGVpZ2h0ID09IHNlbGYuaGVpZ2h0IC0gMQAHEABbAAAAnAIAAAkAAABhc3NlcnRpb24gZmFpbGVkOiBzcmMubGVuKCkgPT0gZHN0LmxlbigpAAcQAFsAAAAcBwAABQAAAAAHEABbAAAAnAQAABYAAAAABxAAWwAAANwEAAAWAAAAYXNzZXJ0aW9uIGZhaWxlZDogZWRnZS5oZWlnaHQgPT0gc2VsZi5ub2RlLmhlaWdodCAtIDEAAAAABxAAWwAAAN0DAAAJAAAAL3J1c3RjLzU2ODBmYTE4ZmVhYTg3ZjNmZjA0MDYzODAwYWVjMjU2YzNkNGI0YmUvbGlicmFyeS9hbGxvYy9zcmMvY29sbGVjdGlvbnMvYnRyZWUvbmF2aWdhdGUucnMATAgQAF8AAABZAgAAMAAAAEwIEABfAAAAFwIAAC8AAABjYWxsZWQgYE9wdGlvbjo6dW53cmFwKClgIG9uIGEgYE5vbmVgIHZhbHVlL3J1c3RjLzU2ODBmYTE4ZmVhYTg3ZjNmZjA0MDYzODAwYWVjMjU2YzNkNGI0YmUvbGlicmFyeS9hbGxvYy9zcmMvY29sbGVjdGlvbnMvYnRyZWUvbmF2aWdhdGUucnMAAPcIEABfAAAAogAAACQAAAALAAAABAAAAAQAAAAMAAAADQAAAA4AAAD3CBAAXwAAAMcAAAAnAAAAMDAwMTAyMDMwNDA1MDYwNzA4MDkxMDExMTIxMzE0MTUxNjE3MTgxOTIwMjEyMjIzMjQyNTI2MjcyODI5MzAzMTMyMzMzNDM1MzYzNzM4Mzk0MDQxNDI0MzQ0NDU0NjQ3NDg0OTUwNTE1MjUzNTQ1NTU2NTc1ODU5NjA2MTYyNjM2NDY1NjY2NzY4Njk3MDcxNzI3Mzc0NzU3Njc3Nzg3OTgwODE4MjgzODQ4NTg2ODc4ODg5OTA5MTkyOTM5NDk1OTY5Nzk4OTkBAEHnlMAAC9EqIJqZmZmZmZmZmZmZmZmZmRkVrkfhehSuR+F6FK5H4XoU3iQGgZVDi2zn+6nx0k1iEJbUCWgibHh6pSxDHOviNhqrQ26GG/D5YYTwaOOItfgUIjZYOEnzx7Q2je21oPfGEGojjcAOUqaHV0ivvJry1xqIT9dmpUG4n985jDDijnkVB6YSH1EBLeaylNYm6AsuEaQJUcuBaK7Wt7q919nffBvqOqeiNO3x3l+VZHnhf/0Vu8iF6PbwJ38ZEeotgZmXEfgN1kC+tAxlwoF2SWjCJRyTcd4zmJBw6gGbK6GGm4QWQ8F+KeCm8yGbFVbnnq8DEjc1MQ/N14VpK7yJ2Jey0hz5kFo/1983IYmW1EZG9Q4X+nNIzEXmX+egq0PS0V1yEl2GDXo8PWalNKzStk/Jgx2xnteUY5ceUV0jQpIMoZwXwUt53YLfftp9T5sOCrTjEmisW2LRmGQqluVeFxAgOR5T8OKBp+C27kRRshJAsy0YqSZPzlJNklhqp46omcJXE0GkfrC3e1Anqth92vXQ8h40UGXAX8mmUrsTy67EQMIYkKbqmUzU6w7JDzzyNprOE4AKEcOtU3mxQRlgUL72sB9nCHQCi9wtwWdHs6b+XloZUqApNW+wJDSGn8Lr/ktIFNsZ7pDyWR2Qnn9oiWXWORBfKbC0HcP7TJcyp6jVI/YZsrpZXbE1lj2sWx+6d+nEFChi4X0nXquXVklM+5KHnRANnWjJ2Mmr8vAOevi3pZUaPhe6OnqhvFtaci4tk4REFctF+y7IGsqvro6LikKdAxFFCZKxpvfcskrkeKqd+zgbBKFBweuSffVugy1VsS/HFQO0Z2eJdWTEWJxXdycmbBHS7KXY24htbfTGJfILPeAb2yPrRhYHvorDOB4oo/1MFkm2VdIRbP5unGBLU08x1xEOiu+2TxOXsWBnRYUYgoscpaG/+HIPrCcauWo3rQHWFh5OmWDCcla54WBVLCTORBKVFsLNAx5X9TXOuxNt4zodq6sBCwMYrCor2C92ik9iF1aJNG8C4Ly7VRPzxG4MtRKJqO2x0MzHku8euNRKeu4dB7pXjkAK09vyS5MQb/vxFwbI33EA1ah89W8P2lj8JxPWDGbpM7un+rtMsimOYKYeEdeEhyn8UpXJo45UCxqFGA6s0NK6yaiqB4PYdm+unRPjrBoeXtza3aXRwFeysGIfT4pIS0uwSH5RQZqsjsAbGdmh09XVWW3L2s3hVqUzFhR7gdx3EXtXPOLX56vqwhEQKs9gWYJe8sY2JqasqgS2GbulgEdoGPVrxVHrVlWdkRSWhAAG7XkqI9GnIt/dfXQQVgc0o+GP3dGBDNExlvxTGkVs9ugac+SnND2n9ET9DxWeVvhT4igdU12XUl1ql9kQYleNuQPbYesu8lCVEL/1GuhFpMfPSE68WFva3aZlkRUga4Ns2dNxY63i4RcfHkERzRGfrSiGHJ9IBAPzZGObGwvbGL5Ta7DlBp01jx3pFRaiFUfLD4nz6mtKkXLkIKsRN7xxeEzbuERGqhuEbQFFHF9jwcbWFccDBVVJA76anRYZ6c1rRd44Njd3B2n+rhcSwUEWRqJjwVZYWHIOl7HyHM5nq9GBHAHfeRP1cRKOKBel7FVBzhY0f2HckMEO2IYSbkdWNX0kIGUCx+do5IykHSU5ePcwHYDqAWy5IB3XtheE+iz587CZuzQjYU0XrPgSOfdHKFNOXF9UOGgV8qxaHi4s07l1C31/Q2BTRFuKSBhYI9zH99Uwmc8ZqTZ8O20TJtL5coyJtI6yjw7x+SsVH7hBLo+jBypyKKYL9Me83Rj6mr6lTzm7wYYe1lwGl+QT9vcwCRnCXpzXMPD61iTUH/hfWgcUaOVJeY0mL9+Ddhlg5uEFECBRbscKUr/lz14UGoWB0QyA2vEFbw6ZhNlLEPXUaIIUAMRP1uTj9KD1Ehord+0Bqplp2RG3HPez99sUvMWKAYgU7q10krDFXPmvECwJ3mim7XxJVOqAb5Qosxok1ORTuFfKOhBVmr92IFwVg3YdQ2B5O2Jzqq7/XoAWEZ69yNFm9SuduBCxMsszVxt/ZG1BUsS8fWAN9I6iXN8VzLaKZ9tp/crmPcPYTn1/Ed+Kd3LFDy+r1y8FjuQu/xuA1ZJbBHPyiKyMaj4dv2UWZkRCSdAo9dNWPVWYSv/qEaOgA0JNQYi5V5W78xAyqxzp5gJo1805YXl3/MJAW+8WVFICIHlxYect+clozRVZEoZQnZmOtWilfFt2dBVWWx3SpkrhPpEgUf0VxfbdRHwXDh+iGv9ATafKRDeSsdDJEkrLafdkzq4LEW5YUE+0Dx47PO7FUNiLPKfxeXM/kAwYycnxN9p5CcqF9MfCMkA9E9tC6b/2wqipb7oMnrdmyB7jm7rMK89TISaVcH4sUqAYgkmVcIlyqRq43SZl8HSzE511iBoPhHX3jC8+COeHhR8XXqB7cjaRXwommAbsnzcZ3+QZllv4QBnVhEYF8H8sFEzqR6uvxgDhEDcF0YyZIxBH3T9FTKRnzuck1bRHj9IZBrHMndbpUtgft93Dn3KoFDgnCktF7tt5GSx+aRnChhBZ2KkRouNfKY9GMA+PNnEaehO7p4Ecs7qla/PY2F4nFS+pleya4yhiUYmPreBL7BAXde/g9zgOnegOTK+arBMbeSpZGpMt2LBTctYl4lapFS5VR0gPvnmN3MHet4FFVBF8uwvafpaPFZScl4zPCLobly/WFP8Rpnd2sN/Wcm0uFnmM3kP/p1H5kfOyePW9vhGOrf3S/j8cwhzst1oiY2Qc2IpkQjIzsAEX8F8VtbW2Fkaig5uOwlkBrFnm3ZDEKxKjAzlfFwT2zqzCo/wa1BIdg5wtTKxpXnK9mxzKSENCF5zjitaJVBj1/eIWCAdpmxLGBau9D1SN7i9r8QzYdMUdBWsi/nJ2176MIsFwRirRFwS8TssoxRL/1k5njWu7DROg+X14dDtRyyR+2HsSX3weTWH++SnJDQm3Ma38QX9jGAqBy5Qh1NegxSckyjTMghN3znhUz7m/Z28MbUMhrTcf+XEt3aWUzB9ZcIrPTVf5GMf0vX1R3dZ/evOhPz6s+hML7i/J6C6+/8O4nDL9efcf1iTzoCC/MWY2+hbC/ceSGXgdXBoazCe4XvurActsdRRg5Hx7rglTkxjJvGei8F0QmaCUxbBC6x70dJQ/aucvGuHmdgQnAonlXCrdMogf8xTn6yudhc6gt7DusCigf8IQ2N/fYW9KAVm0Sk50M8zQGq1M5ucl1c3gKaI+kI/WcxXx1lGGUXdxTe60y9lyeCkR6Ffp1ui+6HuwVKyPhI11GyATId9TMrr8Wd2JDGqk9xWAQucYQyjIY65KbnDu6ZIRZmrYJzgNDQYXEUoaF0MeHOshrewspD1rEnRuexKcfhZWTle98Bz+iNtcWPxB4/4RI0olYrSUlkFfYY1gNgXLHOnUHegpqqtnf+c9TfjQCBeH3RcguyFWuTK5ZNf5c20SpZWMZitpI8LqwTrywux7HR3e1h6JuoLOuzRiWwJXlhcYGN9LB2I1pfz2tOIBrN4SWfNkediciDuU8Yc3NhMxHuH1g8dGSm383FoGxpFCJxgaKwMGn25XMBevntGnm1ITkN7RPMt9JRolGDEcppLqHkDlpzA8/h1It3la44SouxgAUYbAyTFL08XHroKdU8kTzbSjzULpEVIJphfRyIWoH6SQHD4CIdt0B7jfQDqeUxlQDUrLAbQV9wVgGWf75EIUpwoICZsp3vg3s3pS/IM1ENfdDKiRQjCOWbgqt5M57xkTSwogDgKNPuH57vhCYb8UDzwIgD6bPWXnx1j6mxqZEOQsDQBk+MhupQyOkPmQjhrqI6SZ6fnTi7ejcUBh2j4VuxxQ4bqUqTz5gvSZGhX/ECths5vEunXHjtEgw127MRuJGikWapXE0gsO52ixYsEVoXu6EYh30NtvPh+HJ4JnEZuSXRxAv4As5mOYPj/Q2BtJdeRJM8wzvVG2RmX/DEcW1F1Qbo/Wj8qnXgVRzHDSEVPJs+NLVxlE2f1uTq3ngxypOvaCCXlHA+GXJaWK7M8WuvvEaNRgbM+AeYTqbvA/Eir5Bw6HNHrlmvXTEEsaMx0ilDkLbJAuUeIqQ9oIFVwXtanH1bymi9qBVc/h0xCwEocP2SIucd+QnFXlAlOB5h1sDBRPi1pM2hbeHc+omusXiqOppaJ7o654frGlIOIiE6kFqaJqX9J9J5e1opo2nh5U0SCCiH/blx+s904Vkn4Yd6eAzgZmfHlMI8bY3XSYE/ELAeQKcC2PrWujJ5ZUWh9a1gBQolkkDL7vtR94EBUZFUWa2YEUHXD+8vey+dkQFHdqexSbQxfA/lvGKC57DRDyQ5LtxAXyzMosCg59K68ZwpwOvtA3WwpvvaFxyiKMFM7jPstz+UgIjJe0J9UbcBCwn2R47FsO2qwlVAxV+UwawH9QYPCvPnu9t6nWEGEKFTNmQIDzv8uVlyzu3nMa1RBScM1mUmas71hHsGS5kO4a21mkuA6FIyZHbPO2+qaLFUmutpPY0IIebCMpX5WFPBF1sIof9Bqe/aw4qP7uCJQb91nVsimvsZe9k4aYJQcQFix7d/W6JY6sl9yeEx5sphETxVgiKwl9er8t/rjJeT0cdmqtTu+g/WHMV8tgoZSXFsXuvQtZGv7nCRMJ503dEhI6sfxFW11jptyEDtiv++ocyI0wa69KHIWw0D4T82IiF9TXJrzybuPQJtrLdcLogRKGjKTG6heftNcpRomdp5wda3BQBe/fGCpG7gShF4awF4nz2Z0ls+BUa4udTXme8xJ0UvZib+vNh3hFL3wol1IeXahegr8iC9PGar/JhhJCGOS5S2jMGzwPn4j/OtIOaBNtKXlAeixgGJjamJGD5AwfJCGUM8hWs0YT4hMONh3XGLZNQymgeI843LTcpJFK3xOKr2uoZid/WmAhYaGCqssfor/vueuFMhVNtE20m7tvGU6ZjGGJ0Y6qPZCk9uJiWRQM4dYaoafY7srZtitPgkcQRZskXptyJ34R9orfsQMMGgRJHRhJ9YX+Dfg7GVtp1hTQoEoT1F2ey6T5LxR8h6sQTQERUlPJY986XOa5+QusGnFn2nQPoRwZL7Ae+/pvVhXBUkgq2YCwrSXASy8v8xERNFENqo405xUJzRKyfutPG8QNce4+XR+rbQoPKDKJ2RWdpI2LZRcZvFcIDCAo1HoRlDp8Ejzy9CxZDeDM2bn3G0OVltv89MPw4D2zcOHHXxYDERIWl102WhrL9SaBOeYRBOgc8CT8VpCQ3iILNY+jHNDs44wdMN/ZpkuCol0/6RbaI4M9sVl/4euizk6xMlQSXDk4L7XCy2h50X3kToRTHeMtYL9dNdZTlKdkUHIDdhcci+ZlsSp4qXbstqaOz8QS+kTXb7WqJg/xE4vXfbIHHmJq378qIlI/J0NvrGQoBhhOiH+ZiE7bZR+c8olQIDgTSg3MKHRKxW9lk+oPtDPAHjukCYf2oWpZhA8ic/bCmRiWtgds+OfurTbZtPWRNa4TVlcM4PM/fkkk9boigyJ9H0Ws1kz2/2TU6ZCV6GjoMBnRiXg9+P+DQ+5zRO1TICcUdKGTl8bMnM/xjwPxD00fEFICuSWkR2F/HLMF6H+uyxkPNce36dJNzBZc0ez/8aIU2ZDSXyEPCz0SsNojM1uCEMHnUJloS6thULMqBoUrahpnuUAUuqIiTkBcVWtqvCEVU5QA3ZToTgvNSUS87snnEFHtAMiH2hcSSKnTxkp2DBvavQCgbEhG22yH3GvVkaMVr2TNTL0GBUmKn+Pv3adPEbE64nrICgioQ/845i+mshv0Luj7OaI5U2n/kx7zhCgWXfLsL/u0x3WH/w+y9QO6ES7qR+aRIdkiP/9/tiLTXBzyVAaFQYF6tWX//5HoqLAW9UM4NwEBYsS3MjPbhu0mEu6f8/EBaDY6WYTrkaQVCx2LGfYnm7le++BpvHRQETwX1npehuL6fi/nh2NdQHSWElaR/dbQ95flcdk4Ys2GvR2r2sp4DZN5hMF6Leg90soXVhVvLXFCYdCayIqGMagIEyIiGK9OamhNkdqqPU9AdB7otHnyPohTpNquiGQ/AF0Yh11hKP9s3OmuWG1QzJl9E6SVaA1lrmCp5I1IGnpcLx+DRO09t76zuoNxoK5hsPIYNp2KMSwy9i42wea+51n1E/Bhd4ITHb3kiZvXlz/27h9aTiw1qX3Kg6Gv398y+IsZFaVW9yD+oZzn8rJMwvlvFKodEvmzMRtKuSiPcJuUWRDdlbbB7LVeQ/UN5YDF7SgaSt5eAVde5TXEpB1nBIvtFNWxGAGsfrfEaR1+UtAIvhAitlqbeZcloQ8vMLezp8kagV4VSWGst03ZWPP4wh9uFZtLRAeBI8bXreD1kzXmJBErrNM+mwU9WUk0VoYiPW4bvIncyxWe/eBtwxEFgsrxFWOh428RGP6zJGlBN5s7jhHRm9J/tVljhgd1NSXFxRYcDuMOM5EU6dHSkPdQN554FgscP4/adrp0dQ3GQCwY+hF4xjHlkCT37btIo2fgWcMcLQVbt0AdLIvJ07UfTa4CFyQEfF/NfVZv1A8r5nCLaBIGbcaYSMnwfu2yET1OEnQdn72e4AahwJhXwqf9pA6QF+bKS03SgABHeZvsylCl2RKiRHlIHc4A2I7FrUSBCCkegtAtbRfYMxM/0VedmtMgGM6mJCR5RvaoZaesShV2TRN9pDqgjj29dG+leneIVuIeZFCV5j4xZF2Mt/vFBhK1GLemquvLjbZKcCyW0WsOxBNXpKoSExYkERpH8OgSF6Af3+nuDtxEg9oUbPNTQt9MGYAhv9h8nQLiQyMpQ2h/PRQzgTJ6/X1oTjYcVM+5MjEQuM5QkJXJQEq9xrlLKVHoGcYLp6Z31DMIMdLHb4fauRRrCewexnYpoI0O07/SrpQQ39usZKNXQgBJF7j/HX6HGhnjI+q13wHNoBJgmbExORWutRyIkUzOcE115q0njvoQ4lWUprWt4xqvu3BJDH0qG+h3Q4XEV+l78mKNBz2XuxWH+TUEanmHyY61CgZk32IRccK8BhCPpXXkiHfWbGXRGyc1ymumpbf36dOSq/AdQRYfxKG8Hh7GX+4PD1aNsc0RZdMCYWRjo/8Ws7GJSE98HFHcm01QHOky3yiO1AbZyRYOfUlxc+Mgj7Ig2HYFFDsSfC4PgoUFm37qzVnxO1MrHcq+pQGeN6/L7tdH9C/cVRehmIQ0S/lYCb+sbMOMFqsSAEHHv8AACwEQAEHXv8AACwEUAEHnv8AACwEZAEH2v8AACwJAHwBBhsDAAAsCiBMAQZbAwAALAmoYAEGlwMAACwOAhB4AQbXAwAALA9ASEwBBxcDAAAsDhNcXAEHVwMAACwNlzR0AQeTAwAALBCBfoBIAQfTAwAALBOh2SBcAQYTBwAALBKKUGh0AQZPBwAALBUDlnDASAEGjwcAACwWQHsS8FgBBs8HAAAsFNCb1axwAQcLBwAALBoDgN3nDEQBB0sHAAAsGoNiFVzQWAEHiwcAACwbITmdtwRsAQfLBwAALBj2RYORYEQBBgcLAAAsHQIy1eB2vFQBBkcLAAAsHUO/i1uQaGwBBocLAAAvpTZLVTQbP8BAAAAAAAAAAAID2SuHHAi0VAAAAAAAAAAAgtJ3ZeUN4GgAAAAAAAAAAlJACKCwqixAAAAAAAAAAALk0AzK39K0UAAAAAAAAAEDnAYT+5HHZGQAAAAAAAACIMIESHy/nJxAAAAAAAAAAqnwh1+b64DEUAAAAAAAAgNTb6YygOVk+GQAAAAAAAKDJUiSwCIjvjR8AAAAAAAAEvrMWbgW1tbgTAAAAAAAAha1gnMlGIuOmGAAAAAAAQObYeAN82Oqb0B4AAAAAAOiPhyuCTcdyYUITAAAAAADic2m24iB5z/kSGAAAAACA2tADZBtpV0O4Fx4AAAAAkIhigh6xoRYq084SAAAAALQq+yJmHUqc9IeCFwAAAABh9bmrv6Rcw/EpYx0AAACgXDlUy/fmGRo3+l0SAAAAyLNHKb61YKDgxHj1FgAAALqgmbMt43jIGPbWshwAAEB0BECQ/I1Lfc9Zxu8RAABQkQVQtHtxnlxD8LdrFgAApPUGZKHaDcYzVOylBhwAgIZZhN6kqMhboLSzJ4QRACDobyUWztK6csihoDHlFQAo4suum4GHaY86ygh+XhsAWW0/TQGx9KGZZH7FDhsRQK9Ij6BB3XEKwP3ddtJhFRDbGrMIklQODTB9lRRHuhrqyPBvRdv0KAg+bt1sbLQQJPvsyxYSMjOKzckUiIfhFO056H6clv6/7ED8GWrpGRo0JFHPIR7/95OoPVDiMVAQQW0lQ6rl/vW4Ek3kWj5kFJLI7tMUn34zZ1dgnfFNfRm2euoI2kZeAEFtuARuodwfsoySRUjsOqBIRPPC5OTpE94v91Zap0nIWhWw8x1e5BjW+7TsMBFcerEanHCldR0fZR3xk76KeeyukGFmh2lyE79k7Thu7Zen2vT5P+kDTxjvvSjHyeh9URFy+I/jxGIetXZ5HH6x7tJKR/s5Drv9EmLUl6PdXaqHHRl6yNEpvRd7yX0MVfWU6WSfmDpGdKwd7Z3OJ1UZ/RGfY5/kq8iLEmhFwnGqX3zWhjzH3da6LhfC1jIOlXcbjKgLOZWMafocOcbfKL0qkVdJp0Pd94EcEsi3F3NsdXWtG5GU1HWioxa6pd2Px9LSmGK1uUkTi0wclIfqubzDg59dERQO7NavEXkpZeirtGQHtRWZEafMGxbXc37i1uE9SSJb/9XQv6IbZgiPTSatxm31mL+F4rdFEYDK8uBvWDjJMn8vJ9sllxUgfS/Zi26Ge/9e+/BR7/waNK69ZxcFNK1fG502kxXeEMEZrUFdBoGYN2JEBPiaFRUyYBiS9EehfsV6VQW2AVsaHzxP2/jMJG+7bFXDEeF4ECcLIxI3AO5K6scqNFYZlxTwzavWRICp3eR5NcGr37wZtmArBivwiQovbMFYywsWEOQ4tsc1bCzNOsfxLr6OGxQdx6M5Q4d3gAk5rrptciIZ5LgMCBRpleBLx1kpCQ9rH47zB4WsYV1sjxzYuWXpohNy8EmmF7p0R7MjTii/o4sYj2zcj53oURmgrGHyroyuHtnD6XliMdMP5At9V+0XLRPPNGQYu/3HE91OXK3oXfgXA0J93in9uViUYrPYYnX2HUJJDis6PnS3nB1wx10JuhKS29G1yE1R5QMlTDm1i2gXd1JG4zqhpd5ELp+Hoq5CHYrzC87EhCcL63zDlCWtSRJt8I4B9mXxzSVc9PluGNwWiKzygXO/bUEvc3G4ih6THNWrNzGol+SI/edGsxbz2xHKloU9kr0d6/yhGGDc71IWffzmzPYs5SV8yh5406vnG85dEEAaPK+XjT4TK2TLcBFCdRTQIAub/TAO2DU9/swVkpIZBOnNAT29EU6DzD1AG5v7j6KxICFGFssQ0p8mCBGC+jML3mip19v9lMZHMEoVI/kAjhXDk81SPTq4WbycGrabwHjtWXzAU2YkE7j1oRCjwvDWaHCbsOh/7Rcmc8oUTPOsDINMwtzi3+id7w/9GQ8Y7OfRb/nJ7YuxwvUpPhATHudhxst3POnuXTNztE0UmOVg+re+lYujajUAkCFhGf4e+fhlLntuTMVCAPRpuR9fs5u7//wMxU+7KYA44tMTN6CCqj88ULYjKjSgxtrIGERII5VPS+SjrDRBSHgR+x4rDTa9Ea9u5uvAKC3r6lwTdZCDLNZaCuAm8XL4pSU0GJN0pLeL8QyYcK2Pdg8vQR7cyMZS9xYIX2bMGappvegSE3t4J7UcyvZ/P6AUxOyiF9eZVnHio3z0X0/IGfWnix0mINaGbebN+JsxHTD5SHcSMKiL6AhgAfcCfiR8NxsVFzySriILuMG0g50tWwVi2hxlG631BhP5UHKC/FhDfQgSP2IYs8hXN+UOozsvlJyKFs963t+6LYWe0osKO7lDLRzBDOvLlDwTo2OX5sRTSpwR8c/l/rkL2Is8PSC26FwDFu5Dn36oDs6ui0yo4yI0hBt1iiNPKclATdcvSc6VoDIREm3sonP7kCDNe9tBu0h/FVaIp4tQOrVowFpSEuoa3xo2tUhXckRxQbh4c0vScMsQg+Ia7Y6VzVHmVlDeBk3+FCSbYajy+kDmn2zklUjgPRr3AD2p15zo7+PDrl0trGYQNEGMkw3E4uvcdBq1OFeAFIFRb/gQddsmFBJh4gZtoBnxkkWbKilJmEyrfE0kRAQQrfcWQnVzW74f1ttgLVUFFJi1nJJSUPKtp8sSuXiqBhn/4kM3Z+RumZF+V+cWVUgf322KgsBO5f8ar5ZQLjWNE1cJLaNwot6/4Vq85HmCcBitS/jLDEvWL5px610Yo4weTC97/+fu5V0AJ7M67+UXEx/7Wf+hal91wPBfCWvf3RfneTB/SkW3kvDst8tFV9UdMEx+j06LslsW9FKfi1alEjzfXTMiLp/yG7Enhy6sThcLVzXAqvlG72Kd8Sg6VyIdZ1YhuApcjNVdApdZhHY1EgGsKWYNc+9K9cL8byXUwhYBF7S/0E+rnbLz+8suiXMcYI7Qd+IRi6JPeH0/vTXIEfmxxBVb1i2LY9ZcjyxDOhZ33jXb8Uv5bfwLNLP308gbCqsBKXfPu8R9hwDQeoRdEc0VQvNUw+o1XakAhJnltBVAmxIwKnRlg7TTAOX/HiIbCKELXppoH9JQhCDvX1P1EEqJjvXAQqcGZaXo6jeoMhWdK/IycRNRSL7OouVFUn8aQlvXvyasMu02wYWva5OPEBIyzW8wV3+ohDFnm0Z4sxSXfsCL/Cyf0uX9QEJYVuAZHk9Y1x18o6Ovnmgp9zUsEOZiLk0lW4yMW8bC83RDNxSf+3mg7nGvb/J3szBSFEUZh3qYSGpOmwvvVeC8ZlmWH5RMX20CEUFntTUMNuD3vRO6H7cIQ1URwSJDj0PYda0YqOfkypOqVXHrE3NUTtPYHskQz16citUmc+zH9BCERxP71IJ2Q+2K8I/n+TEVZRkYOoojVJSorexzYXh+Wr4fHmQ2lrRciexz6DwLj/jW0xL9w7vhs6vnkCIMzrK2zIgX/bQq2qCWITUrj4Ff5P9qHR6xWogk/jQBe/mwu+7fYhJlXXGqrT2Cwdk3nWrql/sWv7QNFRnN4jHQhUQF5X26HPeQKK0vwC0fotNKI6+O9BE1tXKYOzD5poqIHexasnEWgmKPfkp8t1Ct6iSn8R4OHJGdGY+urXJSrBJ3CFfTiBH2BOAyGlkPZ1fXlMosCOsVMwaYv2Av00AtDTr9N8plG+ADv3ec/YNIPEhE/mKeHxHYxK6VA/2kWkta1b37hWcVDnYae0Q8TjHesEqtemfBGsmJ8Myq5dDeiq5OrKzguBA7rCyAFR+Fli1aYtfXGOcUStc34NpmJvy48DrNDd8gGo7mIsxIAJidc9ZEoGiLVBAyoCv/WgD+hBAMVshCrmkUPoj2vnGAPaYUj2t60xmEGU4qtC6O4MzP2XIGWUgg5R9wmjDdWAzgIcgHpDctNO8TDcF8FG8PWCq6CY2FOAHrGFDxm9lKE+60KEzwpobBJR/SdgHIDswUcZkvVij0mHcThtQBehL/Wc1/u2syMX9VGKhJghjXfrDAX6oGf/3eah4JblFvRk9u2HsqZG9eywITi8klCxjjic4aNT0LNn7DF+477w3eWyyCYYIMjsNdtB11hbXIarlb8XzRxziaupAS0ubiesWnsi3cxfnGQOk0F4agm9m2UR85Uze4+JAjAh1URAFIEpOzA5Qic5s6ViESaZUB2tZ3oAQ5609CyaupFsP6gZDMlchFB+bjkrsWVBy6PFHan12di8Rvzjs1jrQR6Ivl0Ae1hK61C8KKwrEhFuPuHsVJ4iUao45yLTMeqhtNVTMbbq1X8CWZZ/zfUkoRoSoAosmYbWxvf4H7l+ecFUk1gAr8/ohHS99h+n0hBBtOIZCGXZ+1DI8rfbzulOIQoSk06DQH489ydpxrKjobFQo0QSICyduDD5SDBrUIYhqGwGhVoV1psok8EiRxRX0Qp/DCqgm1Ax+syxZtzZacFNGscxVMosQml35cyIC8wxkDTGiNb+U6eB7POX3QVRoQA1/CcMueSRbmQoicROsgFMT28kx+Btybn1OqwxUmKRl2tC/gHQjTgofolDSbb3MfydAdrBLlw7FUEd0AwSWoE/xEJVdX3jTeqVUUQTEvkhg7lu4s7RXCVRRrWZH9urYe5R0VPLRNmbXs4td63jQyE15lGkshof/ip9uNGRbC/he2/uCdaYm/25FS8Z+bcv4dMZ+sAuK1Vymb0/ZDoQe/Ev7GV4Nao63zgYj0lInJbhe9uC0kMQyZcKKqMfrre0oddpOctp6nX4alCl98c41OElS4Q2SGkffnTs12W9Aw4hZpplT953X1oaKAVHIEvZocAehU/rBpOaVl0HTHIrbgEQIi6j0dxIcOfwRSeavjWBaCqmSNJLUp0p6FpleWHO8bkepe2DYRWkODE8j23XF1ETaldo6ElTAUZBh6dFXO0hWDThSy5bo8GX2emNHqgUcbErFMj8/0xS8OY//CMrEMEVbdH3MDcre70Tu/c3/dTxWs1OdPhE6lKsYKr1Df1KMa6+TwsRJRp9q7Zm2SC2WmECYebV5XJVHRasAId07+zxSwZQg2rW6lhYXwyhTi/QMajj/FQSxlh3NT1v5MrX5CEHGPNlJ3PmlQ6Is+oFgeUxROM8QmFY6DZOIuTsju5WcZIkB1cJpxpP2aumF6at/BHxVISYYAx4beoBR9jKIr2RMamtunwHgoFslZnC+Lds8YoYDS0fCWsls7cIP7LVQDH2SQI4NWnk8ZJSYyvZwUYhN+dOwj7IWjX66vfuzDmToYnZHnLGdnjPeZW57nNEBJHgK7EHygwLc6QPnCECHI7RLD6RSbyLBlSZC381QpOqkXMyTawfocv1t0pTCqs4iTHaBWKLkccle5aGdeSnA1fBJIbHLno06t50IB9lzMQhsXWgdP4UyimKGTgTN0fxPiHJhk0QxwZf9E/DCgqC9MDRK+vQUQzD4/Vjs9yJI7n5AWLi0HFH8OzyuKTHp3Csc0HD18hGwPaWFb1m+simb8oBFMm6VHU8M58suLVy2AOwkWHwKPGSg0yO6+bq04YIqLG1Nh+Q+ZID1VN2VsI3w2NxGoufdTv2iMKoV+RywbBIUVEqj1KO+CL3UmXln3IUXmGguJmXnVsT0J2NqXOjXrzxBO6//XSh6NC47RPYkC5gMVIub/jd1lcI7xRY0rg99EGtXvv3iqPwb5tks4+7ELaxDK6+8Wlc9Ht6ReBnqezoUUvearXHrDGeVN9ocYRkKnGTZw63ksGjCv8PlUz2uJCBBDTGaYtyD82mw4KsPGqwoUVN9/fuUouxGIxvRzuFYNGSrXH94e8ykWKvjxkGasUB965tNK8zfaTRo7lxrAa5ITGeCIHfDFUOHgCT0hsAZ3GB8Y6yRs96QZWUyMKVzIlB4T7xKXoxoHsLev95k5/RwT2KrXfEzhCJylm3UAiDzkF46VDZyfGQsDjwKTAKpL3R15fYjBA/DmYZnhW0BKT6oS15zqsQSsYLr/2XLQHONUFw1EZd4F1/iof5CPBOQbKh2ISv+qY4abyU+62YJuUToSKh2/lfxnArzjKJAjyuXIFnTkLrv7AQOrHDN0rDwfexzJTv1UPeHh6vGfyOuF88wRe6I8qoxZmmXux7pmZzBAFhrLy9Tv7wD/6XlpQIE80BvwXv/k9ZVgPzLsQcjQJWIRrDY/XnO7OM8+Z1L6RK+6FVcEzzVQ6gaDDgHnOBZbKRu2YqEhclLkEalgkOPt2PkQZLsJqg5nXVbTeHRcKU84FT0qjFTSwPQrCJeRs/Nihhpmmtd0g/h4G2X+OlDY/ZMQAIENUqQ2V2L+vUlkTv24FEDhkGZNBO36fS1c/aE85xnIjBpgsCLUvG6cWT7lhTAQ+i8heFwrCWyKA/CNXqc8FPh7KZYzdgsHbQRsMTbRSxn22rN7wFPOSIgFx72DxZ4f2mhQTVj0gC11Y5xWcjvDExCDpGBuMeF4UnxD7E4KtBgwMDAxMDIwMzA0MDUwNjA3MDgwOTEwMTExMjEzMTQxNTE2MTcxODE5MjAyMTIyMjMyNDI1MjYyNzI4MjkzMDMxMzIzMzM0MzUzNjM3MzgzOTQwNDE0MjQzNDQ0NTQ2NDc0ODQ5NTA1MTUyNTM1NDU1NTY1NzU4NTk2MDYxNjI2MzY0NjU2NjY3Njg2OTcwNzE3MjczNzQ3NTc2Nzc3ODc5ODA4MTgyODM4NDg1ODY4Nzg4ODk5MDkxOTI5Mzk0OTU5Njk3OTg5OTAuMGNhbGxlZCBgT3B0aW9uOjp1bndyYXAoKWAgb24gYSBgTm9uZWAgdmFsdWUAABMAAAAEAAAABAAAABQAAAAVAAAAFgAAACg1EAAAAAAAZW50aXR5IG5vdCBmb3VuZHBlcm1pc3Npb24gZGVuaWVkY29ubmVjdGlvbiByZWZ1c2VkY29ubmVjdGlvbiByZXNldGhvc3QgdW5yZWFjaGFibGVuZXR3b3JrIHVucmVhY2hhYmxlY29ubmVjdGlvbiBhYm9ydGVkbm90IGNvbm5lY3RlZGFkZHJlc3MgaW4gdXNlYWRkcmVzcyBub3QgYXZhaWxhYmxlbmV0d29yayBkb3duYnJva2VuIHBpcGVlbnRpdHkgYWxyZWFkeSBleGlzdHNvcGVyYXRpb24gd291bGQgYmxvY2tub3QgYSBkaXJlY3RvcnlpcyBhIGRpcmVjdG9yeWRpcmVjdG9yeSBub3QgZW1wdHlyZWFkLW9ubHkgZmlsZXN5c3RlbSBvciBzdG9yYWdlIG1lZGl1bWZpbGVzeXN0ZW0gbG9vcCBvciBpbmRpcmVjdGlvbiBsaW1pdCAoZS5nLiBzeW1saW5rIGxvb3Apc3RhbGUgbmV0d29yayBmaWxlIGhhbmRsZWludmFsaWQgaW5wdXQgcGFyYW1ldGVyaW52YWxpZCBkYXRhdGltZWQgb3V0d3JpdGUgemVyb25vIHN0b3JhZ2Ugc3BhY2VzZWVrIG9uIHVuc2Vla2FibGUgZmlsZWZpbGVzeXN0ZW0gcXVvdGEgZXhjZWVkZWRmaWxlIHRvbyBsYXJnZXJlc291cmNlIGJ1c3lleGVjdXRhYmxlIGZpbGUgYnVzeWRlYWRsb2NrY3Jvc3MtZGV2aWNlIGxpbmsgb3IgcmVuYW1ldG9vIG1hbnkgbGlua3NpbnZhbGlkIGZpbGVuYW1lYXJndW1lbnQgbGlzdCB0b28gbG9uZ29wZXJhdGlvbiBpbnRlcnJ1cHRlZHVuc3VwcG9ydGVkdW5leHBlY3RlZCBlbmQgb2YgZmlsZW91dCBvZiBtZW1vcnlvdGhlciBlcnJvcnVuY2F0ZWdvcml6ZWQgZXJyb3IgKG9zIGVycm9yICkAAAAoNRAAAAAAAB04EAALAAAAKDgQAAEAAABtZW1vcnkgYWxsb2NhdGlvbiBvZiAgYnl0ZXMgZmFpbGVkAABEOBAAFQAAAFk4EAANAAAAbGlicmFyeS9zdGQvc3JjL2FsbG9jLnJzeDgQABgAAABVAQAACQAAAGxpYnJhcnkvc3RkL3NyYy9wYW5pY2tpbmcucnOgOBAAHAAAAE8CAAAfAAAAoDgQABwAAABQAgAAHgAAABcAAAAMAAAABAAAABgAAAATAAAACAAAAAQAAAAZAAAAEwAAAAgAAAAEAAAAGgAAABsAAAAcAAAAEAAAAAQAAAAdAAAAHgAAAB8AAAAAAAAAAQAAACAAAABvcGVyYXRpb24gc3VjY2Vzc2Z1bBAAAAARAAAAEgAAABAAAAAQAAAAEwAAABIAAAANAAAADgAAABUAAAAMAAAACwAAABUAAAAVAAAADwAAAA4AAAATAAAAJgAAADgAAAAZAAAAFwAAAAwAAAAJAAAACgAAABAAAAAXAAAAGQAAAA4AAAANAAAAFAAAAAgAAAAbAAAADgAAABAAAAAWAAAAFQAAAAsAAAAWAAAADQAAAAsAAAATAAAAMDUQAEA1EABRNRAAYzUQAHM1EACDNRAAljUQAKg1EAC1NRAAwzUQANg1EADkNRAA7zUQAAQ2EAAZNhAAKDYQADY2EABJNhAAbzYQAKc2EADANhAA1zYQAOM2EADsNhAA9jYQAAY3EAAdNxAANjcQAEQ3EABRNxAAZTcQAG03EACINxAAljcQAKY3EAC8NxAA0TcQANw3EADyNxAA/zcQAAo4EABsaWJyYXJ5L2FsbG9jL3NyYy9yYXdfdmVjLnJzY2FwYWNpdHkgb3ZlcmZsb3cAAACsOhAAEQAAAJA6EAAcAAAADAIAAAUAAABjYWxsZWQgYE9wdGlvbjo6dW53cmFwKClgIG9uIGEgYE5vbmVgIHZhbHVlbGlicmFyeS9jb3JlL3NyYy9mbXQvbW9kLnJzLi4eOxAAAgAAACYAAAAAAAAAAQAAACcAAABpbmRleCBvdXQgb2YgYm91bmRzOiB0aGUgbGVuIGlzICBidXQgdGhlIGluZGV4IGlzIAAAODsQACAAAABYOxAAEgAAAGA6IADYOhAAAAAAAH07EAACAAAAbGlicmFyeS9jb3JlL3NyYy9mbXQvbnVtLnJzAJA7EAAbAAAAaQAAABQAAAAweDAwMDEwMjAzMDQwNTA2MDcwODA5MTAxMTEyMTMxNDE1MTYxNzE4MTkyMDIxMjIyMzI0MjUyNjI3MjgyOTMwMzEzMjMzMzQzNTM2MzczODM5NDA0MTQyNDM0NDQ1NDY0NzQ4NDk1MDUxNTI1MzU0NTU1NjU3NTg1OTYwNjE2MjYzNjQ2NTY2Njc2ODY5NzA3MTcyNzM3NDc1NzY3Nzc4Nzk4MDgxODI4Mzg0ODU4Njg3ODg4OTkwOTE5MjkzOTQ5NTk2OTc5ODk5AAADOxAAGwAAABsJAAAWAAAAAzsQABsAAAAUCQAAHgAAAHJhbmdlIHN0YXJ0IGluZGV4ICBvdXQgb2YgcmFuZ2UgZm9yIHNsaWNlIG9mIGxlbmd0aCCoPBAAEgAAALo8EAAiAAAAcmFuZ2UgZW5kIGluZGV4IOw8EAAQAAAAujwQACIAAABzbGljZSBpbmRleCBzdGFydHMgYXQgIGJ1dCBlbmRzIGF0IAAMPRAAFgAAACI9EAANAAAAWy4uLl1ieXRlIGluZGV4ICBpcyBub3QgYSBjaGFyIGJvdW5kYXJ5OyBpdCBpcyBpbnNpZGUgIChieXRlcyApIG9mIGBFPRAACwAAAFA9EAAmAAAAdj0QAAgAAAB+PRAABgAAAHw7EAABAAAAYmVnaW4gPD0gZW5kICggPD0gKSB3aGVuIHNsaWNpbmcgYAAArD0QAA4AAAC6PRAABAAAAL49EAAQAAAAfDsQAAEAAAAgaXMgb3V0IG9mIGJvdW5kcyBvZiBgAABFPRAACwAAAPA9EAAWAAAAfDsQAAEAAABsaWJyYXJ5L2NvcmUvc3JjL3N0ci9tb2QucnMAID4QABsAAAADAQAAHQAAAGxpYnJhcnkvY29yZS9zcmMvdW5pY29kZS9wcmludGFibGUucnMAAABMPhAAJQAAABoAAAA2AAAATD4QACUAAAAKAAAAHAAAAAAGAQEDAQQCBQcHAggICQIKBQsCDgQQARECEgUTERQBFQIXAhkNHAUdCB8BJAFqBGsCrwOxArwCzwLRAtQM1QnWAtcC2gHgBeEC5wToAu4g8AT4AvoD+wEMJzs+Tk+Pnp6fe4uTlqKyuoaxBgcJNj0+VvPQ0QQUGDY3Vld/qq6vvTXgEoeJjp4EDQ4REikxNDpFRklKTk9kZVy2txscBwgKCxQXNjk6qKnY2Qk3kJGoBwo7PmZpj5IRb1+/7u9aYvT8/1NUmpsuLycoVZ2goaOkp6iturzEBgsMFR06P0VRpqfMzaAHGRoiJT4/5+zv/8XGBCAjJSYoMzg6SEpMUFNVVlhaXF5gY2Vma3N4fX+KpKqvsMDQrq9ub76TXiJ7BQMELQNmAwEvLoCCHQMxDxwEJAkeBSsFRAQOKoCqBiQEJAQoCDQLTkOBNwkWCggYO0U5A2MICTAWBSEDGwUBQDgESwUvBAoHCQdAICcEDAk2AzoFGgcEDAdQSTczDTMHLggKgSZSSysIKhYaJhwUFwlOBCQJRA0ZBwoGSAgnCXULQj4qBjsFCgZRBgEFEAMFgItiHkgICoCmXiJFCwoGDRM6Bgo2LAQXgLk8ZFMMSAkKRkUbSAhTDUkHCoD2RgodA0dJNwMOCAoGOQcKgTYZBzsDHFYBDzINg5tmdQuAxIpMYw2EMBAWj6qCR6G5gjkHKgRcBiYKRgooBROCsFtlSwQ5BxFABQsCDpf4CITWKgmi54EzDwEdBg4ECIGMiQRrBQ0DCQcQkmBHCXQ8gPYKcwhwFUZ6FAwUDFcJGYCHgUcDhUIPFYRQHwYGgNUrBT4hAXAtAxoEAoFAHxE6BQGB0CqC5oD3KUwECgQCgxFETD2AwjwGAQRVBRs0AoEOLARkDFYKgK44HQ0sBAkHAg4GgJqD2AQRAw0DdwRfBgwEAQ8MBDgICgYoCCJOgVQMHQMJBzYIDgQJBwkHgMslCoQGAAEDBQUGBgIHBggHCREKHAsZDBoNEA4MDwQQAxISEwkWARcEGAEZAxoHGwEcAh8WIAMrAy0LLgEwAzECMgGnAqkCqgSrCPoC+wX9Av4D/wmteHmLjaIwV1iLjJAc3Q4PS0z7/C4vP1xdX+KEjY6RkqmxurvFxsnK3uTl/wAEERIpMTQ3Ojs9SUpdhI6SqbG0urvGys7P5OUABA0OERIpMTQ6O0VGSUpeZGWEkZudyc7PDREpOjtFSVdbXF5fZGWNkam0urvFyd/k5fANEUVJZGWAhLK8vr/V1/Dxg4WLpKa+v8XHz9rbSJi9zcbOz0lOT1dZXl+Jjo+xtre/wcbH1xEWF1tc9vf+/4Btcd7fDh9ubxwdX31+rq9/u7wWFx4fRkdOT1haXF5+f7XF1NXc8PH1cnOPdHWWJi4vp6+3v8fP19+aQJeYMI8f0tTO/05PWlsHCA8QJy/u725vNz0/QkWQkVNndcjJ0NHY2ef+/wAgXyKC3wSCRAgbBAYRgawOgKsFHwmBGwMZCAEELwQ0BAcDAQcGBxEKUA8SB1UHAwQcCgkDCAMHAwIDAwMMBAUDCwYBDhUFTgcbB1cHAgYXDFAEQwMtAwEEEQYPDDoEHSVfIG0EaiWAyAWCsAMaBoL9A1kHFgkYCRQMFAxqBgoGGgZZBysFRgosBAwEAQMxCywEGgYLA4CsBgoGLzFNA4CkCDwDDwM8BzgIKwWC/xEYCC8RLQMhDyEPgIwEgpcZCxWIlAUvBTsHAg4YCYC+InQMgNYaDAWA/wWA3wzynQM3CYFcFIC4CIDLBQoYOwMKBjgIRggMBnQLHgNaBFkJgIMYHAoWCUwEgIoGq6QMFwQxoQSB2iYHDAUFgKYQgfUHASAqBkwEgI0EgL4DGwMPDWxpYnJhcnkvY29yZS9zcmMvdW5pY29kZS91bmljb2RlX2RhdGEucnMQRBAAKAAAAFAAAAAoAAAAEEQQACgAAABcAAAAFgAAADAxMjM0NTY3ODlhYmNkZWZsaWJyYXJ5L2NvcmUvc3JjL2VzY2FwZS5ycwAAaEQQABoAAAA0AAAABQAAAFx1ewBoRBAAGgAAAGIAAAAjAAAARXJyb3IAAAAAAwAAgwQgAJEFYABdE6AAEhcgHwwgYB/vLKArKjAgLG+m4CwCqGAtHvtgLgD+IDae/2A2/QHhNgEKITckDeE3qw5hOS8YoTkwHGFI8x6hTEA0YVDwaqFRT28hUp28oVIAz2FTZdGhUwDaIVQA4OFVruJhV+zkIVnQ6KFZIADuWfABf1oAcAAHAC0BAQECAQIBAUgLMBUQAWUHAgYCAgEEIwEeG1sLOgkJARgEAQkBAwEFKwM8CCoYASA3AQEBBAgEAQMHCgIdAToBAQECBAgBCQEKAhoBAgI5AQQCBAICAwMBHgIDAQsCOQEEBQECBAEUAhYGAQE6AQECAQQIAQcDCgIeATsBAQEMAQkBKAEDATcBAQMFAwEEBwILAh0BOgECAQIBAwEFAgcCCwIcAjkCAQECBAgBCQEKAh0BSAEEAQIDAQEIAVEBAgcMCGIBAgkLB0kCGwEBAQEBNw4BBQECBQsBJAkBZgQBBgECAgIZAgQDEAQNAQICBgEPAQADAAMdAh4CHgJAAgEHCAECCwkBLQMBAXUCIgF2AwQCCQEGA9sCAgE6AQEHAQEBAQIIBgoCATAfMQQwBwEBBQEoCQwCIAQCAgEDOAEBAgMBAQM6CAICmAMBDQEHBAEGAQMCxkAAAcMhAAONAWAgAAZpAgAEAQogAlACAAEDAQQBGQIFAZcCGhINASYIGQsuAzABAgQCAicBQwYCAgICDAEIAS8BMwEBAwICBQIBASoCCAHuAQIBBAEAAQAQEBAAAgAB4gGVBQADAQIFBCgDBAGlAgAEAAJQA0YLMQR7ATYPKQECAgoDMQQCAgcBPQMkBQEIPgEMAjQJCgQCAV8DAgEBAgYBAgGdAQMIFQI5AgEBAQEWAQ4HAwXDCAIDAQEXAVEBAgYBAQIBAQIBAusBAgQGAgECGwJVCAIBAQJqAQEBAgYBAWUDAgQBBQAJAQL1AQoCAQEEAZAEAgIEASAKKAYCBAgBCQYCAy4NAQIABwEGAQFSFgIHAQIBAnoGAwEBAgEHAQFIAgMBAQEAAgsCNAUFAQEBAAEGDwAFOwcAAT8EUQEAAgAuAhcAAQEDBAUICAIHHgSUAwA3BDIIAQ4BFgUBDwAHARECBwECAQVkAaAHAAE9BAAEAAdtBwBggPAAbwlwcm9kdWNlcnMCCGxhbmd1YWdlAQRSdXN0AAxwcm9jZXNzZWQtYnkDBXJ1c3RjHTEuNzIuMCAoNTY4MGZhMThmIDIwMjMtMDgtMjMpBndhbHJ1cwYwLjIwLjMMd2FzbS1iaW5kZ2VuBjAuMi44OQAsD3RhcmdldF9mZWF0dXJlcwIrD211dGFibGUtZ2xvYmFscysIc2lnbi1leHQ=",
let imports: any = {};
imports["__wbindgen_placeholder__"] = imports;
aocDay2
@nbbaier
// @title Day 2 solutions
Script
// @aoc2023
// @title Day 2 solutions
import getAocData from "https://esm.town/v/nbbaier/getAocData";
const data = await getAocData(2);
const input = (await data.text()).split("\n").slice(0, -1);
aocDay4
@nbbaier
// @title Day 4 solutions
Script
// @aoc2023
// @title Day 4 solutions
import _ from "npm:lodash-es";
import getAocData from "https://esm.town/v/nbbaier/getAocData";
const data = await (await getAocData(4)).text();
const input = data.split("\n").slice(0, -1);
aocDatabase
@nbbaier
An interactive, runnable TypeScript val by nbbaier
Script
const { key } = extractValInfo(import.meta.url);
const aocDb = await BlobPreset<Data>(key, { days: [] });
type AocDB = typeof aocDb;
async function updateAocDB(db: AocDB, day: number, input: string, riddleUrl: string) {
db.data.days.push({ id: day, input, riddleUrl });
return await db.write();
export { aocDb, updateAocDB };
aoc15_05
@karfau
// expect(secondStar(""), "*1 sample 2").to.equal("?");
Script
const sum = (sum, current) => sum + current;
const firstStar = (input: string) => {
return input.split("\n").map(
(line, li) => {
if (/ab|cd|pq|xy/.test(line)) return 0;
const vowels = line.match(/[aeiou]/g)?.length;
if ((vowels ?? 0) < 3) return 0;
const double = line.split("").find((c, i) => c === line[i + 1]);
// double && debug(double, `${li} ${line} (${vowels})`);
return double ? 1 : 0;
aoc_2023_10_1
@robsimmons
An interactive, runnable TypeScript val by robsimmons
Script
.map((line) => line.trim().split(""));
const dusa = new Dusa(`
# AOC Day 10, Part 1
#builtin INT_PLUS plus
#builtin INT_MINUS minus
aoc_2023_10_2
@robsimmons
An interactive, runnable TypeScript val by robsimmons
Script
.map((line) => line.trim().split(""));
const dusa = new Dusa(`
# AOC Day 10, Part 2
#builtin INT_PLUS plus
#builtin INT_MINUS minus